
Example program with HTTPServer and sensor data streaming over TCPSockets, using Donatien Garnier's Net APIs and services code on top of LWIP. Files StreamServer.h and .cpp encapsulate streaming over TCPSockets. Broadcast is done by sendToAll(), and all incoming data is echoed back to the client. Echo code can be replaced with some remote control of the streaming interface. See main() that shows how to periodically send some data to all subscribed clients. To subscribe, a client should open a socket at <mbed_ip> port 123. I used few lines in TCL code to set up a quick sink for the data. HTTP files are served on port 80 concurrently to the streaming.
icmp.h
00001 /* 00002 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 * 00029 * Author: Adam Dunkels <adam@sics.se> 00030 * 00031 */ 00032 #ifndef __LWIP_ICMP_H__ 00033 #define __LWIP_ICMP_H__ 00034 00035 #include "lwip/opt.h" 00036 #include "lwip/pbuf.h" 00037 #include "lwip/ip_addr.h" 00038 #include "lwip/netif.h" 00039 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif 00043 00044 #define ICMP_ER 0 /* echo reply */ 00045 #define ICMP_DUR 3 /* destination unreachable */ 00046 #define ICMP_SQ 4 /* source quench */ 00047 #define ICMP_RD 5 /* redirect */ 00048 #define ICMP_ECHO 8 /* echo */ 00049 #define ICMP_TE 11 /* time exceeded */ 00050 #define ICMP_PP 12 /* parameter problem */ 00051 #define ICMP_TS 13 /* timestamp */ 00052 #define ICMP_TSR 14 /* timestamp reply */ 00053 #define ICMP_IRQ 15 /* information request */ 00054 #define ICMP_IR 16 /* information reply */ 00055 00056 enum icmp_dur_type { 00057 ICMP_DUR_NET = 0, /* net unreachable */ 00058 ICMP_DUR_HOST = 1, /* host unreachable */ 00059 ICMP_DUR_PROTO = 2, /* protocol unreachable */ 00060 ICMP_DUR_PORT = 3, /* port unreachable */ 00061 ICMP_DUR_FRAG = 4, /* fragmentation needed and DF set */ 00062 ICMP_DUR_SR = 5 /* source route failed */ 00063 }; 00064 00065 enum icmp_te_type { 00066 ICMP_TE_TTL = 0, /* time to live exceeded in transit */ 00067 ICMP_TE_FRAG = 1 /* fragment reassembly time exceeded */ 00068 }; 00069 00070 #ifdef PACK_STRUCT_USE_INCLUDES 00071 # include "arch/bpstruct.h" 00072 #endif 00073 /** This is the standard ICMP header only that the u32_t data 00074 * is splitted to two u16_t like ICMP echo needs it. 00075 * This header is also used for other ICMP types that do not 00076 * use the data part. 00077 */ 00078 PACK_STRUCT_BEGIN 00079 struct icmp_echo_hdr { 00080 PACK_STRUCT_FIELD(u8_t type); 00081 PACK_STRUCT_FIELD(u8_t code); 00082 PACK_STRUCT_FIELD(u16_t chksum); 00083 PACK_STRUCT_FIELD(u16_t id); 00084 PACK_STRUCT_FIELD(u16_t seqno); 00085 } PACK_STRUCT_STRUCT; 00086 PACK_STRUCT_END 00087 #ifdef PACK_STRUCT_USE_INCLUDES 00088 # include "arch/epstruct.h" 00089 #endif 00090 00091 #define ICMPH_TYPE(hdr) ((hdr)->type) 00092 #define ICMPH_CODE(hdr) ((hdr)->code) 00093 00094 /** Combines type and code to an u16_t */ 00095 #define ICMPH_TYPE_SET(hdr, t) ((hdr)->type = (t)) 00096 #define ICMPH_CODE_SET(hdr, c) ((hdr)->code = (c)) 00097 00098 00099 #if LWIP_ICMP /* don't build if not configured for use in lwipopts.h */ 00100 00101 void icmp_input(struct pbuf *p, struct netif *inp); 00102 void icmp_dest_unreach(struct pbuf *p, enum icmp_dur_type t); 00103 void icmp_time_exceeded(struct pbuf *p, enum icmp_te_type t); 00104 00105 #endif /* LWIP_ICMP */ 00106 00107 #ifdef __cplusplus 00108 } 00109 #endif 00110 00111 #endif /* __LWIP_ICMP_H__ */
Generated on Tue Jul 12 2022 21:10:25 by
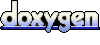