
ADC example on AIN_0
Dependencies: max32630fthr mbed
main.cpp
00001 /****************************************************************************** 00002 * MIT License 00003 * 00004 * Copyright (c) 2017 Justin J. Jordan 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 00013 * The above copyright notice and this permission notice shall be included in all 00014 * copies or substantial portions of the Software. 00015 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00022 * SOFTWARE. 00023 ******************************************************************************/ 00024 00025 00026 #include "mbed.h" 00027 #include "max32630fthr.h" 00028 00029 00030 int main () 00031 { 00032 //Init board and set GPIO to 3.3V logic 00033 MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 00034 00035 //Turn RGB LED off 00036 DigitalOut rLED(LED1, LED_OFF); 00037 DigitalOut gLED(LED2, LED_OFF); 00038 DigitalOut bLED(LED3, LED_OFF); 00039 00040 AnalogIn a0(AIN_0); 00041 float a0_val; 00042 00043 while(1) 00044 { 00045 //ADC is configured for no input scaling, no input buffer bypass, 00046 //and internal ref of 1.2V 00047 //Default range is 0 to 1.2V. 00048 //See target 'analogin_api.c' for mbed-2.0 and mbed-os. 00049 //Target User's guide page 567 covers ADC 00050 //https://www.maximintegrated.com/en/app-notes/index.mvp/id/6349 00051 a0_val = (a0.read() * 1.2F); 00052 00053 printf("AIN_0 = %f volts\r\n", a0_val); 00054 00055 wait(0.25); 00056 gLED = !gLED; 00057 } 00058 }
Generated on Wed Jul 20 2022 01:09:23 by
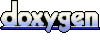