
Example program with HTTPServer and sensor data streaming over TCPSockets, using Donatien Garnier's Net APIs and services code on top of LWIP. Files StreamServer.h and .cpp encapsulate streaming over TCPSockets. Broadcast is done by sendToAll(), and all incoming data is echoed back to the client. Echo code can be replaced with some remote control of the streaming interface. See main() that shows how to periodically send some data to all subscribed clients. To subscribe, a client should open a socket at <mbed_ip> port 123. I used few lines in TCL code to set up a quick sink for the data. HTTP files are served on port 80 concurrently to the streaming.
opt.h
00001 /** 00002 * @file 00003 * 00004 * lwIP Options Configuration 00005 */ 00006 00007 /* 00008 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00009 * All rights reserved. 00010 * 00011 * Redistribution and use in source and binary forms, with or without modification, 00012 * are permitted provided that the following conditions are met: 00013 * 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. The name of the author may not be used to endorse or promote products 00020 * derived from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00023 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00024 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00025 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00026 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00027 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00028 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00029 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00030 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00031 * OF SUCH DAMAGE. 00032 * 00033 * This file is part of the lwIP TCP/IP stack. 00034 * 00035 * Author: Adam Dunkels <adam@sics.se> 00036 * 00037 */ 00038 #ifndef __LWIP_OPT_H__ 00039 #define __LWIP_OPT_H__ 00040 00041 /* 00042 * Include user defined options first. Anything not defined in these files 00043 * will be set to standard values. Override anything you dont like! 00044 */ 00045 #include "lwipopts.h" 00046 #include "lwip/debug.h" 00047 00048 /* 00049 ----------------------------------------------- 00050 ---------- Platform specific locking ---------- 00051 ----------------------------------------------- 00052 */ 00053 00054 /** 00055 * SYS_LIGHTWEIGHT_PROT==1: if you want inter-task protection for certain 00056 * critical regions during buffer allocation, deallocation and memory 00057 * allocation and deallocation. 00058 */ 00059 #ifndef SYS_LIGHTWEIGHT_PROT 00060 #define SYS_LIGHTWEIGHT_PROT 0 00061 #endif 00062 00063 /** 00064 * NO_SYS==1: Provides VERY minimal functionality. Otherwise, 00065 * use lwIP facilities. 00066 */ 00067 #ifndef NO_SYS 00068 #define NO_SYS 0 00069 #endif 00070 00071 /** 00072 * MEMCPY: override this if you have a faster implementation at hand than the 00073 * one included in your C library 00074 */ 00075 #ifndef MEMCPY 00076 #define MEMCPY(dst,src,len) memcpy(dst,src,len) 00077 #endif 00078 00079 /** 00080 * SMEMCPY: override this with care! Some compilers (e.g. gcc) can inline a 00081 * call to memcpy() if the length is known at compile time and is small. 00082 */ 00083 #ifndef SMEMCPY 00084 #define SMEMCPY(dst,src,len) memcpy(dst,src,len) 00085 #endif 00086 00087 /* 00088 ------------------------------------ 00089 ---------- Memory options ---------- 00090 ------------------------------------ 00091 */ 00092 /** 00093 * MEM_LIBC_MALLOC==1: Use malloc/free/realloc provided by your C-library 00094 * instead of the lwip internal allocator. Can save code size if you 00095 * already use it. 00096 */ 00097 #ifndef MEM_LIBC_MALLOC 00098 #define MEM_LIBC_MALLOC 0 00099 #endif 00100 00101 /** 00102 * MEMP_MEM_MALLOC==1: Use mem_malloc/mem_free instead of the lwip pool allocator. 00103 * Especially useful with MEM_LIBC_MALLOC but handle with care regarding execution 00104 * speed and usage from interrupts! 00105 */ 00106 #ifndef MEMP_MEM_MALLOC 00107 #define MEMP_MEM_MALLOC 0 00108 #endif 00109 00110 /** 00111 * MEM_ALIGNMENT: should be set to the alignment of the CPU 00112 * 4 byte alignment -> #define MEM_ALIGNMENT 4 00113 * 2 byte alignment -> #define MEM_ALIGNMENT 2 00114 */ 00115 #ifndef MEM_ALIGNMENT 00116 #define MEM_ALIGNMENT 1 00117 #endif 00118 00119 /** 00120 * MEM_SIZE: the size of the heap memory. If the application will send 00121 * a lot of data that needs to be copied, this should be set high. 00122 */ 00123 #ifndef MEM_SIZE 00124 #define MEM_SIZE 1600 00125 #endif 00126 00127 /** 00128 * MEMP_SEPARATE_POOLS: if defined to 1, each pool is placed in its own array. 00129 * This can be used to individually change the location of each pool. 00130 * Default is one big array for all pools 00131 */ 00132 #ifndef MEMP_SEPARATE_POOLS 00133 #define MEMP_SEPARATE_POOLS 0 00134 #endif 00135 00136 /** 00137 * MEMP_OVERFLOW_CHECK: memp overflow protection reserves a configurable 00138 * amount of bytes before and after each memp element in every pool and fills 00139 * it with a prominent default value. 00140 * MEMP_OVERFLOW_CHECK == 0 no checking 00141 * MEMP_OVERFLOW_CHECK == 1 checks each element when it is freed 00142 * MEMP_OVERFLOW_CHECK >= 2 checks each element in every pool every time 00143 * memp_malloc() or memp_free() is called (useful but slow!) 00144 */ 00145 #ifndef MEMP_OVERFLOW_CHECK 00146 #define MEMP_OVERFLOW_CHECK 0 00147 #endif 00148 00149 /** 00150 * MEMP_SANITY_CHECK==1: run a sanity check after each memp_free() to make 00151 * sure that there are no cycles in the linked lists. 00152 */ 00153 #ifndef MEMP_SANITY_CHECK 00154 #define MEMP_SANITY_CHECK 0 00155 #endif 00156 00157 /** 00158 * MEM_USE_POOLS==1: Use an alternative to malloc() by allocating from a set 00159 * of memory pools of various sizes. When mem_malloc is called, an element of 00160 * the smallest pool that can provide the length needed is returned. 00161 * To use this, MEMP_USE_CUSTOM_POOLS also has to be enabled. 00162 */ 00163 #ifndef MEM_USE_POOLS 00164 #define MEM_USE_POOLS 0 00165 #endif 00166 00167 /** 00168 * MEM_USE_POOLS_TRY_BIGGER_POOL==1: if one malloc-pool is empty, try the next 00169 * bigger pool - WARNING: THIS MIGHT WASTE MEMORY but it can make a system more 00170 * reliable. */ 00171 #ifndef MEM_USE_POOLS_TRY_BIGGER_POOL 00172 #define MEM_USE_POOLS_TRY_BIGGER_POOL 0 00173 #endif 00174 00175 /** 00176 * MEMP_USE_CUSTOM_POOLS==1: whether to include a user file lwippools.h 00177 * that defines additional pools beyond the "standard" ones required 00178 * by lwIP. If you set this to 1, you must have lwippools.h in your 00179 * inlude path somewhere. 00180 */ 00181 #ifndef MEMP_USE_CUSTOM_POOLS 00182 #define MEMP_USE_CUSTOM_POOLS 0 00183 #endif 00184 00185 /** 00186 * Set this to 1 if you want to free PBUF_RAM pbufs (or call mem_free()) from 00187 * interrupt context (or another context that doesn't allow waiting for a 00188 * semaphore). 00189 * If set to 1, mem_malloc will be protected by a semaphore and SYS_ARCH_PROTECT, 00190 * while mem_free will only use SYS_ARCH_PROTECT. mem_malloc SYS_ARCH_UNPROTECTs 00191 * with each loop so that mem_free can run. 00192 * 00193 * ATTENTION: As you can see from the above description, this leads to dis-/ 00194 * enabling interrupts often, which can be slow! Also, on low memory, mem_malloc 00195 * can need longer. 00196 * 00197 * If you don't want that, at least for NO_SYS=0, you can still use the following 00198 * functions to enqueue a deallocation call which then runs in the tcpip_thread 00199 * context: 00200 * - pbuf_free_callback(p); 00201 * - mem_free_callback(m); 00202 */ 00203 #ifndef LWIP_ALLOW_MEM_FREE_FROM_OTHER_CONTEXT 00204 #define LWIP_ALLOW_MEM_FREE_FROM_OTHER_CONTEXT 0 00205 #endif 00206 00207 /* 00208 ------------------------------------------------ 00209 ---------- Internal Memory Pool Sizes ---------- 00210 ------------------------------------------------ 00211 */ 00212 /** 00213 * MEMP_NUM_PBUF: the number of memp struct pbufs (used for PBUF_ROM and PBUF_REF). 00214 * If the application sends a lot of data out of ROM (or other static memory), 00215 * this should be set high. 00216 */ 00217 #ifndef MEMP_NUM_PBUF 00218 #define MEMP_NUM_PBUF 16 00219 #endif 00220 00221 /** 00222 * MEMP_NUM_RAW_PCB: Number of raw connection PCBs 00223 * (requires the LWIP_RAW option) 00224 */ 00225 #ifndef MEMP_NUM_RAW_PCB 00226 #define MEMP_NUM_RAW_PCB 4 00227 #endif 00228 00229 /** 00230 * MEMP_NUM_UDP_PCB: the number of UDP protocol control blocks. One 00231 * per active UDP "connection". 00232 * (requires the LWIP_UDP option) 00233 */ 00234 #ifndef MEMP_NUM_UDP_PCB 00235 #define MEMP_NUM_UDP_PCB 4 00236 #endif 00237 00238 /** 00239 * MEMP_NUM_TCP_PCB: the number of simulatenously active TCP connections. 00240 * (requires the LWIP_TCP option) 00241 */ 00242 #ifndef MEMP_NUM_TCP_PCB 00243 #define MEMP_NUM_TCP_PCB 5 00244 #endif 00245 00246 /** 00247 * MEMP_NUM_TCP_PCB_LISTEN: the number of listening TCP connections. 00248 * (requires the LWIP_TCP option) 00249 */ 00250 #ifndef MEMP_NUM_TCP_PCB_LISTEN 00251 #define MEMP_NUM_TCP_PCB_LISTEN 8 00252 #endif 00253 00254 /** 00255 * MEMP_NUM_TCP_SEG: the number of simultaneously queued TCP segments. 00256 * (requires the LWIP_TCP option) 00257 */ 00258 #ifndef MEMP_NUM_TCP_SEG 00259 #define MEMP_NUM_TCP_SEG 16 00260 #endif 00261 00262 /** 00263 * MEMP_NUM_REASSDATA: the number of simultaneously IP packets queued for 00264 * reassembly (whole packets, not fragments!) 00265 */ 00266 #ifndef MEMP_NUM_REASSDATA 00267 #define MEMP_NUM_REASSDATA 5 00268 #endif 00269 00270 /** 00271 * MEMP_NUM_ARP_QUEUE: the number of simulateously queued outgoing 00272 * packets (pbufs) that are waiting for an ARP request (to resolve 00273 * their destination address) to finish. 00274 * (requires the ARP_QUEUEING option) 00275 */ 00276 #ifndef MEMP_NUM_ARP_QUEUE 00277 #define MEMP_NUM_ARP_QUEUE 30 00278 #endif 00279 00280 /** 00281 * MEMP_NUM_IGMP_GROUP: The number of multicast groups whose network interfaces 00282 * can be members et the same time (one per netif - allsystems group -, plus one 00283 * per netif membership). 00284 * (requires the LWIP_IGMP option) 00285 */ 00286 #ifndef MEMP_NUM_IGMP_GROUP 00287 #define MEMP_NUM_IGMP_GROUP 8 00288 #endif 00289 00290 /** 00291 * MEMP_NUM_SYS_TIMEOUT: the number of simulateously active timeouts. 00292 * (requires NO_SYS==0) 00293 */ 00294 #ifndef MEMP_NUM_SYS_TIMEOUT 00295 #define MEMP_NUM_SYS_TIMEOUT 3 00296 #endif 00297 00298 /** 00299 * MEMP_NUM_NETBUF: the number of struct netbufs. 00300 * (only needed if you use the sequential API, like api_lib.c) 00301 */ 00302 #ifndef MEMP_NUM_NETBUF 00303 #define MEMP_NUM_NETBUF 2 00304 #endif 00305 00306 /** 00307 * MEMP_NUM_NETCONN: the number of struct netconns. 00308 * (only needed if you use the sequential API, like api_lib.c) 00309 */ 00310 #ifndef MEMP_NUM_NETCONN 00311 #define MEMP_NUM_NETCONN 4 00312 #endif 00313 00314 /** 00315 * MEMP_NUM_TCPIP_MSG_API: the number of struct tcpip_msg, which are used 00316 * for callback/timeout API communication. 00317 * (only needed if you use tcpip.c) 00318 */ 00319 #ifndef MEMP_NUM_TCPIP_MSG_API 00320 #define MEMP_NUM_TCPIP_MSG_API 8 00321 #endif 00322 00323 /** 00324 * MEMP_NUM_TCPIP_MSG_INPKT: the number of struct tcpip_msg, which are used 00325 * for incoming packets. 00326 * (only needed if you use tcpip.c) 00327 */ 00328 #ifndef MEMP_NUM_TCPIP_MSG_INPKT 00329 #define MEMP_NUM_TCPIP_MSG_INPKT 8 00330 #endif 00331 00332 /** 00333 * MEMP_NUM_SNMP_NODE: the number of leafs in the SNMP tree. 00334 */ 00335 #ifndef MEMP_NUM_SNMP_NODE 00336 #define MEMP_NUM_SNMP_NODE 50 00337 #endif 00338 00339 /** 00340 * MEMP_NUM_SNMP_ROOTNODE: the number of branches in the SNMP tree. 00341 * Every branch has one leaf (MEMP_NUM_SNMP_NODE) at least! 00342 */ 00343 #ifndef MEMP_NUM_SNMP_ROOTNODE 00344 #define MEMP_NUM_SNMP_ROOTNODE 30 00345 #endif 00346 00347 /** 00348 * MEMP_NUM_SNMP_VARBIND: the number of concurrent requests (does not have to 00349 * be changed normally) - 2 of these are used per request (1 for input, 00350 * 1 for output) 00351 */ 00352 #ifndef MEMP_NUM_SNMP_VARBIND 00353 #define MEMP_NUM_SNMP_VARBIND 2 00354 #endif 00355 00356 /** 00357 * MEMP_NUM_SNMP_VALUE: the number of OID or values concurrently used 00358 * (does not have to be changed normally) - 3 of these are used per request 00359 * (1 for the value read and 2 for OIDs - input and output) 00360 */ 00361 #ifndef MEMP_NUM_SNMP_VALUE 00362 #define MEMP_NUM_SNMP_VALUE 3 00363 #endif 00364 00365 /** 00366 * MEMP_NUM_NETDB: the number of concurrently running lwip_addrinfo() calls 00367 * (before freeing the corresponding memory using lwip_freeaddrinfo()). 00368 */ 00369 #ifndef MEMP_NUM_NETDB 00370 #define MEMP_NUM_NETDB 1 00371 #endif 00372 00373 /** 00374 * PBUF_POOL_SIZE: the number of buffers in the pbuf pool. 00375 */ 00376 #ifndef PBUF_POOL_SIZE 00377 #define PBUF_POOL_SIZE 16 00378 #endif 00379 00380 /* 00381 --------------------------------- 00382 ---------- ARP options ---------- 00383 --------------------------------- 00384 */ 00385 /** 00386 * LWIP_ARP==1: Enable ARP functionality. 00387 */ 00388 #ifndef LWIP_ARP 00389 #define LWIP_ARP 1 00390 #endif 00391 00392 /** 00393 * ARP_TABLE_SIZE: Number of active MAC-IP address pairs cached. 00394 */ 00395 #ifndef ARP_TABLE_SIZE 00396 #define ARP_TABLE_SIZE 10 00397 #endif 00398 00399 /** 00400 * ARP_QUEUEING==1: Outgoing packets are queued during hardware address 00401 * resolution. 00402 */ 00403 #ifndef ARP_QUEUEING 00404 #define ARP_QUEUEING 1 00405 #endif 00406 00407 /** 00408 * ETHARP_TRUST_IP_MAC==1: Incoming IP packets cause the ARP table to be 00409 * updated with the source MAC and IP addresses supplied in the packet. 00410 * You may want to disable this if you do not trust LAN peers to have the 00411 * correct addresses, or as a limited approach to attempt to handle 00412 * spoofing. If disabled, lwIP will need to make a new ARP request if 00413 * the peer is not already in the ARP table, adding a little latency. 00414 * The peer *is* in the ARP table if it requested our address before. 00415 * Also notice that this slows down input processing of every IP packet! 00416 */ 00417 #ifndef ETHARP_TRUST_IP_MAC 00418 #define ETHARP_TRUST_IP_MAC 0 00419 #endif 00420 00421 /** 00422 * ETHARP_SUPPORT_VLAN==1: support receiving ethernet packets with VLAN header. 00423 * Additionally, you can define ETHARP_VLAN_CHECK to an u16_t VLAN ID to check. 00424 * If ETHARP_VLAN_CHECK is defined, only VLAN-traffic for this VLAN is accepted. 00425 * If ETHARP_VLAN_CHECK is not defined, all traffic is accepted. 00426 */ 00427 #ifndef ETHARP_SUPPORT_VLAN 00428 #define ETHARP_SUPPORT_VLAN 0 00429 #endif 00430 00431 /** LWIP_ETHERNET==1: enable ethernet support for PPPoE even though ARP 00432 * might be disabled 00433 */ 00434 #ifndef LWIP_ETHERNET 00435 #define LWIP_ETHERNET (LWIP_ARP || PPPOE_SUPPORT) 00436 #endif 00437 00438 /** ETH_PAD_SIZE: number of bytes added before the ethernet header to ensure 00439 * alignment of payload after that header. Since the header is 14 bytes long, 00440 * without this padding e.g. addresses in the IP header will not be aligned 00441 * on a 32-bit boundary, so setting this to 2 can speed up 32-bit-platforms. 00442 */ 00443 #ifndef ETH_PAD_SIZE 00444 #define ETH_PAD_SIZE 0 00445 #endif 00446 00447 /* 00448 -------------------------------- 00449 ---------- IP options ---------- 00450 -------------------------------- 00451 */ 00452 /** 00453 * IP_FORWARD==1: Enables the ability to forward IP packets across network 00454 * interfaces. If you are going to run lwIP on a device with only one network 00455 * interface, define this to 0. 00456 */ 00457 #ifndef IP_FORWARD 00458 #define IP_FORWARD 0 00459 #endif 00460 00461 /** 00462 * IP_OPTIONS_ALLOWED: Defines the behavior for IP options. 00463 * IP_OPTIONS_ALLOWED==0: All packets with IP options are dropped. 00464 * IP_OPTIONS_ALLOWED==1: IP options are allowed (but not parsed). 00465 */ 00466 #ifndef IP_OPTIONS_ALLOWED 00467 #define IP_OPTIONS_ALLOWED 1 00468 #endif 00469 00470 /** 00471 * IP_REASSEMBLY==1: Reassemble incoming fragmented IP packets. Note that 00472 * this option does not affect outgoing packet sizes, which can be controlled 00473 * via IP_FRAG. 00474 */ 00475 #ifndef IP_REASSEMBLY 00476 #define IP_REASSEMBLY 1 00477 #endif 00478 00479 /** 00480 * IP_FRAG==1: Fragment outgoing IP packets if their size exceeds MTU. Note 00481 * that this option does not affect incoming packet sizes, which can be 00482 * controlled via IP_REASSEMBLY. 00483 */ 00484 #ifndef IP_FRAG 00485 #define IP_FRAG 1 00486 #endif 00487 00488 /** 00489 * IP_REASS_MAXAGE: Maximum time (in multiples of IP_TMR_INTERVAL - so seconds, normally) 00490 * a fragmented IP packet waits for all fragments to arrive. If not all fragments arrived 00491 * in this time, the whole packet is discarded. 00492 */ 00493 #ifndef IP_REASS_MAXAGE 00494 #define IP_REASS_MAXAGE 3 00495 #endif 00496 00497 /** 00498 * IP_REASS_MAX_PBUFS: Total maximum amount of pbufs waiting to be reassembled. 00499 * Since the received pbufs are enqueued, be sure to configure 00500 * PBUF_POOL_SIZE > IP_REASS_MAX_PBUFS so that the stack is still able to receive 00501 * packets even if the maximum amount of fragments is enqueued for reassembly! 00502 */ 00503 #ifndef IP_REASS_MAX_PBUFS 00504 #define IP_REASS_MAX_PBUFS 10 00505 #endif 00506 00507 /** 00508 * IP_FRAG_USES_STATIC_BUF==1: Use a static MTU-sized buffer for IP 00509 * fragmentation. Otherwise pbufs are allocated and reference the original 00510 * packet data to be fragmented. 00511 */ 00512 #ifndef IP_FRAG_USES_STATIC_BUF 00513 #define IP_FRAG_USES_STATIC_BUF 1 00514 #endif 00515 00516 /** 00517 * IP_FRAG_MAX_MTU: Assumed max MTU on any interface for IP frag buffer 00518 * (requires IP_FRAG_USES_STATIC_BUF==1) 00519 */ 00520 #if IP_FRAG_USES_STATIC_BUF && !defined(IP_FRAG_MAX_MTU) 00521 #define IP_FRAG_MAX_MTU 1500 00522 #endif 00523 00524 /** 00525 * IP_DEFAULT_TTL: Default value for Time-To-Live used by transport layers. 00526 */ 00527 #ifndef IP_DEFAULT_TTL 00528 #define IP_DEFAULT_TTL 255 00529 #endif 00530 00531 /** 00532 * IP_SOF_BROADCAST=1: Use the SOF_BROADCAST field to enable broadcast 00533 * filter per pcb on udp and raw send operations. To enable broadcast filter 00534 * on recv operations, you also have to set IP_SOF_BROADCAST_RECV=1. 00535 */ 00536 #ifndef IP_SOF_BROADCAST 00537 #define IP_SOF_BROADCAST 0 00538 #endif 00539 00540 /** 00541 * IP_SOF_BROADCAST_RECV (requires IP_SOF_BROADCAST=1) enable the broadcast 00542 * filter on recv operations. 00543 */ 00544 #ifndef IP_SOF_BROADCAST_RECV 00545 #define IP_SOF_BROADCAST_RECV 0 00546 #endif 00547 00548 /* 00549 ---------------------------------- 00550 ---------- ICMP options ---------- 00551 ---------------------------------- 00552 */ 00553 /** 00554 * LWIP_ICMP==1: Enable ICMP module inside the IP stack. 00555 * Be careful, disable that make your product non-compliant to RFC1122 00556 */ 00557 #ifndef LWIP_ICMP 00558 #define LWIP_ICMP 1 00559 #endif 00560 00561 /** 00562 * ICMP_TTL: Default value for Time-To-Live used by ICMP packets. 00563 */ 00564 #ifndef ICMP_TTL 00565 #define ICMP_TTL (IP_DEFAULT_TTL) 00566 #endif 00567 00568 /** 00569 * LWIP_BROADCAST_PING==1: respond to broadcast pings (default is unicast only) 00570 */ 00571 #ifndef LWIP_BROADCAST_PING 00572 #define LWIP_BROADCAST_PING 0 00573 #endif 00574 00575 /** 00576 * LWIP_MULTICAST_PING==1: respond to multicast pings (default is unicast only) 00577 */ 00578 #ifndef LWIP_MULTICAST_PING 00579 #define LWIP_MULTICAST_PING 0 00580 #endif 00581 00582 /* 00583 --------------------------------- 00584 ---------- RAW options ---------- 00585 --------------------------------- 00586 */ 00587 /** 00588 * LWIP_RAW==1: Enable application layer to hook into the IP layer itself. 00589 */ 00590 #ifndef LWIP_RAW 00591 #define LWIP_RAW 1 00592 #endif 00593 00594 /** 00595 * LWIP_RAW==1: Enable application layer to hook into the IP layer itself. 00596 */ 00597 #ifndef RAW_TTL 00598 #define RAW_TTL (IP_DEFAULT_TTL) 00599 #endif 00600 00601 /* 00602 ---------------------------------- 00603 ---------- DHCP options ---------- 00604 ---------------------------------- 00605 */ 00606 /** 00607 * LWIP_DHCP==1: Enable DHCP module. 00608 */ 00609 #ifndef LWIP_DHCP 00610 #define LWIP_DHCP 0 00611 #endif 00612 00613 /** 00614 * DHCP_DOES_ARP_CHECK==1: Do an ARP check on the offered address. 00615 */ 00616 #ifndef DHCP_DOES_ARP_CHECK 00617 #define DHCP_DOES_ARP_CHECK ((LWIP_DHCP) && (LWIP_ARP)) 00618 #endif 00619 00620 /* 00621 ------------------------------------ 00622 ---------- AUTOIP options ---------- 00623 ------------------------------------ 00624 */ 00625 /** 00626 * LWIP_AUTOIP==1: Enable AUTOIP module. 00627 */ 00628 #ifndef LWIP_AUTOIP 00629 #define LWIP_AUTOIP 0 00630 #endif 00631 00632 /** 00633 * LWIP_DHCP_AUTOIP_COOP==1: Allow DHCP and AUTOIP to be both enabled on 00634 * the same interface at the same time. 00635 */ 00636 #ifndef LWIP_DHCP_AUTOIP_COOP 00637 #define LWIP_DHCP_AUTOIP_COOP 0 00638 #endif 00639 00640 /** 00641 * LWIP_DHCP_AUTOIP_COOP_TRIES: Set to the number of DHCP DISCOVER probes 00642 * that should be sent before falling back on AUTOIP. This can be set 00643 * as low as 1 to get an AutoIP address very quickly, but you should 00644 * be prepared to handle a changing IP address when DHCP overrides 00645 * AutoIP. 00646 */ 00647 #ifndef LWIP_DHCP_AUTOIP_COOP_TRIES 00648 #define LWIP_DHCP_AUTOIP_COOP_TRIES 9 00649 #endif 00650 00651 /* 00652 ---------------------------------- 00653 ---------- SNMP options ---------- 00654 ---------------------------------- 00655 */ 00656 /** 00657 * LWIP_SNMP==1: Turn on SNMP module. UDP must be available for SNMP 00658 * transport. 00659 */ 00660 #ifndef LWIP_SNMP 00661 #define LWIP_SNMP 0 00662 #endif 00663 00664 /** 00665 * SNMP_CONCURRENT_REQUESTS: Number of concurrent requests the module will 00666 * allow. At least one request buffer is required. 00667 * Does not have to be changed unless external MIBs answer request asynchronously 00668 */ 00669 #ifndef SNMP_CONCURRENT_REQUESTS 00670 #define SNMP_CONCURRENT_REQUESTS 1 00671 #endif 00672 00673 /** 00674 * SNMP_TRAP_DESTINATIONS: Number of trap destinations. At least one trap 00675 * destination is required 00676 */ 00677 #ifndef SNMP_TRAP_DESTINATIONS 00678 #define SNMP_TRAP_DESTINATIONS 1 00679 #endif 00680 00681 /** 00682 * SNMP_PRIVATE_MIB: 00683 * When using a private MIB, you have to create a file 'private_mib.h' that contains 00684 * a 'struct mib_array_node mib_private' which contains your MIB. 00685 */ 00686 #ifndef SNMP_PRIVATE_MIB 00687 #define SNMP_PRIVATE_MIB 0 00688 #endif 00689 00690 /** 00691 * Only allow SNMP write actions that are 'safe' (e.g. disabeling netifs is not 00692 * a safe action and disabled when SNMP_SAFE_REQUESTS = 1). 00693 * Unsafe requests are disabled by default! 00694 */ 00695 #ifndef SNMP_SAFE_REQUESTS 00696 #define SNMP_SAFE_REQUESTS 1 00697 #endif 00698 00699 /** 00700 * The maximum length of strings used. This affects the size of 00701 * MEMP_SNMP_VALUE elements. 00702 */ 00703 #ifndef SNMP_MAX_OCTET_STRING_LEN 00704 #define SNMP_MAX_OCTET_STRING_LEN 127 00705 #endif 00706 00707 /** 00708 * The maximum depth of the SNMP tree. 00709 * With private MIBs enabled, this depends on your MIB! 00710 * This affects the size of MEMP_SNMP_VALUE elements. 00711 */ 00712 #ifndef SNMP_MAX_TREE_DEPTH 00713 #define SNMP_MAX_TREE_DEPTH 15 00714 #endif 00715 00716 /** 00717 * The size of the MEMP_SNMP_VALUE elements, normally calculated from 00718 * SNMP_MAX_OCTET_STRING_LEN and SNMP_MAX_TREE_DEPTH. 00719 */ 00720 #ifndef SNMP_MAX_VALUE_SIZE 00721 #define SNMP_MAX_VALUE_SIZE LWIP_MAX((SNMP_MAX_OCTET_STRING_LEN)+1, sizeof(s32_t)*(SNMP_MAX_TREE_DEPTH)) 00722 #endif 00723 00724 /* 00725 ---------------------------------- 00726 ---------- IGMP options ---------- 00727 ---------------------------------- 00728 */ 00729 /** 00730 * LWIP_IGMP==1: Turn on IGMP module. 00731 */ 00732 #ifndef LWIP_IGMP 00733 #define LWIP_IGMP 0 00734 #endif 00735 00736 /* 00737 ---------------------------------- 00738 ---------- DNS options ----------- 00739 ---------------------------------- 00740 */ 00741 /** 00742 * LWIP_DNS==1: Turn on DNS module. UDP must be available for DNS 00743 * transport. 00744 */ 00745 #ifndef LWIP_DNS 00746 #define LWIP_DNS 0 00747 #endif 00748 00749 /** DNS maximum number of entries to maintain locally. */ 00750 #ifndef DNS_TABLE_SIZE 00751 #define DNS_TABLE_SIZE 4 00752 #endif 00753 00754 /** DNS maximum host name length supported in the name table. */ 00755 #ifndef DNS_MAX_NAME_LENGTH 00756 #define DNS_MAX_NAME_LENGTH 256 00757 #endif 00758 00759 /** The maximum of DNS servers */ 00760 #ifndef DNS_MAX_SERVERS 00761 #define DNS_MAX_SERVERS 2 00762 #endif 00763 00764 /** DNS do a name checking between the query and the response. */ 00765 #ifndef DNS_DOES_NAME_CHECK 00766 #define DNS_DOES_NAME_CHECK 1 00767 #endif 00768 00769 /** DNS message max. size. Default value is RFC compliant. */ 00770 #ifndef DNS_MSG_SIZE 00771 #define DNS_MSG_SIZE 512 00772 #endif 00773 00774 /** DNS_LOCAL_HOSTLIST: Implements a local host-to-address list. If enabled, 00775 * you have to define 00776 * #define DNS_LOCAL_HOSTLIST_INIT {{"host1", 0x123}, {"host2", 0x234}} 00777 * (an array of structs name/address, where address is an u32_t in network 00778 * byte order). 00779 * 00780 * Instead, you can also use an external function: 00781 * #define DNS_LOOKUP_LOCAL_EXTERN(x) extern u32_t my_lookup_function(const char *name) 00782 * that returns the IP address or INADDR_NONE if not found. 00783 */ 00784 #ifndef DNS_LOCAL_HOSTLIST 00785 #define DNS_LOCAL_HOSTLIST 0 00786 #endif /* DNS_LOCAL_HOSTLIST */ 00787 00788 /** If this is turned on, the local host-list can be dynamically changed 00789 * at runtime. */ 00790 #ifndef DNS_LOCAL_HOSTLIST_IS_DYNAMIC 00791 #define DNS_LOCAL_HOSTLIST_IS_DYNAMIC 0 00792 #endif /* DNS_LOCAL_HOSTLIST_IS_DYNAMIC */ 00793 00794 /* 00795 --------------------------------- 00796 ---------- UDP options ---------- 00797 --------------------------------- 00798 */ 00799 /** 00800 * LWIP_UDP==1: Turn on UDP. 00801 */ 00802 #ifndef LWIP_UDP 00803 #define LWIP_UDP 1 00804 #endif 00805 00806 /** 00807 * LWIP_UDPLITE==1: Turn on UDP-Lite. (Requires LWIP_UDP) 00808 */ 00809 #ifndef LWIP_UDPLITE 00810 #define LWIP_UDPLITE 0 00811 #endif 00812 00813 /** 00814 * UDP_TTL: Default Time-To-Live value. 00815 */ 00816 #ifndef UDP_TTL 00817 #define UDP_TTL (IP_DEFAULT_TTL) 00818 #endif 00819 00820 /** 00821 * LWIP_NETBUF_RECVINFO==1: append destination addr and port to every netbuf. 00822 */ 00823 #ifndef LWIP_NETBUF_RECVINFO 00824 #define LWIP_NETBUF_RECVINFO 0 00825 #endif 00826 00827 /* 00828 --------------------------------- 00829 ---------- TCP options ---------- 00830 --------------------------------- 00831 */ 00832 /** 00833 * LWIP_TCP==1: Turn on TCP. 00834 */ 00835 #ifndef LWIP_TCP 00836 #define LWIP_TCP 1 00837 #endif 00838 00839 /** 00840 * TCP_TTL: Default Time-To-Live value. 00841 */ 00842 #ifndef TCP_TTL 00843 #define TCP_TTL (IP_DEFAULT_TTL) 00844 #endif 00845 00846 /** 00847 * TCP_WND: The size of a TCP window. This must be at least 00848 * (2 * TCP_MSS) for things to work well 00849 */ 00850 #ifndef TCP_WND 00851 #define TCP_WND (4 * TCP_MSS) 00852 #endif 00853 00854 /** 00855 * TCP_MAXRTX: Maximum number of retransmissions of data segments. 00856 */ 00857 #ifndef TCP_MAXRTX 00858 #define TCP_MAXRTX 12 00859 #endif 00860 00861 /** 00862 * TCP_SYNMAXRTX: Maximum number of retransmissions of SYN segments. 00863 */ 00864 #ifndef TCP_SYNMAXRTX 00865 #define TCP_SYNMAXRTX 6 00866 #endif 00867 00868 /** 00869 * TCP_QUEUE_OOSEQ==1: TCP will queue segments that arrive out of order. 00870 * Define to 0 if your device is low on memory. 00871 */ 00872 #ifndef TCP_QUEUE_OOSEQ 00873 #define TCP_QUEUE_OOSEQ (LWIP_TCP) 00874 #endif 00875 00876 /** 00877 * TCP_MSS: TCP Maximum segment size. (default is 536, a conservative default, 00878 * you might want to increase this.) 00879 * For the receive side, this MSS is advertised to the remote side 00880 * when opening a connection. For the transmit size, this MSS sets 00881 * an upper limit on the MSS advertised by the remote host. 00882 */ 00883 #ifndef TCP_MSS 00884 #define TCP_MSS 536 00885 #endif 00886 00887 /** 00888 * TCP_CALCULATE_EFF_SEND_MSS: "The maximum size of a segment that TCP really 00889 * sends, the 'effective send MSS,' MUST be the smaller of the send MSS (which 00890 * reflects the available reassembly buffer size at the remote host) and the 00891 * largest size permitted by the IP layer" (RFC 1122) 00892 * Setting this to 1 enables code that checks TCP_MSS against the MTU of the 00893 * netif used for a connection and limits the MSS if it would be too big otherwise. 00894 */ 00895 #ifndef TCP_CALCULATE_EFF_SEND_MSS 00896 #define TCP_CALCULATE_EFF_SEND_MSS 1 00897 #endif 00898 00899 00900 /** 00901 * TCP_SND_BUF: TCP sender buffer space (bytes). 00902 */ 00903 #ifndef TCP_SND_BUF 00904 #define TCP_SND_BUF 256 00905 #endif 00906 00907 /** 00908 * TCP_SND_QUEUELEN: TCP sender buffer space (pbufs). This must be at least 00909 * as much as (2 * TCP_SND_BUF/TCP_MSS) for things to work. 00910 */ 00911 #ifndef TCP_SND_QUEUELEN 00912 #define TCP_SND_QUEUELEN (4 * (TCP_SND_BUF)/(TCP_MSS)) 00913 #endif 00914 00915 /** 00916 * TCP_SNDLOWAT: TCP writable space (bytes). This must be less than 00917 * TCP_SND_BUF. It is the amount of space which must be available in the 00918 * TCP snd_buf for select to return writable (combined with TCP_SNDQUEUELOWAT). 00919 */ 00920 #ifndef TCP_SNDLOWAT 00921 #define TCP_SNDLOWAT ((TCP_SND_BUF)/2) 00922 #endif 00923 00924 /** 00925 * TCP_SNDQUEUELOWAT: TCP writable bufs (pbuf count). This must be grater 00926 * than TCP_SND_QUEUELEN. If the number of pbufs queued on a pcb drops below 00927 * this number, select returns writable (combined with TCP_SNDLOWAT). 00928 */ 00929 #ifndef TCP_SNDQUEUELOWAT 00930 #define TCP_SNDQUEUELOWAT ((TCP_SND_QUEUELEN)/2) 00931 #endif 00932 00933 /** 00934 * TCP_LISTEN_BACKLOG: Enable the backlog option for tcp listen pcb. 00935 */ 00936 #ifndef TCP_LISTEN_BACKLOG 00937 #define TCP_LISTEN_BACKLOG 0 00938 #endif 00939 00940 /** 00941 * The maximum allowed backlog for TCP listen netconns. 00942 * This backlog is used unless another is explicitly specified. 00943 * 0xff is the maximum (u8_t). 00944 */ 00945 #ifndef TCP_DEFAULT_LISTEN_BACKLOG 00946 #define TCP_DEFAULT_LISTEN_BACKLOG 0xff 00947 #endif 00948 00949 /** 00950 * TCP_OVERSIZE: The maximum number of bytes that tcp_write may 00951 * allocate ahead of time in an attempt to create shorter pbuf chains 00952 * for transmission. The meaningful range is 0 to TCP_MSS. Some 00953 * suggested values are: 00954 * 00955 * 0: Disable oversized allocation. Each tcp_write() allocates a new 00956 pbuf (old behaviour). 00957 * 1: Allocate size-aligned pbufs with minimal excess. Use this if your 00958 * scatter-gather DMA requires aligned fragments. 00959 * 128: Limit the pbuf/memory overhead to 20%. 00960 * TCP_MSS: Try to create unfragmented TCP packets. 00961 * TCP_MSS/4: Try to create 4 fragments or less per TCP packet. 00962 */ 00963 #ifndef TCP_OVERSIZE 00964 #define TCP_OVERSIZE TCP_MSS 00965 #endif 00966 00967 /** 00968 * LWIP_TCP_TIMESTAMPS==1: support the TCP timestamp option. 00969 */ 00970 #ifndef LWIP_TCP_TIMESTAMPS 00971 #define LWIP_TCP_TIMESTAMPS 0 00972 #endif 00973 00974 /** 00975 * TCP_WND_UPDATE_THRESHOLD: difference in window to trigger an 00976 * explicit window update 00977 */ 00978 #ifndef TCP_WND_UPDATE_THRESHOLD 00979 #define TCP_WND_UPDATE_THRESHOLD (TCP_WND / 4) 00980 #endif 00981 00982 /** 00983 * LWIP_EVENT_API and LWIP_CALLBACK_API: Only one of these should be set to 1. 00984 * LWIP_EVENT_API==1: The user defines lwip_tcp_event() to receive all 00985 * events (accept, sent, etc) that happen in the system. 00986 * LWIP_CALLBACK_API==1: The PCB callback function is called directly 00987 * for the event. 00988 */ 00989 #ifndef LWIP_EVENT_API 00990 #define LWIP_EVENT_API 0 00991 #define LWIP_CALLBACK_API 1 00992 #else 00993 #define LWIP_EVENT_API 1 00994 #define LWIP_CALLBACK_API 0 00995 #endif 00996 00997 00998 /* 00999 ---------------------------------- 01000 ---------- Pbuf options ---------- 01001 ---------------------------------- 01002 */ 01003 /** 01004 * PBUF_LINK_HLEN: the number of bytes that should be allocated for a 01005 * link level header. The default is 14, the standard value for 01006 * Ethernet. 01007 */ 01008 #ifndef PBUF_LINK_HLEN 01009 #define PBUF_LINK_HLEN (14 + ETH_PAD_SIZE) 01010 #endif 01011 01012 /** 01013 * PBUF_POOL_BUFSIZE: the size of each pbuf in the pbuf pool. The default is 01014 * designed to accomodate single full size TCP frame in one pbuf, including 01015 * TCP_MSS, IP header, and link header. 01016 */ 01017 #ifndef PBUF_POOL_BUFSIZE 01018 #define PBUF_POOL_BUFSIZE LWIP_MEM_ALIGN_SIZE(TCP_MSS+40+PBUF_LINK_HLEN) 01019 #endif 01020 01021 /* 01022 ------------------------------------------------ 01023 ---------- Network Interfaces options ---------- 01024 ------------------------------------------------ 01025 */ 01026 /** 01027 * LWIP_NETIF_HOSTNAME==1: use DHCP_OPTION_HOSTNAME with netif's hostname 01028 * field. 01029 */ 01030 #ifndef LWIP_NETIF_HOSTNAME 01031 #define LWIP_NETIF_HOSTNAME 0 01032 #endif 01033 01034 /** 01035 * LWIP_NETIF_API==1: Support netif api (in netifapi.c) 01036 */ 01037 #ifndef LWIP_NETIF_API 01038 #define LWIP_NETIF_API 0 01039 #endif 01040 01041 /** 01042 * LWIP_NETIF_STATUS_CALLBACK==1: Support a callback function whenever an interface 01043 * changes its up/down status (i.e., due to DHCP IP acquistion) 01044 */ 01045 #ifndef LWIP_NETIF_STATUS_CALLBACK 01046 #define LWIP_NETIF_STATUS_CALLBACK 0 01047 #endif 01048 01049 /** 01050 * LWIP_NETIF_LINK_CALLBACK==1: Support a callback function from an interface 01051 * whenever the link changes (i.e., link down) 01052 */ 01053 #ifndef LWIP_NETIF_LINK_CALLBACK 01054 #define LWIP_NETIF_LINK_CALLBACK 0 01055 #endif 01056 01057 /** 01058 * LWIP_NETIF_HWADDRHINT==1: Cache link-layer-address hints (e.g. table 01059 * indices) in struct netif. TCP and UDP can make use of this to prevent 01060 * scanning the ARP table for every sent packet. While this is faster for big 01061 * ARP tables or many concurrent connections, it might be counterproductive 01062 * if you have a tiny ARP table or if there never are concurrent connections. 01063 */ 01064 #ifndef LWIP_NETIF_HWADDRHINT 01065 #define LWIP_NETIF_HWADDRHINT 0 01066 #endif 01067 01068 /** 01069 * LWIP_NETIF_LOOPBACK==1: Support sending packets with a destination IP 01070 * address equal to the netif IP address, looping them back up the stack. 01071 */ 01072 #ifndef LWIP_NETIF_LOOPBACK 01073 #define LWIP_NETIF_LOOPBACK 0 01074 #endif 01075 01076 /** 01077 * LWIP_LOOPBACK_MAX_PBUFS: Maximum number of pbufs on queue for loopback 01078 * sending for each netif (0 = disabled) 01079 */ 01080 #ifndef LWIP_LOOPBACK_MAX_PBUFS 01081 #define LWIP_LOOPBACK_MAX_PBUFS 0 01082 #endif 01083 01084 /** 01085 * LWIP_NETIF_LOOPBACK_MULTITHREADING: Indicates whether threading is enabled in 01086 * the system, as netifs must change how they behave depending on this setting 01087 * for the LWIP_NETIF_LOOPBACK option to work. 01088 * Setting this is needed to avoid reentering non-reentrant functions like 01089 * tcp_input(). 01090 * LWIP_NETIF_LOOPBACK_MULTITHREADING==1: Indicates that the user is using a 01091 * multithreaded environment like tcpip.c. In this case, netif->input() 01092 * is called directly. 01093 * LWIP_NETIF_LOOPBACK_MULTITHREADING==0: Indicates a polling (or NO_SYS) setup. 01094 * The packets are put on a list and netif_poll() must be called in 01095 * the main application loop. 01096 */ 01097 #ifndef LWIP_NETIF_LOOPBACK_MULTITHREADING 01098 #define LWIP_NETIF_LOOPBACK_MULTITHREADING (!NO_SYS) 01099 #endif 01100 01101 /** 01102 * LWIP_NETIF_TX_SINGLE_PBUF: if this is set to 1, lwIP tries to put all data 01103 * to be sent into one single pbuf. This is for compatibility with DMA-enabled 01104 * MACs that do not support scatter-gather. 01105 * Beware that this might involve CPU-memcpy before transmitting that would not 01106 * be needed without this flag! Use this only if you need to! 01107 * 01108 * @todo: TCP and IP-frag do not work with this, yet: 01109 */ 01110 #ifndef LWIP_NETIF_TX_SINGLE_PBUF 01111 #define LWIP_NETIF_TX_SINGLE_PBUF 0 01112 #endif /* LWIP_NETIF_TX_SINGLE_PBUF */ 01113 01114 /* 01115 ------------------------------------ 01116 ---------- LOOPIF options ---------- 01117 ------------------------------------ 01118 */ 01119 /** 01120 * LWIP_HAVE_LOOPIF==1: Support loop interface (127.0.0.1) and loopif.c 01121 */ 01122 #ifndef LWIP_HAVE_LOOPIF 01123 #define LWIP_HAVE_LOOPIF 0 01124 #endif 01125 01126 /* 01127 ------------------------------------ 01128 ---------- SLIPIF options ---------- 01129 ------------------------------------ 01130 */ 01131 /** 01132 * LWIP_HAVE_SLIPIF==1: Support slip interface and slipif.c 01133 */ 01134 #ifndef LWIP_HAVE_SLIPIF 01135 #define LWIP_HAVE_SLIPIF 0 01136 #endif 01137 01138 /* 01139 ------------------------------------ 01140 ---------- Thread options ---------- 01141 ------------------------------------ 01142 */ 01143 /** 01144 * TCPIP_THREAD_NAME: The name assigned to the main tcpip thread. 01145 */ 01146 #ifndef TCPIP_THREAD_NAME 01147 #define TCPIP_THREAD_NAME "tcpip_thread" 01148 #endif 01149 01150 /** 01151 * TCPIP_THREAD_STACKSIZE: The stack size used by the main tcpip thread. 01152 * The stack size value itself is platform-dependent, but is passed to 01153 * sys_thread_new() when the thread is created. 01154 */ 01155 #ifndef TCPIP_THREAD_STACKSIZE 01156 #define TCPIP_THREAD_STACKSIZE 0 01157 #endif 01158 01159 /** 01160 * TCPIP_THREAD_PRIO: The priority assigned to the main tcpip thread. 01161 * The priority value itself is platform-dependent, but is passed to 01162 * sys_thread_new() when the thread is created. 01163 */ 01164 #ifndef TCPIP_THREAD_PRIO 01165 #define TCPIP_THREAD_PRIO 1 01166 #endif 01167 01168 /** 01169 * TCPIP_MBOX_SIZE: The mailbox size for the tcpip thread messages 01170 * The queue size value itself is platform-dependent, but is passed to 01171 * sys_mbox_new() when tcpip_init is called. 01172 */ 01173 #ifndef TCPIP_MBOX_SIZE 01174 #define TCPIP_MBOX_SIZE 0 01175 #endif 01176 01177 /** 01178 * SLIPIF_THREAD_NAME: The name assigned to the slipif_loop thread. 01179 */ 01180 #ifndef SLIPIF_THREAD_NAME 01181 #define SLIPIF_THREAD_NAME "slipif_loop" 01182 #endif 01183 01184 /** 01185 * SLIP_THREAD_STACKSIZE: The stack size used by the slipif_loop thread. 01186 * The stack size value itself is platform-dependent, but is passed to 01187 * sys_thread_new() when the thread is created. 01188 */ 01189 #ifndef SLIPIF_THREAD_STACKSIZE 01190 #define SLIPIF_THREAD_STACKSIZE 0 01191 #endif 01192 01193 /** 01194 * SLIPIF_THREAD_PRIO: The priority assigned to the slipif_loop thread. 01195 * The priority value itself is platform-dependent, but is passed to 01196 * sys_thread_new() when the thread is created. 01197 */ 01198 #ifndef SLIPIF_THREAD_PRIO 01199 #define SLIPIF_THREAD_PRIO 1 01200 #endif 01201 01202 /** 01203 * PPP_THREAD_NAME: The name assigned to the pppInputThread. 01204 */ 01205 #ifndef PPP_THREAD_NAME 01206 #define PPP_THREAD_NAME "pppInputThread" 01207 #endif 01208 01209 /** 01210 * PPP_THREAD_STACKSIZE: The stack size used by the pppInputThread. 01211 * The stack size value itself is platform-dependent, but is passed to 01212 * sys_thread_new() when the thread is created. 01213 */ 01214 #ifndef PPP_THREAD_STACKSIZE 01215 #define PPP_THREAD_STACKSIZE 0 01216 #endif 01217 01218 /** 01219 * PPP_THREAD_PRIO: The priority assigned to the pppInputThread. 01220 * The priority value itself is platform-dependent, but is passed to 01221 * sys_thread_new() when the thread is created. 01222 */ 01223 #ifndef PPP_THREAD_PRIO 01224 #define PPP_THREAD_PRIO 1 01225 #endif 01226 01227 /** 01228 * DEFAULT_THREAD_NAME: The name assigned to any other lwIP thread. 01229 */ 01230 #ifndef DEFAULT_THREAD_NAME 01231 #define DEFAULT_THREAD_NAME "lwIP" 01232 #endif 01233 01234 /** 01235 * DEFAULT_THREAD_STACKSIZE: The stack size used by any other lwIP thread. 01236 * The stack size value itself is platform-dependent, but is passed to 01237 * sys_thread_new() when the thread is created. 01238 */ 01239 #ifndef DEFAULT_THREAD_STACKSIZE 01240 #define DEFAULT_THREAD_STACKSIZE 0 01241 #endif 01242 01243 /** 01244 * DEFAULT_THREAD_PRIO: The priority assigned to any other lwIP thread. 01245 * The priority value itself is platform-dependent, but is passed to 01246 * sys_thread_new() when the thread is created. 01247 */ 01248 #ifndef DEFAULT_THREAD_PRIO 01249 #define DEFAULT_THREAD_PRIO 1 01250 #endif 01251 01252 /** 01253 * DEFAULT_RAW_RECVMBOX_SIZE: The mailbox size for the incoming packets on a 01254 * NETCONN_RAW. The queue size value itself is platform-dependent, but is passed 01255 * to sys_mbox_new() when the recvmbox is created. 01256 */ 01257 #ifndef DEFAULT_RAW_RECVMBOX_SIZE 01258 #define DEFAULT_RAW_RECVMBOX_SIZE 0 01259 #endif 01260 01261 /** 01262 * DEFAULT_UDP_RECVMBOX_SIZE: The mailbox size for the incoming packets on a 01263 * NETCONN_UDP. The queue size value itself is platform-dependent, but is passed 01264 * to sys_mbox_new() when the recvmbox is created. 01265 */ 01266 #ifndef DEFAULT_UDP_RECVMBOX_SIZE 01267 #define DEFAULT_UDP_RECVMBOX_SIZE 0 01268 #endif 01269 01270 /** 01271 * DEFAULT_TCP_RECVMBOX_SIZE: The mailbox size for the incoming packets on a 01272 * NETCONN_TCP. The queue size value itself is platform-dependent, but is passed 01273 * to sys_mbox_new() when the recvmbox is created. 01274 */ 01275 #ifndef DEFAULT_TCP_RECVMBOX_SIZE 01276 #define DEFAULT_TCP_RECVMBOX_SIZE 0 01277 #endif 01278 01279 /** 01280 * DEFAULT_ACCEPTMBOX_SIZE: The mailbox size for the incoming connections. 01281 * The queue size value itself is platform-dependent, but is passed to 01282 * sys_mbox_new() when the acceptmbox is created. 01283 */ 01284 #ifndef DEFAULT_ACCEPTMBOX_SIZE 01285 #define DEFAULT_ACCEPTMBOX_SIZE 0 01286 #endif 01287 01288 /* 01289 ---------------------------------------------- 01290 ---------- Sequential layer options ---------- 01291 ---------------------------------------------- 01292 */ 01293 /** 01294 * LWIP_TCPIP_CORE_LOCKING: (EXPERIMENTAL!) 01295 * Don't use it if you're not an active lwIP project member 01296 */ 01297 #ifndef LWIP_TCPIP_CORE_LOCKING 01298 #define LWIP_TCPIP_CORE_LOCKING 0 01299 #endif 01300 01301 /** 01302 * LWIP_TCPIP_CORE_LOCKING_INPUT: (EXPERIMENTAL!) 01303 * Don't use it if you're not an active lwIP project member 01304 */ 01305 #ifndef LWIP_TCPIP_CORE_LOCKING_INPUT 01306 #define LWIP_TCPIP_CORE_LOCKING_INPUT 0 01307 #endif 01308 01309 /** 01310 * LWIP_NETCONN==1: Enable Netconn API (require to use api_lib.c) 01311 */ 01312 #ifndef LWIP_NETCONN 01313 #define LWIP_NETCONN 1 01314 #endif 01315 01316 /* 01317 ------------------------------------ 01318 ---------- Socket options ---------- 01319 ------------------------------------ 01320 */ 01321 /** 01322 * LWIP_SOCKET==1: Enable Socket API (require to use sockets.c) 01323 */ 01324 #ifndef LWIP_SOCKET 01325 #define LWIP_SOCKET 1 01326 #endif 01327 01328 /** 01329 * LWIP_COMPAT_SOCKETS==1: Enable BSD-style sockets functions names. 01330 * (only used if you use sockets.c) 01331 */ 01332 #ifndef LWIP_COMPAT_SOCKETS 01333 #define LWIP_COMPAT_SOCKETS 1 01334 #endif 01335 01336 /** 01337 * LWIP_POSIX_SOCKETS_IO_NAMES==1: Enable POSIX-style sockets functions names. 01338 * Disable this option if you use a POSIX operating system that uses the same 01339 * names (read, write & close). (only used if you use sockets.c) 01340 */ 01341 #ifndef LWIP_POSIX_SOCKETS_IO_NAMES 01342 #define LWIP_POSIX_SOCKETS_IO_NAMES 1 01343 #endif 01344 01345 /** 01346 * LWIP_TCP_KEEPALIVE==1: Enable TCP_KEEPIDLE, TCP_KEEPINTVL and TCP_KEEPCNT 01347 * options processing. Note that TCP_KEEPIDLE and TCP_KEEPINTVL have to be set 01348 * in seconds. (does not require sockets.c, and will affect tcp.c) 01349 */ 01350 #ifndef LWIP_TCP_KEEPALIVE 01351 #define LWIP_TCP_KEEPALIVE 0 01352 #endif 01353 01354 /** 01355 * LWIP_SO_RCVTIMEO==1: Enable SO_RCVTIMEO processing. 01356 */ 01357 #ifndef LWIP_SO_RCVTIMEO 01358 #define LWIP_SO_RCVTIMEO 0 01359 #endif 01360 01361 /** 01362 * LWIP_SO_RCVBUF==1: Enable SO_RCVBUF processing. 01363 */ 01364 #ifndef LWIP_SO_RCVBUF 01365 #define LWIP_SO_RCVBUF 0 01366 #endif 01367 01368 /** 01369 * If LWIP_SO_RCVBUF is used, this is the default value for recv_bufsize. 01370 */ 01371 #ifndef RECV_BUFSIZE_DEFAULT 01372 #define RECV_BUFSIZE_DEFAULT INT_MAX 01373 #endif 01374 01375 /** 01376 * SO_REUSE==1: Enable SO_REUSEADDR and SO_REUSEPORT options. DO NOT USE! 01377 */ 01378 #ifndef SO_REUSE 01379 #define SO_REUSE 0 01380 #endif 01381 01382 /* 01383 ---------------------------------------- 01384 ---------- Statistics options ---------- 01385 ---------------------------------------- 01386 */ 01387 /** 01388 * LWIP_STATS==1: Enable statistics collection in lwip_stats. 01389 */ 01390 #ifndef LWIP_STATS 01391 #define LWIP_STATS 1 01392 #endif 01393 01394 #if LWIP_STATS 01395 01396 /** 01397 * LWIP_STATS_DISPLAY==1: Compile in the statistics output functions. 01398 */ 01399 #ifndef LWIP_STATS_DISPLAY 01400 #define LWIP_STATS_DISPLAY 0 01401 #endif 01402 01403 /** 01404 * LINK_STATS==1: Enable link stats. 01405 */ 01406 #ifndef LINK_STATS 01407 #define LINK_STATS 1 01408 #endif 01409 01410 /** 01411 * ETHARP_STATS==1: Enable etharp stats. 01412 */ 01413 #ifndef ETHARP_STATS 01414 #define ETHARP_STATS (LWIP_ARP) 01415 #endif 01416 01417 /** 01418 * IP_STATS==1: Enable IP stats. 01419 */ 01420 #ifndef IP_STATS 01421 #define IP_STATS 1 01422 #endif 01423 01424 /** 01425 * IPFRAG_STATS==1: Enable IP fragmentation stats. Default is 01426 * on if using either frag or reass. 01427 */ 01428 #ifndef IPFRAG_STATS 01429 #define IPFRAG_STATS (IP_REASSEMBLY || IP_FRAG) 01430 #endif 01431 01432 /** 01433 * ICMP_STATS==1: Enable ICMP stats. 01434 */ 01435 #ifndef ICMP_STATS 01436 #define ICMP_STATS 1 01437 #endif 01438 01439 /** 01440 * IGMP_STATS==1: Enable IGMP stats. 01441 */ 01442 #ifndef IGMP_STATS 01443 #define IGMP_STATS (LWIP_IGMP) 01444 #endif 01445 01446 /** 01447 * UDP_STATS==1: Enable UDP stats. Default is on if 01448 * UDP enabled, otherwise off. 01449 */ 01450 #ifndef UDP_STATS 01451 #define UDP_STATS (LWIP_UDP) 01452 #endif 01453 01454 /** 01455 * TCP_STATS==1: Enable TCP stats. Default is on if TCP 01456 * enabled, otherwise off. 01457 */ 01458 #ifndef TCP_STATS 01459 #define TCP_STATS (LWIP_TCP) 01460 #endif 01461 01462 /** 01463 * MEM_STATS==1: Enable mem.c stats. 01464 */ 01465 #ifndef MEM_STATS 01466 #define MEM_STATS ((MEM_LIBC_MALLOC == 0) && (MEM_USE_POOLS == 0)) 01467 #endif 01468 01469 /** 01470 * MEMP_STATS==1: Enable memp.c pool stats. 01471 */ 01472 #ifndef MEMP_STATS 01473 #define MEMP_STATS (MEMP_MEM_MALLOC == 0) 01474 #endif 01475 01476 /** 01477 * SYS_STATS==1: Enable system stats (sem and mbox counts, etc). 01478 */ 01479 #ifndef SYS_STATS 01480 #define SYS_STATS (NO_SYS == 0) 01481 #endif 01482 01483 #else 01484 01485 #define LINK_STATS 0 01486 #define IP_STATS 0 01487 #define IPFRAG_STATS 0 01488 #define ICMP_STATS 0 01489 #define IGMP_STATS 0 01490 #define UDP_STATS 0 01491 #define TCP_STATS 0 01492 #define MEM_STATS 0 01493 #define MEMP_STATS 0 01494 #define SYS_STATS 0 01495 #define LWIP_STATS_DISPLAY 0 01496 01497 #endif /* LWIP_STATS */ 01498 01499 /* 01500 --------------------------------- 01501 ---------- PPP options ---------- 01502 --------------------------------- 01503 */ 01504 /** 01505 * PPP_SUPPORT==1: Enable PPP. 01506 */ 01507 #ifndef PPP_SUPPORT 01508 #define PPP_SUPPORT 0 01509 #endif 01510 01511 /** 01512 * PPPOE_SUPPORT==1: Enable PPP Over Ethernet 01513 */ 01514 #ifndef PPPOE_SUPPORT 01515 #define PPPOE_SUPPORT 0 01516 #endif 01517 01518 /** 01519 * PPPOS_SUPPORT==1: Enable PPP Over Serial 01520 */ 01521 #ifndef PPPOS_SUPPORT 01522 #define PPPOS_SUPPORT PPP_SUPPORT 01523 #endif 01524 01525 #if PPP_SUPPORT 01526 01527 /** 01528 * NUM_PPP: Max PPP sessions. 01529 */ 01530 #ifndef NUM_PPP 01531 #define NUM_PPP 1 01532 #endif 01533 01534 /** 01535 * PAP_SUPPORT==1: Support PAP. 01536 */ 01537 #ifndef PAP_SUPPORT 01538 #define PAP_SUPPORT 0 01539 #endif 01540 01541 /** 01542 * CHAP_SUPPORT==1: Support CHAP. 01543 */ 01544 #ifndef CHAP_SUPPORT 01545 #define CHAP_SUPPORT 0 01546 #endif 01547 01548 /** 01549 * MSCHAP_SUPPORT==1: Support MSCHAP. CURRENTLY NOT SUPPORTED! DO NOT SET! 01550 */ 01551 #ifndef MSCHAP_SUPPORT 01552 #define MSCHAP_SUPPORT 0 01553 #endif 01554 01555 /** 01556 * CBCP_SUPPORT==1: Support CBCP. CURRENTLY NOT SUPPORTED! DO NOT SET! 01557 */ 01558 #ifndef CBCP_SUPPORT 01559 #define CBCP_SUPPORT 0 01560 #endif 01561 01562 /** 01563 * CCP_SUPPORT==1: Support CCP. CURRENTLY NOT SUPPORTED! DO NOT SET! 01564 */ 01565 #ifndef CCP_SUPPORT 01566 #define CCP_SUPPORT 0 01567 #endif 01568 01569 /** 01570 * VJ_SUPPORT==1: Support VJ header compression. 01571 */ 01572 #ifndef VJ_SUPPORT 01573 #define VJ_SUPPORT 0 01574 #endif 01575 01576 /** 01577 * MD5_SUPPORT==1: Support MD5 (see also CHAP). 01578 */ 01579 #ifndef MD5_SUPPORT 01580 #define MD5_SUPPORT 0 01581 #endif 01582 01583 /* 01584 * Timeouts 01585 */ 01586 #ifndef FSM_DEFTIMEOUT 01587 #define FSM_DEFTIMEOUT 6 /* Timeout time in seconds */ 01588 #endif 01589 01590 #ifndef FSM_DEFMAXTERMREQS 01591 #define FSM_DEFMAXTERMREQS 2 /* Maximum Terminate-Request transmissions */ 01592 #endif 01593 01594 #ifndef FSM_DEFMAXCONFREQS 01595 #define FSM_DEFMAXCONFREQS 10 /* Maximum Configure-Request transmissions */ 01596 #endif 01597 01598 #ifndef FSM_DEFMAXNAKLOOPS 01599 #define FSM_DEFMAXNAKLOOPS 5 /* Maximum number of nak loops */ 01600 #endif 01601 01602 #ifndef UPAP_DEFTIMEOUT 01603 #define UPAP_DEFTIMEOUT 6 /* Timeout (seconds) for retransmitting req */ 01604 #endif 01605 01606 #ifndef UPAP_DEFREQTIME 01607 #define UPAP_DEFREQTIME 30 /* Time to wait for auth-req from peer */ 01608 #endif 01609 01610 #ifndef CHAP_DEFTIMEOUT 01611 #define CHAP_DEFTIMEOUT 6 /* Timeout time in seconds */ 01612 #endif 01613 01614 #ifndef CHAP_DEFTRANSMITS 01615 #define CHAP_DEFTRANSMITS 10 /* max # times to send challenge */ 01616 #endif 01617 01618 /* Interval in seconds between keepalive echo requests, 0 to disable. */ 01619 #ifndef LCP_ECHOINTERVAL 01620 #define LCP_ECHOINTERVAL 0 01621 #endif 01622 01623 /* Number of unanswered echo requests before failure. */ 01624 #ifndef LCP_MAXECHOFAILS 01625 #define LCP_MAXECHOFAILS 3 01626 #endif 01627 01628 /* Max Xmit idle time (in jiffies) before resend flag char. */ 01629 #ifndef PPP_MAXIDLEFLAG 01630 #define PPP_MAXIDLEFLAG 100 01631 #endif 01632 01633 /* 01634 * Packet sizes 01635 * 01636 * Note - lcp shouldn't be allowed to negotiate stuff outside these 01637 * limits. See lcp.h in the pppd directory. 01638 * (XXX - these constants should simply be shared by lcp.c instead 01639 * of living in lcp.h) 01640 */ 01641 #define PPP_MTU 1500 /* Default MTU (size of Info field) */ 01642 #ifndef PPP_MAXMTU 01643 /* #define PPP_MAXMTU 65535 - (PPP_HDRLEN + PPP_FCSLEN) */ 01644 #define PPP_MAXMTU 1500 /* Largest MTU we allow */ 01645 #endif 01646 #define PPP_MINMTU 64 01647 #define PPP_MRU 1500 /* default MRU = max length of info field */ 01648 #define PPP_MAXMRU 1500 /* Largest MRU we allow */ 01649 #ifndef PPP_DEFMRU 01650 #define PPP_DEFMRU 296 /* Try for this */ 01651 #endif 01652 #define PPP_MINMRU 128 /* No MRUs below this */ 01653 01654 #ifndef MAXNAMELEN 01655 #define MAXNAMELEN 256 /* max length of hostname or name for auth */ 01656 #endif 01657 #ifndef MAXSECRETLEN 01658 #define MAXSECRETLEN 256 /* max length of password or secret */ 01659 #endif 01660 01661 #endif /* PPP_SUPPORT */ 01662 01663 /* 01664 -------------------------------------- 01665 ---------- Checksum options ---------- 01666 -------------------------------------- 01667 */ 01668 /** 01669 * CHECKSUM_GEN_IP==1: Generate checksums in software for outgoing IP packets. 01670 */ 01671 #ifndef CHECKSUM_GEN_IP 01672 #define CHECKSUM_GEN_IP 1 01673 #endif 01674 01675 /** 01676 * CHECKSUM_GEN_UDP==1: Generate checksums in software for outgoing UDP packets. 01677 */ 01678 #ifndef CHECKSUM_GEN_UDP 01679 #define CHECKSUM_GEN_UDP 1 01680 #endif 01681 01682 /** 01683 * CHECKSUM_GEN_TCP==1: Generate checksums in software for outgoing TCP packets. 01684 */ 01685 #ifndef CHECKSUM_GEN_TCP 01686 #define CHECKSUM_GEN_TCP 1 01687 #endif 01688 01689 /** 01690 * CHECKSUM_CHECK_IP==1: Check checksums in software for incoming IP packets. 01691 */ 01692 #ifndef CHECKSUM_CHECK_IP 01693 #define CHECKSUM_CHECK_IP 1 01694 #endif 01695 01696 /** 01697 * CHECKSUM_CHECK_UDP==1: Check checksums in software for incoming UDP packets. 01698 */ 01699 #ifndef CHECKSUM_CHECK_UDP 01700 #define CHECKSUM_CHECK_UDP 1 01701 #endif 01702 01703 /** 01704 * CHECKSUM_CHECK_TCP==1: Check checksums in software for incoming TCP packets. 01705 */ 01706 #ifndef CHECKSUM_CHECK_TCP 01707 #define CHECKSUM_CHECK_TCP 1 01708 #endif 01709 01710 /** 01711 * LWIP_CHECKSUM_ON_COPY==1: Calculate checksum when copying data from 01712 * application buffers to pbufs. 01713 */ 01714 #ifndef LWIP_CHECKSUM_ON_COPY 01715 #define LWIP_CHECKSUM_ON_COPY 0 01716 #endif 01717 01718 /* 01719 --------------------------------------- 01720 ---------- Debugging options ---------- 01721 --------------------------------------- 01722 */ 01723 /** 01724 * LWIP_DBG_MIN_LEVEL: After masking, the value of the debug is 01725 * compared against this value. If it is smaller, then debugging 01726 * messages are written. 01727 */ 01728 #ifndef LWIP_DBG_MIN_LEVEL 01729 #define LWIP_DBG_MIN_LEVEL LWIP_DBG_LEVEL_ALL 01730 #endif 01731 01732 /** 01733 * LWIP_DBG_TYPES_ON: A mask that can be used to globally enable/disable 01734 * debug messages of certain types. 01735 */ 01736 #ifndef LWIP_DBG_TYPES_ON 01737 #define LWIP_DBG_TYPES_ON LWIP_DBG_ON 01738 #endif 01739 01740 /** 01741 * ETHARP_DEBUG: Enable debugging in etharp.c. 01742 */ 01743 #ifndef ETHARP_DEBUG 01744 #define ETHARP_DEBUG LWIP_DBG_OFF 01745 #endif 01746 01747 /** 01748 * NETIF_DEBUG: Enable debugging in netif.c. 01749 */ 01750 #ifndef NETIF_DEBUG 01751 #define NETIF_DEBUG LWIP_DBG_OFF 01752 #endif 01753 01754 /** 01755 * PBUF_DEBUG: Enable debugging in pbuf.c. 01756 */ 01757 #ifndef PBUF_DEBUG 01758 #define PBUF_DEBUG LWIP_DBG_OFF 01759 #endif 01760 01761 /** 01762 * API_LIB_DEBUG: Enable debugging in api_lib.c. 01763 */ 01764 #ifndef API_LIB_DEBUG 01765 #define API_LIB_DEBUG LWIP_DBG_OFF 01766 #endif 01767 01768 /** 01769 * API_MSG_DEBUG: Enable debugging in api_msg.c. 01770 */ 01771 #ifndef API_MSG_DEBUG 01772 #define API_MSG_DEBUG LWIP_DBG_OFF 01773 #endif 01774 01775 /** 01776 * SOCKETS_DEBUG: Enable debugging in sockets.c. 01777 */ 01778 #ifndef SOCKETS_DEBUG 01779 #define SOCKETS_DEBUG LWIP_DBG_OFF 01780 #endif 01781 01782 /** 01783 * ICMP_DEBUG: Enable debugging in icmp.c. 01784 */ 01785 #ifndef ICMP_DEBUG 01786 #define ICMP_DEBUG LWIP_DBG_OFF 01787 #endif 01788 01789 /** 01790 * IGMP_DEBUG: Enable debugging in igmp.c. 01791 */ 01792 #ifndef IGMP_DEBUG 01793 #define IGMP_DEBUG LWIP_DBG_OFF 01794 #endif 01795 01796 /** 01797 * INET_DEBUG: Enable debugging in inet.c. 01798 */ 01799 #ifndef INET_DEBUG 01800 #define INET_DEBUG LWIP_DBG_OFF 01801 #endif 01802 01803 /** 01804 * IP_DEBUG: Enable debugging for IP. 01805 */ 01806 #ifndef IP_DEBUG 01807 #define IP_DEBUG LWIP_DBG_OFF 01808 #endif 01809 01810 /** 01811 * IP_REASS_DEBUG: Enable debugging in ip_frag.c for both frag & reass. 01812 */ 01813 #ifndef IP_REASS_DEBUG 01814 #define IP_REASS_DEBUG LWIP_DBG_OFF 01815 #endif 01816 01817 /** 01818 * RAW_DEBUG: Enable debugging in raw.c. 01819 */ 01820 #ifndef RAW_DEBUG 01821 #define RAW_DEBUG LWIP_DBG_OFF 01822 #endif 01823 01824 /** 01825 * MEM_DEBUG: Enable debugging in mem.c. 01826 */ 01827 #ifndef MEM_DEBUG 01828 #define MEM_DEBUG LWIP_DBG_OFF 01829 #endif 01830 01831 /** 01832 * MEMP_DEBUG: Enable debugging in memp.c. 01833 */ 01834 #ifndef MEMP_DEBUG 01835 #define MEMP_DEBUG LWIP_DBG_OFF 01836 #endif 01837 01838 /** 01839 * SYS_DEBUG: Enable debugging in sys.c. 01840 */ 01841 #ifndef SYS_DEBUG 01842 #define SYS_DEBUG LWIP_DBG_OFF 01843 #endif 01844 01845 /** 01846 * TIMERS_DEBUG: Enable debugging in timers.c. 01847 */ 01848 #ifndef TIMERS_DEBUG 01849 #define TIMERS_DEBUG LWIP_DBG_OFF 01850 #endif 01851 01852 /** 01853 * TCP_DEBUG: Enable debugging for TCP. 01854 */ 01855 #ifndef TCP_DEBUG 01856 #define TCP_DEBUG LWIP_DBG_OFF 01857 #endif 01858 01859 /** 01860 * TCP_INPUT_DEBUG: Enable debugging in tcp_in.c for incoming debug. 01861 */ 01862 #ifndef TCP_INPUT_DEBUG 01863 #define TCP_INPUT_DEBUG LWIP_DBG_OFF 01864 #endif 01865 01866 /** 01867 * TCP_FR_DEBUG: Enable debugging in tcp_in.c for fast retransmit. 01868 */ 01869 #ifndef TCP_FR_DEBUG 01870 #define TCP_FR_DEBUG LWIP_DBG_OFF 01871 #endif 01872 01873 /** 01874 * TCP_RTO_DEBUG: Enable debugging in TCP for retransmit 01875 * timeout. 01876 */ 01877 #ifndef TCP_RTO_DEBUG 01878 #define TCP_RTO_DEBUG LWIP_DBG_OFF 01879 #endif 01880 01881 /** 01882 * TCP_CWND_DEBUG: Enable debugging for TCP congestion window. 01883 */ 01884 #ifndef TCP_CWND_DEBUG 01885 #define TCP_CWND_DEBUG LWIP_DBG_OFF 01886 #endif 01887 01888 /** 01889 * TCP_WND_DEBUG: Enable debugging in tcp_in.c for window updating. 01890 */ 01891 #ifndef TCP_WND_DEBUG 01892 #define TCP_WND_DEBUG LWIP_DBG_OFF 01893 #endif 01894 01895 /** 01896 * TCP_OUTPUT_DEBUG: Enable debugging in tcp_out.c output functions. 01897 */ 01898 #ifndef TCP_OUTPUT_DEBUG 01899 #define TCP_OUTPUT_DEBUG LWIP_DBG_OFF 01900 #endif 01901 01902 /** 01903 * TCP_RST_DEBUG: Enable debugging for TCP with the RST message. 01904 */ 01905 #ifndef TCP_RST_DEBUG 01906 #define TCP_RST_DEBUG LWIP_DBG_OFF 01907 #endif 01908 01909 /** 01910 * TCP_QLEN_DEBUG: Enable debugging for TCP queue lengths. 01911 */ 01912 #ifndef TCP_QLEN_DEBUG 01913 #define TCP_QLEN_DEBUG LWIP_DBG_OFF 01914 #endif 01915 01916 /** 01917 * UDP_DEBUG: Enable debugging in UDP. 01918 */ 01919 #ifndef UDP_DEBUG 01920 #define UDP_DEBUG LWIP_DBG_OFF 01921 #endif 01922 01923 /** 01924 * TCPIP_DEBUG: Enable debugging in tcpip.c. 01925 */ 01926 #ifndef TCPIP_DEBUG 01927 #define TCPIP_DEBUG LWIP_DBG_OFF 01928 #endif 01929 01930 /** 01931 * PPP_DEBUG: Enable debugging for PPP. 01932 */ 01933 #ifndef PPP_DEBUG 01934 #define PPP_DEBUG LWIP_DBG_OFF 01935 #endif 01936 01937 /** 01938 * SLIP_DEBUG: Enable debugging in slipif.c. 01939 */ 01940 #ifndef SLIP_DEBUG 01941 #define SLIP_DEBUG LWIP_DBG_OFF 01942 #endif 01943 01944 /** 01945 * DHCP_DEBUG: Enable debugging in dhcp.c. 01946 */ 01947 #ifndef DHCP_DEBUG 01948 #define DHCP_DEBUG LWIP_DBG_OFF 01949 #endif 01950 01951 /** 01952 * AUTOIP_DEBUG: Enable debugging in autoip.c. 01953 */ 01954 #ifndef AUTOIP_DEBUG 01955 #define AUTOIP_DEBUG LWIP_DBG_OFF 01956 #endif 01957 01958 /** 01959 * SNMP_MSG_DEBUG: Enable debugging for SNMP messages. 01960 */ 01961 #ifndef SNMP_MSG_DEBUG 01962 #define SNMP_MSG_DEBUG LWIP_DBG_OFF 01963 #endif 01964 01965 /** 01966 * SNMP_MIB_DEBUG: Enable debugging for SNMP MIBs. 01967 */ 01968 #ifndef SNMP_MIB_DEBUG 01969 #define SNMP_MIB_DEBUG LWIP_DBG_OFF 01970 #endif 01971 01972 /** 01973 * DNS_DEBUG: Enable debugging for DNS. 01974 */ 01975 #ifndef DNS_DEBUG 01976 #define DNS_DEBUG LWIP_DBG_OFF 01977 #endif 01978 01979 #endif /* __LWIP_OPT_H__ */
Generated on Tue Jul 12 2022 21:10:26 by
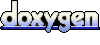