Library for uVGAIII
Embed:
(wiki syntax)
Show/hide line numbers
uVGAIII_main.cpp
00001 // 00002 // uVGAIII is a class to drive 4D Systems TFT touch screens 00003 // 00004 // Copyright (C) <2010> Stephane ROCHON <stephane.rochon at free.fr> 00005 // 00006 // uVGAIII is free software: you can redistribute it and/or modify 00007 // it under the terms of the GNU General Public License as published by 00008 // the Free Software Foundation, either version 3 of the License, or 00009 // (at your option) any later version. 00010 // 00011 // uVGAIII is distributed in the hope that it will be useful, 00012 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 // GNU General Public License for more details. 00015 // 00016 // You should have received a copy of the GNU General Public License 00017 // along with uVGAIII. If not, see <http://www.gnu.org/licenses/>. 00018 00019 #include "mbed.h" 00020 #include "uVGAIII.h" 00021 00022 #define ARRAY_SIZE(X) sizeof(X)/sizeof(X[0]) 00023 00024 //Serial pc(USBTX,USBRX); 00025 00026 //****************************************************************************************************** 00027 uVGAIII :: uVGAIII(PinName tx, PinName rx, PinName rst) : _cmd(tx, rx), 00028 _rst(rst) 00029 #if DEBUGMODE 00030 ,pc(USBTX, USBRX) 00031 #endif // DEBUGMODE 00032 { // Constructor 00033 00034 #if DEBUGMODE 00035 pc.baud(115200); 00036 00037 pc.printf("\n\n\n"); 00038 pc.printf("********************\n"); 00039 pc.printf("uVGAIII CONSTRUCTOR\n"); 00040 pc.printf("********************\n"); 00041 #endif 00042 _cmd.baud(9600); 00043 _rst = 1; // put RESET pin to high to start TFT screen 00044 00045 reset(); 00046 cls(); // clear screen 00047 speversion(); // get SPE version information 00048 pmmcversion(); // get PmmC version information 00049 00050 current_col = 0; // initial cursor col 00051 current_row = 0; // initial cursor row 00052 current_color = WHITE; // initial text color 00053 current_orientation = IS_LANDSCAPE; // initial screen orientation 00054 00055 set_font(FONT3); // initial font ( This line can be removed because font has been set to be FONT3 ) 00056 text_opacity(OPAQUE); // initial text opacity ( This line can be removed because text opacity has been set to be OPAQUE ) 00057 } 00058 00059 //****************************************************************************************************** 00060 void uVGAIII :: writeBYTE(char c) { // send a BYTE command to screen 00061 00062 _cmd.putc(c); 00063 wait_ms(1); 00064 00065 #if DEBUGMODE 00066 pc.printf(" Char sent : 0x%02X\n",c); 00067 #endif 00068 00069 } 00070 00071 //****************************************************************************************************** 00072 void uVGAIII :: freeBUFFER(void) { // Clear serial buffer before writing command 00073 00074 while (_cmd.readable()) _cmd.getc(); // clear buffer garbage 00075 } 00076 00077 //****************************************************************************************************** 00078 int uVGAIII :: writeCOMMAND(char *command, int commNum, int respNum) { // send several BYTES making a command and return an answer 00079 00080 #if DEBUGMODE 00081 pc.printf("\n"); 00082 pc.printf("New COMMAND : 0x%02X%02X\n", command[0], command[1]); 00083 #endif 00084 int i, resp = 0; 00085 freeBUFFER(); 00086 00087 for (i = 0; i < commNum; i++) {writeBYTE(command[i]); wait_ms(100);} // send command to serial port 00088 00089 for (i = 0; i < respNum; i++) { 00090 while (!_cmd.readable()) wait_ms(TEMPO); // wait for screen answer 00091 if (_cmd.readable()) { 00092 resp = _cmd.getc(); // read response if any 00093 #if DEBUGMODE 00094 pc.printf("response = 0x%02X\n",resp); 00095 #endif 00096 } 00097 } 00098 return resp; 00099 } 00100 00101 //************************************************************************** 00102 void uVGAIII :: reset() { // Reset Screen 00103 00104 _rst = 0; // put RESET pin to low 00105 wait_ms(TEMPO); // wait a few milliseconds for command reception 00106 _rst = 1; // put RESET back to high 00107 wait(3.2); // wait 3s for screen to restart 00108 00109 freeBUFFER(); // clean buffer from possible garbage 00110 00111 #if DEBUGMODE 00112 pc.printf("Reset completed.\n"); 00113 #endif 00114 } 00115 00116 //************************************************************************** 00117 void uVGAIII :: speversion() { // get SPE version 00118 char command[2] = ""; 00119 int spe = 0; 00120 command[0] = (SPEVERSION >> 8) & 0xFF; 00121 command[1] = SPEVERSION & 0xFF; 00122 00123 spe = readVERSION(command, 2); 00124 00125 pc.printf("spe:%d\n",spe); 00126 } 00127 00128 //************************************************************************** 00129 void uVGAIII :: pmmcversion() { // get PmmC version 00130 char command[2] = ""; 00131 int pmmc = 0; 00132 command[0] = (PMMCVERSION >> 8) & 0xFF; 00133 command[1] = PMMCVERSION & 0xFF; 00134 00135 pmmc = readVERSION(command, 2); 00136 00137 pc.printf("pmmc:%d\n",pmmc); 00138 } 00139 00140 //************************************************************************** 00141 void uVGAIII :: baudrate(int speed) { // set screen baud rate 00142 char command[4]= ""; 00143 command[0] = ( BAUDRATE >> 8 ) & 0xFF; 00144 command[1] = BAUDRATE & 0xFF; 00145 switch (speed) { 00146 case 110 : 00147 command[2] = ( BAUD_110 >> 8 ) & 0xFF; 00148 command[3] = BAUD_110 & 0xFF; 00149 break; 00150 case 300 : 00151 command[2] = ( BAUD_300 >> 8 ) & 0xFF; 00152 command[3] = BAUD_300 & 0xFF; 00153 break; 00154 case 600 : 00155 command[2] = ( BAUD_600 >> 8 ) & 0xFF; 00156 command[3] = BAUD_600 & 0xFF; 00157 break; 00158 case 1200 : 00159 command[2] = ( BAUD_1200>> 8 ) & 0xFF; 00160 command[3] = BAUD_1200 & 0xFF; 00161 break; 00162 case 2400 : 00163 command[2] = ( BAUD_2400 >> 8 ) & 0xFF; 00164 command[3] = BAUD_2400 & 0xFF; 00165 break; 00166 case 4800 : 00167 command[2] = ( BAUD_4800 >> 8 ) & 0xFF; 00168 command[3] = BAUD_4800 & 0xFF; 00169 break; 00170 case 9600 : 00171 command[2] = ( BAUD_9600 >> 8 ) & 0xFF; 00172 command[3] = BAUD_9600 & 0xFF; 00173 break; 00174 case 14400 : 00175 command[2] = ( BAUD_9600 >> 8 ) & 0xFF; 00176 command[3] = BAUD_9600 & 0xFF; 00177 break; 00178 case 19200 : 00179 command[2] = ( BAUD_19200 >> 8 ) & 0xFF; 00180 command[3] = BAUD_19200 & 0xFF; 00181 break; 00182 case 31250 : 00183 command[2] = ( BAUD_31250 >> 8 ) & 0xFF; 00184 command[3] = BAUD_31250 & 0xFF; 00185 break; 00186 case 38400 : 00187 command[2] = ( BAUD_38400 >> 8 ) & 0xFF; 00188 command[3] = BAUD_38400 & 0xFF; 00189 break; 00190 case 56000 : 00191 command[2] = ( BAUD_56000 >> 8 ) & 0xFF; 00192 command[3] = BAUD_56000 & 0xFF; 00193 break; 00194 case 57600 : 00195 command[2] = ( BAUD_57600 >> 8 ) & 0xFF; 00196 command[3] = BAUD_57600 & 0xFF; 00197 break; 00198 case 115200 : 00199 command[2] = ( BAUD_115200 >> 8 ) & 0xFF; 00200 command[3] = BAUD_115200 & 0xFF; 00201 break; 00202 case 128000 : 00203 command[2] = ( BAUD_128000 >> 8 ) & 0xFF; 00204 command[3] = BAUD_128000 & 0xFF; 00205 break; 00206 case 256000 : 00207 command[2] = ( BAUD_256000 >> 8 ) & 0xFF; 00208 command[3] = BAUD_256000 & 0xFF; 00209 break; 00210 default : 00211 command[2] = ( BAUD_9600 >> 8 ) & 0xFF; 00212 command[3] = BAUD_9600 & 0xFF; 00213 speed = 9600; 00214 break; 00215 } 00216 #if DEBUGMODE 00217 pc.printf("\n"); 00218 pc.printf("New COMMAND : 0x%02X%02X\n", command[0], command[1]); 00219 #endif 00220 int i, resp = 0; 00221 freeBUFFER(); 00222 00223 for (i = 0; i < 4; i++) writeBYTE(command[i]); // send command to serial port 00224 wait_ms(5); 00225 _cmd.baud(speed); // set mbed to same speed 00226 00227 while (!_cmd.readable()) wait_ms(TEMPO); // wait for screen answer 00228 if (_cmd.readable()) resp = _cmd.getc(); // read response if any 00229 00230 #if DEBUGMODE 00231 pc.printf("response = 0x%02X\n",resp); 00232 #endif 00233 00234 #if DEBUGMODE 00235 pc.printf("Set baudrate completed.\n"); 00236 #endif 00237 } 00238 00239 //****************************************************************************************************** 00240 int uVGAIII :: readVERSION(char *command, int number) { // read screen info and populate data 00241 00242 int i, temp = 0, resp = 0; 00243 char response[5] = ""; 00244 00245 #if DEBUGMODE 00246 pc.printf("\n"); 00247 pc.printf("New COMMAND : 0x%02X%02X\n", command[0], command[1]); 00248 #endif 00249 00250 freeBUFFER(); 00251 00252 for (i = 0; i < number; i++) writeBYTE(command[i]); // send all chars to serial port 00253 00254 while (!_cmd.readable()) wait_ms(TEMPO); // wait for screen answer 00255 00256 while (_cmd.readable() && resp < ARRAY_SIZE(response)) { 00257 temp = _cmd.getc(); 00258 response[resp] = (char)temp; 00259 pc.printf("response = 0x%02X\n",response[resp]); 00260 resp++; 00261 } 00262 switch (resp) { 00263 case 3 : // if OK populate data and return version 00264 version = int(response[1]) << 8 | response[2]; 00265 break; 00266 default : 00267 version = 0; // return 0 00268 break; 00269 } 00270 return version; 00271 } 00272 00273 //**************************************************************************************************** 00274 void uVGAIII :: set_volume(char value) { // set sound volume to value 00275 char command[4] = ""; 00276 00277 command[0] = (SETVOLUME >> 8) & 0xFF; 00278 command[1] = SETVOLUME & 0xFF; 00279 00280 command[2] = 0; 00281 command[3] = value; 00282 00283 writeCOMMAND(command, 4, 1); 00284 00285 #if DEBUGMODE 00286 pc.printf("Sound volume completed.\n"); 00287 #endif 00288 } 00289 00290 //****************************************************************************************************** 00291 void uVGAIII :: getTOUCH(char *command, int number, int *xy) { // read screen info and populate data 00292 00293 #if DEBUGMODE 00294 pc.printf("\n"); 00295 pc.printf("New COMMAND : 0x%02X%02X\n", command[0], command[1]); 00296 #endif 00297 int i, temp = 0, resp = 0; 00298 char response[5] = ""; 00299 00300 freeBUFFER(); 00301 00302 for (i = 0; i < number; i++) writeBYTE(command[i]); // send all chars to serial port 00303 00304 while (!_cmd.readable()) wait_ms(TEMPO); // wait for screen answer 00305 00306 while (_cmd.readable() && resp < ARRAY_SIZE(response)) { 00307 temp = _cmd.getc(); 00308 response[resp++] = (char)temp; 00309 } 00310 00311 #if DEBUGMODE 00312 pc.printf(" Answer received %d : 0x%02X 0x%02X 0x%02X\n", resp, response[0], response[1], response[2]); 00313 #endif 00314 00315 switch (resp) { 00316 case 3 : // if OK populate data 00317 *xy = ((response[1]<<8)+ response[2]) * (response[1] != 0xFF); 00318 break; 00319 default : 00320 *xy = -1; 00321 break; 00322 } 00323 00324 #if DEBUGMODE 00325 pc.printf(" X or Y : %03d\n", *xy); 00326 #endif 00327 } 00328 00329 //****************************************************************************************************** 00330 int uVGAIII :: getSTATUS(char *command, int number) { // read screen info and populate data 00331 00332 #if DEBUGMODE 00333 pc.printf("\n"); 00334 pc.printf("New COMMAND : 0x%02X%02X\n", command[0], command[1]); 00335 #endif 00336 00337 int i, temp = 0, resp = 0; 00338 char response[5] = ""; 00339 00340 freeBUFFER(); 00341 00342 for (i = 0; i < number; i++) writeBYTE(command[i]); // send all chars to serial port 00343 00344 while (!_cmd.readable()) wait_ms(TEMPO); // wait for screen answer 00345 00346 while (_cmd.readable() && resp < ARRAY_SIZE(response)) { 00347 temp = _cmd.getc(); 00348 response[resp++] = (char)temp; 00349 } 00350 switch (resp) { 00351 case 3 : 00352 resp = (int)response[2]; // if OK populate data 00353 break; 00354 default : 00355 resp = -1; // else return 0 00356 break; 00357 } 00358 00359 #if DEBUGMODE 00360 pc.printf(" Answer received : %d\n", resp); 00361 #endif 00362 00363 return resp; 00364 }
Generated on Tue Jul 12 2022 17:04:14 by
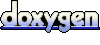