Library for uVGAIII
Embed:
(wiki syntax)
Show/hide line numbers
uVGAIII_Text.cpp
00001 // 00002 // uVGAIII is a class to drive 4D Systems TFT touch screens 00003 // 00004 // Copyright (C) <2010> Stephane ROCHON <stephane.rochon at free.fr> 00005 // 00006 // uVGAIII is free software: you can redistribute it and/or modify 00007 // it under the terms of the GNU General Public License as published by 00008 // the Free Software Foundation, either version 3 of the License, or 00009 // (at your option) any later version. 00010 // 00011 // uVGAIII is distributed in the hope that it will be useful, 00012 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 // GNU General Public License for more details. 00015 // 00016 // You should have received a copy of the GNU General Public License 00017 // along with uVGAIII. If not, see <http://www.gnu.org/licenses/>. 00018 00019 #include "mbed.h" 00020 #include "uVGAIII.h" 00021 00022 //**************************************************************************************************** 00023 void uVGAIII :: set_font(char mode) { // set font size 00024 char command[4] = ""; 00025 00026 command[0] = (SETFONT >> 8) & 0xFF; 00027 command[1] = SETFONT & 0xFF; 00028 command[2] = 0; 00029 command[3] = mode; 00030 00031 current_font = mode; 00032 00033 if (current_orientation == IS_PORTRAIT) { 00034 current_w = SIZE_X; 00035 current_h = SIZE_Y; 00036 } else { 00037 current_w = SIZE_Y; 00038 current_h = SIZE_X; 00039 } 00040 00041 switch (mode) { 00042 case FONT1 : 00043 current_fx = 7; 00044 current_fy = 8; 00045 break; 00046 case FONT2 : 00047 current_fx = 8; 00048 current_fy = 8; 00049 break; 00050 case FONT3 : 00051 current_fx = 8; 00052 current_fy = 12; 00053 break; 00054 } 00055 00056 max_col = current_w / current_fx; 00057 max_row = current_h / current_fy; 00058 00059 writeCOMMAND(command, 4, 3); 00060 00061 #if DEBUGMODE 00062 pc.printf("Set font completed.\n"); 00063 #endif 00064 } 00065 00066 //**************************************************************************************************** 00067 void uVGAIII :: char_width(char c) { // Get the width in pixel units for a character 00068 char command[3] = ""; 00069 00070 command[0] = (CHARWIDTH >> 8) & 0xFF; 00071 command[1] = CHARWIDTH & 0xFF; 00072 00073 command[2] = c; 00074 00075 writeCOMMAND(command, 3, 3); 00076 00077 #if DEBUGMODE 00078 pc.printf("Character width completed.\n"); 00079 #endif 00080 } 00081 00082 //**************************************************************************************************** 00083 void uVGAIII :: char_height(char c) { // Get the height in pixel units for a character 00084 char command[3] = ""; 00085 00086 command[0] = (CHARHEIGHT >> 8) & 0xFF; 00087 command[1] = CHARHEIGHT & 0xFF; 00088 00089 command[2] = c; 00090 00091 writeCOMMAND(command, 3, 3); 00092 00093 #if DEBUGMODE 00094 pc.printf("Character height completed.\n"); 00095 #endif 00096 } 00097 00098 //**************************************************************************************************** 00099 void uVGAIII :: text_opacity(char mode) { // set text mode 00100 char command[4] = ""; 00101 00102 command[0] = (TEXTOPACITY >> 8) & 0xFF; 00103 command[1] = TEXTOPACITY & 0xFF; 00104 command[2] = 0x00; 00105 command[3] = mode; 00106 00107 writeCOMMAND(command, 4, 3); 00108 00109 #if DEBUGMODE 00110 pc.printf("Text opacity completed.\n"); 00111 #endif 00112 } 00113 00114 //**************************************************************************************************** 00115 void uVGAIII :: text_width(int multiplier) { // Set teh text width multiplier between 1 and 16 00116 char command[4] = ""; 00117 00118 command[0] = (TEXTWIDTH >> 8) & 0xFF; 00119 command[1] = TEXTWIDTH & 0xFF; 00120 00121 command[2] = (multiplier >> 8) & 0xFF; 00122 command[3] = multiplier & 0xFF; 00123 00124 writeCOMMAND(command, 4, 3); 00125 00126 #if DEBUGMODE 00127 pc.printf("Text width completed.\n"); 00128 #endif 00129 } 00130 00131 //**************************************************************************************************** 00132 void uVGAIII :: text_height(int multiplier) { // Set teh text height multiplier between 1 and 16 00133 char command[4] = ""; 00134 00135 command[0] = (TEXTHEIGHT >> 8) & 0xFF; 00136 command[1] = TEXTHEIGHT & 0xFF; 00137 00138 command[2] = (multiplier >> 8) & 0xFF; 00139 command[3] = multiplier & 0xFF; 00140 00141 writeCOMMAND(command, 4, 3); 00142 00143 #if DEBUGMODE 00144 pc.printf("Text width completed.\n"); 00145 #endif 00146 } 00147 00148 //**************************************************************************************************** 00149 void uVGAIII :: text_y_gap(int pixelcount) { // set the pixel gap between characters(y-axis), and the gap is in pixel units 00150 char command[4] = ""; 00151 00152 command[0] = (TEXTYGAP >> 8) & 0xFF; 00153 command[1] = TEXTYGAP & 0xFF; 00154 00155 command[2] = (pixelcount >> 8) & 0xFF; 00156 command[3] = pixelcount & 0xFF; 00157 00158 writeCOMMAND(command, 4, 3); 00159 00160 #if DEBUGMODE 00161 pc.printf("Text Y-gap completed.\n"); 00162 #endif 00163 } 00164 00165 //**************************************************************************************************** 00166 void uVGAIII :: text_x_gap(int pixelcount) { // set the pixel gap between characters(x-axis), and the gap is in pixel units 00167 char command[4] = ""; 00168 00169 command[0] = (TEXTXGAP >> 8) & 0xFF; 00170 command[1] = TEXTXGAP & 0xFF; 00171 00172 command[2] = (pixelcount >> 8) & 0xFF; 00173 command[3] = pixelcount & 0xFF; 00174 00175 writeCOMMAND(command, 4, 3); 00176 00177 #if DEBUGMODE 00178 pc.printf("Text X-gap completed.\n"); 00179 #endif 00180 } 00181 00182 //**************************************************************************************************** 00183 void uVGAIII :: text_bold(char mode) { // set the Bold attribute for the text 00184 char command[4] = ""; 00185 00186 command[0] = (TEXTBOLD >> 8) & 0xFF; 00187 command[1] = TEXTBOLD & 0xFF; 00188 00189 command[2] = 0x00; 00190 command[3] = mode & 0xFF; 00191 00192 writeCOMMAND(command, 4, 3); 00193 00194 #if DEBUGMODE 00195 pc.printf("Text bold completed.\n"); 00196 #endif 00197 } 00198 00199 //**************************************************************************************************** 00200 void uVGAIII :: text_inverse(char mode) { // set the text foreground and background color to be inverse 00201 char command[4] = ""; 00202 00203 command[0] = (TEXTINVERSE >> 8) & 0xFF; 00204 command[1] = TEXTINVERSE & 0xFF; 00205 00206 command[2] = 0x00; 00207 command[3] = mode & 0xFF; 00208 00209 writeCOMMAND(command, 4, 3); 00210 00211 #if DEBUGMODE 00212 pc.printf("Text inverse completed.\n"); 00213 #endif 00214 } 00215 00216 //**************************************************************************************************** 00217 void uVGAIII :: text_italic(char mode) { // set the text to italic 00218 char command[4] = ""; 00219 00220 command[0] = (TEXTITALIC >> 8) & 0xFF; 00221 command[1] = TEXTITALIC & 0xFF; 00222 00223 command[2] = 0x00; 00224 command[3] = mode & 0xFF; 00225 00226 writeCOMMAND(command, 4, 3); 00227 00228 #if DEBUGMODE 00229 pc.printf("Text italic completed.\n"); 00230 #endif 00231 } 00232 00233 //**************************************************************************************************** 00234 void uVGAIII :: text_underline(char mode) { // Set whether the text to be underlined 00235 char command[4] = ""; // For text underline to work, text Y-gap must be set to at least 2 00236 00237 command[0] = (TEXTUNDLINE >> 8) & 0xFF; 00238 command[1] = TEXTUNDLINE & 0xFF; 00239 00240 command[2] = 0x00; 00241 command[3] = mode & 0xFF; 00242 00243 writeCOMMAND(command, 4, 3); 00244 00245 #if DEBUGMODE 00246 pc.printf("Text underline completed.\n"); 00247 #endif 00248 } 00249 00250 //**************************************************************************************************** 00251 void uVGAIII :: text_attributes(int value) { // Set text attributes: bold, italic, inverse, underlined 00252 char command[4] = ""; // For text underline to work, text Y-gap must be set to at least 2 00253 00254 command[0] = (TEXTATTRIBU >> 8) & 0xFF; 00255 command[1] = TEXTATTRIBU & 0xFF; 00256 00257 command[2] = (value >> 8) & 0xFF; 00258 command[3] = value & 0xFF; 00259 00260 writeCOMMAND(command, 4, 3); 00261 00262 #if DEBUGMODE 00263 pc.printf("Text attributes completed.\n"); 00264 #endif 00265 } 00266 00267 //**************************************************************************************************** 00268 void uVGAIII :: move_cursor(int line, int column) { // move cursor 00269 char command[6] = ""; 00270 00271 command[0] = (MOVECURSOR >> 8) & 0xFF; 00272 command[1] = MOVECURSOR & 0xFF; 00273 00274 command[2] = (line >> 8) & 0xFF; 00275 command[3] = line & 0xFF; 00276 00277 command[4] = (column >> 8) & 0xFF; 00278 command[5] = column & 0xFF; 00279 00280 writeCOMMAND(command, 6, 1); 00281 current_row = line; 00282 current_col = column; 00283 00284 #if DEBUGMODE 00285 pc.printf("Move cursor completed.\n"); 00286 #endif 00287 } 00288 00289 //**************************************************************************************************** 00290 void uVGAIII :: put_char(char c) { // draw a text char 00291 char command[4] = ""; 00292 00293 command[0] = (PUTCHAR >> 8) & 0xFF; 00294 command[1] = PUTCHAR & 0xFF; 00295 00296 command[2] = 0x00; 00297 command[3] = c; 00298 00299 writeCOMMAND(command, 4, 1); 00300 00301 #if DEBUGMODE 00302 pc.printf("Put character completed.\n"); 00303 #endif 00304 } 00305 00306 //**************************************************************************************************** 00307 void uVGAIII :: put_string(char *s) { // draw a text string 00308 00309 char command[1000]= ""; 00310 int size = strlen(s); 00311 int i = 0; 00312 00313 command[0] = (PUTSTRING >> 8) & 0xFF; 00314 command[1] = PUTSTRING & 0xFF; 00315 00316 for (i=0; i<size; i++) command[2+i] = s[i]; 00317 00318 command[2+size] = 0; 00319 00320 writeCOMMAND(command, 3 + size, 1); 00321 00322 #if DEBUGMODE 00323 pc.printf("Put string completed.\n"); 00324 #endif 00325 } 00326 00327 //**************************************************************************************************** 00328 void uVGAIII :: text_fgd_color(int color) { // Set text foreground color 00329 char command[4] = ""; 00330 00331 command[0] = (TEXTFGCOLOR >> 8) & 0xFF; 00332 command[1] = TEXTFGCOLOR & 0xFF; 00333 00334 current_color = color; 00335 00336 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00337 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00338 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00339 00340 command[2] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00341 command[3] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00342 00343 writeCOMMAND(command, 4, 3); 00344 00345 #if DEBUGMODE 00346 pc.printf("Set text foreground color completed.\n"); 00347 #endif 00348 } 00349 00350 //**************************************************************************************************** 00351 void uVGAIII :: text_bgd_color(int color) { // Set text background color 00352 char command[4] = ""; 00353 00354 command[0] = (TEXTBGCOLOR >> 8) & 0xFF; 00355 command[1] = TEXTBGCOLOR & 0xFF; 00356 00357 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00358 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00359 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00360 00361 command[2] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00362 command[3] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00363 00364 writeCOMMAND(command, 4, 3); 00365 00366 #if DEBUGMODE 00367 pc.printf("Set text foreground color completed.\n"); 00368 #endif 00369 } 00370 00371 //**************************************************************************************************** 00372 void uVGAIII :: putc(char c) // place char at current cursor position 00373 //used by virtual printf function _putc 00374 { 00375 00376 pc.printf("\nCursor position: %d, %d",current_row,current_col); 00377 if(c<0x20) { 00378 if(c=='\n') { 00379 current_col = 0; 00380 current_row++; 00381 move_cursor(current_row, current_col); 00382 } 00383 if(c=='\r') { 00384 current_col = 0; 00385 move_cursor(current_row, current_col); 00386 } 00387 if(c=='\f') { 00388 cls(); //clear screen on form feed 00389 } 00390 } else { 00391 put_char(c); 00392 current_col++; 00393 move_cursor(current_row, current_col); 00394 } 00395 if (current_col >= max_col) { 00396 current_col = 0; 00397 current_row++; 00398 move_cursor(current_row, current_col); 00399 } 00400 if (current_row >= max_row) { 00401 current_row = 0; 00402 move_cursor(current_row, current_col); 00403 } 00404 } 00405 00406 //**************************************************************************************************** 00407 void uVGAIII :: puts(char *s) { // place string at current cursor position 00408 00409 put_string(s); 00410 00411 current_col += strlen(s); 00412 00413 if (current_col >= max_col) { 00414 current_row += current_col / max_col; 00415 current_col %= max_col; 00416 } 00417 if (current_row >= max_row) { 00418 current_row %= max_row; 00419 } 00420 }
Generated on Tue Jul 12 2022 17:04:14 by
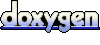