
A web server for monitoring and controlling a MakerBot Replicator over the USB host and ethernet.
Dependencies: IAP NTPClient RTC mbed-rtos mbed Socket lwip-sys lwip BurstSPI
Fork of LPC1768_Mini-DK by
SimpleSocket.h
00001 #ifndef SIMPLE_SOCKET_H 00002 #define SIMPLE_SOCKET_H 00003 00004 #include "mbed.h" 00005 #include "EthernetInterface.h" 00006 #include <stdarg.h> 00007 00008 extern Serial pc; 00009 00010 /** 00011 * client socket class for communication endpoint 00012 */ 00013 class ClientSocket { 00014 friend class ServerSocket; 00015 00016 public: 00017 ClientSocket(char *hostname, int port) : preread(-1), timeout(1.5) { 00018 if (EthernetInterface::getIPAddress() == NULL) { 00019 EthernetInterface::init(); 00020 EthernetInterface::connect(); 00021 } 00022 sock = new TCPSocketConnection(); 00023 sock->connect(hostname, port); 00024 sock->set_blocking(false, (int) (timeout * 1000)); 00025 } 00026 00027 ClientSocket(TCPSocketConnection *sock) : sock(sock), preread(-1), timeout(1.5) { 00028 sock->set_blocking(false, (int) (timeout * 1000)); 00029 } 00030 00031 ~ClientSocket() { 00032 // do NOT close in the destructor. 00033 } 00034 00035 void setTimeout(float timeout) { 00036 sock->set_blocking(false, (int) (timeout * 1000)); 00037 this->timeout = timeout; 00038 } 00039 00040 bool available() { 00041 if (preread == -1) { 00042 char c; 00043 sock->set_blocking(false, 0); 00044 if (sock->receive((char *) &c, 1) <= 0) { 00045 sock->set_blocking(false, (int) (timeout * 1000)); 00046 return false; 00047 } 00048 preread = c & 255; 00049 sock->set_blocking(false, (int) (timeout * 1000)); 00050 } 00051 00052 return true; 00053 } 00054 00055 int read() { 00056 char c; 00057 if (preread != -1) { 00058 c = (char) preread; 00059 preread = -1; 00060 } else { 00061 if (sock->receive((char *) &c, 1) <= 0) 00062 return -1; 00063 } 00064 00065 return c & 255; 00066 } 00067 00068 int read(char *buf, int size) { 00069 if (size <= 0) 00070 return 0; 00071 00072 int nread = 0; 00073 if (preread != -1) { 00074 nread = 1; 00075 *buf++ = (char) preread; 00076 preread = -1; 00077 size--; 00078 } 00079 00080 if (size > 0) { 00081 size = sock->receive_all(buf, size - 1); 00082 if (size > 0) 00083 nread += size; 00084 } 00085 00086 return (nread > 0) ? nread : -1; 00087 } 00088 00089 int scanf(const char *format, ...) { 00090 va_list argp; 00091 va_start(argp, format); 00092 char buf[256]; 00093 00094 int len = read(buf, sizeof(buf) - 1); 00095 if (len <= 0) 00096 return 0; 00097 buf[len] = '\0'; 00098 00099 int ret = vsscanf(buf, format, argp); 00100 va_end(argp); 00101 00102 return ret; 00103 } 00104 00105 int write(char c) { 00106 return sock->send_all(&c, 1); 00107 } 00108 00109 int write(char *buf, int size) { 00110 return sock->send_all(buf, size); 00111 } 00112 00113 int printf(const char *format, ...) { 00114 va_list argp; 00115 va_start(argp, format); 00116 char buf[256]; 00117 00118 int len = vsnprintf(buf, sizeof(buf), format, argp); 00119 va_end(argp); 00120 00121 return write(buf, len); 00122 } 00123 00124 void close() { 00125 if (sock) { 00126 sock->close(); 00127 delete sock; 00128 sock = 0; 00129 } 00130 } 00131 00132 bool connected() { 00133 return sock && sock->is_connected(); 00134 } 00135 00136 operator bool() { 00137 return connected(); 00138 } 00139 00140 char* get_address() { 00141 return sock->get_address(); 00142 } 00143 00144 private: 00145 TCPSocketConnection *sock; 00146 int preread; 00147 float timeout; 00148 }; 00149 00150 /** 00151 * server socket class for handling incoming communication requests 00152 */ 00153 class ServerSocket { 00154 public: 00155 ServerSocket(int port) { 00156 // Assume that the interface is initialized. 00157 // For some reason this initialization code doesn't like being threaded. 00158 //if (EthernetInterface::getIPAddress() == NULL) { 00159 // EthernetInterface::init(); 00160 // EthernetInterface::connect(); 00161 //} 00162 ssock.bind(port); 00163 ssock.listen(); 00164 } 00165 00166 ClientSocket accept() { 00167 TCPSocketConnection *sock = new TCPSocketConnection(); 00168 ssock.accept(*sock); 00169 00170 return ClientSocket(sock); 00171 } 00172 00173 private: 00174 TCPSocketServer ssock; 00175 }; 00176 00177 /** 00178 * class for handling datagram 00179 */ 00180 class DatagramSocket { 00181 public: 00182 DatagramSocket(int port = 0, int bufsize = 512) : bufsize(bufsize) { 00183 if (EthernetInterface::getIPAddress() == NULL) { 00184 EthernetInterface::init(); 00185 EthernetInterface::connect(); 00186 } 00187 buf = new char[bufsize + 1]; 00188 usock.init(); 00189 usock.bind(port); 00190 usock.set_blocking(false, 1500); 00191 } 00192 00193 DatagramSocket(Endpoint& local, int bufsize = 512) : bufsize(bufsize) { 00194 if (EthernetInterface::getIPAddress() == NULL) { 00195 EthernetInterface::init(); 00196 EthernetInterface::connect(); 00197 } 00198 buf = new char[bufsize + 1]; 00199 usock.init(); 00200 usock.bind(local.get_port()); 00201 usock.set_blocking(false, 1500); 00202 } 00203 00204 ~DatagramSocket() { 00205 delete[] buf; 00206 } 00207 00208 void setTimeout(float timeout = 1.5) { 00209 usock.set_blocking(false, (int) (timeout * 1000)); 00210 } 00211 00212 int write(char *buf, int length) { 00213 if (length > bufsize) length = bufsize; 00214 this->length = length; 00215 memcpy(this->buf, buf, length); 00216 00217 return length; 00218 } 00219 00220 int printf(const char* format, ...) { 00221 va_list argp; 00222 va_start(argp, format); 00223 int len = vsnprintf(buf, bufsize, format, argp); 00224 va_end(argp); 00225 if (len > 0) 00226 length = len; 00227 00228 return len; 00229 } 00230 00231 void send(const char *host, int port) { 00232 Endpoint remote; 00233 remote.reset_address(); 00234 remote.set_address(host, port); 00235 usock.sendTo(remote, buf, length); 00236 } 00237 00238 void send(Endpoint& remote) { 00239 usock.sendTo(remote, buf, length); 00240 } 00241 00242 int receive(char *host = 0, int *port = 0) { 00243 Endpoint remote; 00244 length = usock.receiveFrom(remote, buf, bufsize); 00245 if (length > 0) { 00246 if (host) strcpy(host, remote.get_address()); 00247 if (port) *port = remote.get_port(); 00248 } 00249 00250 return length; 00251 } 00252 00253 int receive(Endpoint& remote) { 00254 return usock.receiveFrom(remote, buf, bufsize); 00255 } 00256 00257 int read(char *buf, int size) { 00258 int len = length < size ? length : size; 00259 if (len > 0) 00260 memcpy(buf, this->buf, len); 00261 00262 return len; 00263 } 00264 00265 int scanf(const char* format, ...) { 00266 va_list argp; 00267 va_start(argp, format); 00268 buf[length] = '\0'; 00269 int ret = vsscanf(buf, format, argp); 00270 va_end(argp); 00271 00272 return ret; 00273 } 00274 00275 private: 00276 char *buf; 00277 int bufsize; 00278 int length; 00279 UDPSocket usock; 00280 }; 00281 00282 #endif
Generated on Tue Jul 12 2022 17:52:03 by
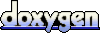