Interface for Sharp LS012B7DD01 TFT-LCD
Dependents: MAX32630FTHR_iButton_uSD_Logger
Sharp_LS012B7DD01.h
00001 /******************************************************************************* 00002 * The MIT License (MIT) 00003 * 00004 * Copyright (c) 2016 j3 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in 00014 * all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00022 * SOFTWARE. 00023 ******************************************************************************/ 00024 00025 #ifndef SHARP_LS012B7DD01_H 00026 #define SHARP_LS012B7DD01_H 00027 00028 #include "mbed.h" 00029 00030 /** 00031 * @brief Sharp LS012B&DD01 TFT-LCD 00032 */ 00033 class SharpLS012B7DD01 00034 { 00035 public: 00036 00037 static const uint8_t DATA_UPDATE_MODE = 0x80; 00038 static const uint8_t DISPLAY_MODE = 0; 00039 static const uint8_t ALL_CLEAR_MODE = 0x20; 00040 00041 enum SharpCmdResult 00042 { 00043 Success, 00044 Failure, 00045 }; 00046 00047 /** 00048 * @brief SharpLS012B7DD01 Constructor 00049 */ 00050 SharpLS012B7DD01(PinName disp, PinName extcomin, PinName cs, SPI &spiBus); 00051 00052 /** 00053 * @brief SharpLS012B7DD01 Destructor 00054 */ 00055 ~SharpLS012B7DD01(); 00056 00057 /** 00058 * @brief Prints character to given line and position 00059 */ 00060 SharpCmdResult print_char(uint8_t ln, uint8_t pos, char c); 00061 00062 /** 00063 * @breif Prints string to given line and position 00064 */ 00065 SharpCmdResult print_str(uint8_t ln, uint8_t pos, const char *s); 00066 00067 void clear_display(); 00068 00069 private: 00070 00071 /** 00072 * @brief Writes memory of Sharp LS012B7DD01 00073 */ 00074 SharpCmdResult write_memory(uint8_t data[][23], uint8_t lines, uint8_t offset); 00075 00076 /** 00077 * @brief Updates display 00078 */ 00079 void update(const uint8_t mode); 00080 00081 /** 00082 * @brief Reverses bit order 00083 */ 00084 uint8_t rev_bit(uint8_t data); 00085 00086 /** 00087 * @brief Call back for ticker 00088 */ 00089 void toggle_extcomin(); 00090 00091 uint8_t m_data[38][23]; 00092 00093 DigitalOut m_disp; 00094 DigitalOut m_extcomin; 00095 DigitalOut m_cs; 00096 SPI m_spi; 00097 Ticker m_extcominTicker; 00098 }; 00099 00100 #endif /*SHARP_LS012B7DD01_H*/
Generated on Tue Jul 12 2022 18:25:26 by
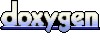