1/27/16 updated JJ
Embed:
(wiki syntax)
Show/hide line numbers
vl6180x.h
00001 #pragma once 00002 00003 #include "mbed.h" 00004 00005 class VL6180x 00006 { 00007 00008 public: 00009 struct Identification { 00010 char model; 00011 char modelRevMajor; 00012 char modelRevMinor; 00013 char moduleRevMajor; 00014 char moduleRevMinor; 00015 int date; 00016 int time; 00017 }; 00018 00019 VL6180x(PinName sda, PinName scl, char _address = 0xE0); 00020 VL6180x(I2C &i2c, char address = 0xE0 ); 00021 void initialize(); 00022 00023 int getDistance(); 00024 int getSingleDistance(); 00025 float getAmbientLight(); 00026 Identification getIdentification(); 00027 static void printIdentification(Identification id); 00028 void startContinuousOperation(); 00029 00030 void setRegister(int register, int value); 00031 int getRegister(int reg); 00032 00033 void setAddress(int address); 00034 00035 protected: 00036 int address; 00037 I2C i2c; 00038 00039 static const int I2C_SLAVE_DEFAULT_ADDRESS = 0x52; 00040 00041 static const int IDENTIFICATION_MODEL_ID = 0x000; 00042 static const int IDENTIFICATION_MODEL_REV_MAJOR = 0x001; 00043 static const int IDENTIFICATION_MODEL_REV_MINOR = 0x002; 00044 static const int IDENTIFICATION_MODULE_REV_MAJOR = 0x003; 00045 static const int IDENTIFICATION_MODULE_REV_MINOR = 0x004; 00046 static const int IDENTIFICATION_DATE_HI = 0x006; 00047 static const int IDENTIFICATION_DATE_LO = 0x007; 00048 static const int IDENTIFICATION_TIME = 0x008; 00049 static const int SYSTEM_MODE_GPIO0 = 0x010; 00050 static const int SYSTEM_MODE_GPIO1 = 0x011; 00051 static const int SYSTEM_HISTORY_CTRL = 0x012; 00052 static const int SYSTEM_INTERRUPT_CONFIG_GPIO = 0x014; 00053 static const int SYSTEM_INTERRUPT_CLEAR = 0x015; 00054 static const int SYSTEM_FRESH_OUT_OF_RESET = 0x016; 00055 static const int SYSTEM_GROUPED_PARAMETER_HOLD = 0x017; 00056 static const int SYSRANGE_START = 0x018; 00057 static const int SYSRANGE_THRESH_HIGH = 0x019; 00058 static const int SYSRANGE_THRESH_LOW = 0x01A; 00059 static const int SYSRANGE_INTERMEASUREMENT_PERIOD = 0x01B; 00060 static const int SYSRANGE_MAX_CONVERGENCE_TIME = 0x01C; 00061 static const int SYSRANGE_CROSSTALK_COMPENSATION_RATE = 0x01E; 00062 static const int SYSRANGE_CROSSTALK_VALID_HEIGHT = 0x021; 00063 static const int SYSRANGE_EARLY_CONVERGENCE_ESTIMATE = 0x022; 00064 static const int SYSRANGE_PART_TO_PART_RANGE_OFFSET = 0x024; 00065 static const int SYSRANGE_RANGE_IGNORE_VALID_HEIGHT = 0x025; 00066 static const int SYSRANGE_RANGE_IGNORE_THRESHOLD = 0x026; 00067 static const int SYSRANGE_MAX_AMBIENT_LEVEL_MULT = 0x02C; 00068 static const int SYSRANGE_RANGE_CHECK_ENABLES = 0x02D; 00069 static const int SYSRANGE_VHV_RECALIBRATE = 0x02E; 00070 static const int SYSRANGE_VHV_REPEAT_RATE = 0x031; 00071 static const int SYSALS_START = 0x038; 00072 static const int SYSALS_THRESH_HIGH = 0x03A; 00073 static const int SYSALS_THRESH_LOW = 0x03C; 00074 static const int SYSALS_INTERMEASUREMENT_PERIOD = 0x03E; 00075 static const int SYSALS_ANALOGUE_GAIN = 0x03F; 00076 static const int SYSALS_INTEGRATION_PERIOD = 0x040; 00077 static const int RESULT_RANGE_STATUS = 0x04D; 00078 static const int RESULT_ALS_STATUS = 0x04E; 00079 static const int RESULT_INTERRUPT_STATUS_GPIO = 0x04F; 00080 static const int RESULT_ALS_VAL = 0x050; 00081 static const int RESULT_HISTORY_BUFFER_0 = 0x052; 00082 static const int RESULT_HISTORY_BUFFER_1 = 0x054; 00083 static const int RESULT_HISTORY_BUFFER_2 = 0x056; 00084 static const int RESULT_HISTORY_BUFFER_3 = 0x058; 00085 static const int RESULT_HISTORY_BUFFER_4 = 0x05A; 00086 static const int RESULT_HISTORY_BUFFER_5 = 0x05C; 00087 static const int RESULT_HISTORY_BUFFER_6 = 0x05E; 00088 static const int RESULT_HISTORY_BUFFER_7 = 0x060; 00089 static const int RESULT_RANGE_VAL = 0x062; 00090 static const int RESULT_RANGE_RAW = 0x064; 00091 static const int RESULT_RANGE_RETURN_RATE = 0x066; 00092 static const int RESULT_RANGE_REFERENCE_RATE = 0x068; 00093 static const int RESULT_RANGE_RETURN_SIGNAL_COUNT = 0x06C; 00094 static const int RESULT_RANGE_REFERENCE_SIGNAL_COUNT = 0x070; 00095 static const int RESULT_RANGE_RETURN_AMB_COUNT = 0x074; 00096 static const int RESULT_RANGE_REFERENCE_AMB_COUNT = 0x078; 00097 static const int RESULT_RANGE_RETURN_CONV_TIME = 0x07C; 00098 static const int RESULT_RANGE_REFERENCE_CONV_TIME = 0x080; 00099 static const int READOUT_AVERAGING_SAMPLE_PERIOD = 0x10A; 00100 static const int FIRMWARE_BOOTUP = 0x119; 00101 static const int FIRMWARE_RESULT_SCALER = 0x120; 00102 static const int I2C_SLAVE_DEVICE_ADDRESS = 0x212; 00103 static const int INTERLEAVED_MODE_ENABLE = 0x2A3; 00104 };
Generated on Sun Jul 17 2022 12:11:35 by
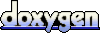