
Example program with HTTPServer and sensor data streaming over TCPSockets, using Donatien Garnier's Net APIs and services code on top of LWIP. Files StreamServer.h and .cpp encapsulate streaming over TCPSockets. Broadcast is done by sendToAll(), and all incoming data is echoed back to the client. Echo code can be replaced with some remote control of the streaming interface. See main() that shows how to periodically send some data to all subscribed clients. To subscribe, a client should open a socket at <mbed_ip> port 123. I used few lines in TCL code to set up a quick sink for the data. HTTP files are served on port 80 concurrently to the streaming.
etharp.h
00001 /* 00002 * Copyright (c) 2001-2003 Swedish Institute of Computer Science. 00003 * Copyright (c) 2003-2004 Leon Woestenberg <leon.woestenberg@axon.tv> 00004 * Copyright (c) 2003-2004 Axon Digital Design B.V., The Netherlands. 00005 * All rights reserved. 00006 * 00007 * Redistribution and use in source and binary forms, with or without modification, 00008 * are permitted provided that the following conditions are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright notice, 00011 * this list of conditions and the following disclaimer. 00012 * 2. Redistributions in binary form must reproduce the above copyright notice, 00013 * this list of conditions and the following disclaimer in the documentation 00014 * and/or other materials provided with the distribution. 00015 * 3. The name of the author may not be used to endorse or promote products 00016 * derived from this software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00019 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00020 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00021 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00022 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00023 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00024 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00025 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00026 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00027 * OF SUCH DAMAGE. 00028 * 00029 * This file is part of the lwIP TCP/IP stack. 00030 * 00031 * Author: Adam Dunkels <adam@sics.se> 00032 * 00033 */ 00034 00035 #ifndef __NETIF_ETHARP_H__ 00036 #define __NETIF_ETHARP_H__ 00037 00038 #include "lwip/opt.h" 00039 00040 #if LWIP_ARP || LWIP_ETHERNET /* don't build if not configured for use in lwipopts.h */ 00041 00042 #include "lwip/pbuf.h" 00043 #include "lwip/ip_addr.h" 00044 #include "lwip/netif.h" 00045 #include "lwip/ip.h" 00046 00047 #ifdef __cplusplus 00048 extern "C" { 00049 #endif 00050 00051 #ifndef ETHARP_HWADDR_LEN 00052 #define ETHARP_HWADDR_LEN 6 00053 #endif 00054 00055 #ifdef PACK_STRUCT_USE_INCLUDES 00056 # include "arch/bpstruct.h" 00057 #endif 00058 PACK_STRUCT_BEGIN 00059 struct eth_addr { 00060 PACK_STRUCT_FIELD(u8_t addr[ETHARP_HWADDR_LEN]); 00061 } PACK_STRUCT_STRUCT; 00062 PACK_STRUCT_END 00063 #ifdef PACK_STRUCT_USE_INCLUDES 00064 # include "arch/epstruct.h" 00065 #endif 00066 00067 #ifdef PACK_STRUCT_USE_INCLUDES 00068 # include "arch/bpstruct.h" 00069 #endif 00070 PACK_STRUCT_BEGIN 00071 /** Ethernet header */ 00072 struct eth_hdr { 00073 #if ETH_PAD_SIZE 00074 PACK_STRUCT_FIELD(u8_t padding[ETH_PAD_SIZE]); 00075 #endif 00076 PACK_STRUCT_FIELD(struct eth_addr dest); 00077 PACK_STRUCT_FIELD(struct eth_addr src); 00078 PACK_STRUCT_FIELD(u16_t type); 00079 } PACK_STRUCT_STRUCT; 00080 PACK_STRUCT_END 00081 #ifdef PACK_STRUCT_USE_INCLUDES 00082 # include "arch/epstruct.h" 00083 #endif 00084 00085 #define SIZEOF_ETH_HDR (14 + ETH_PAD_SIZE) 00086 00087 #if ETHARP_SUPPORT_VLAN 00088 00089 #ifdef PACK_STRUCT_USE_INCLUDES 00090 # include "arch/bpstruct.h" 00091 #endif 00092 PACK_STRUCT_BEGIN 00093 /** VLAN header inserted between ethernet header and payload 00094 * if 'type' in ethernet header is ETHTYPE_VLAN. 00095 * See IEEE802.Q */ 00096 struct eth_vlan_hdr { 00097 PACK_STRUCT_FIELD(u16_t tpid); 00098 PACK_STRUCT_FIELD(u16_t prio_vid); 00099 } PACK_STRUCT_STRUCT; 00100 PACK_STRUCT_END 00101 #ifdef PACK_STRUCT_USE_INCLUDES 00102 # include "arch/epstruct.h" 00103 #endif 00104 00105 #define SIZEOF_VLAN_HDR 4 00106 #define VLAN_ID(vlan_hdr) (htons((vlan_hdr)->prio_vid) & 0xFFF) 00107 00108 #endif /* ETHARP_SUPPORT_VLAN */ 00109 00110 #ifdef PACK_STRUCT_USE_INCLUDES 00111 # include "arch/bpstruct.h" 00112 #endif 00113 PACK_STRUCT_BEGIN 00114 /** the ARP message, see RFC 826 ("Packet format") */ 00115 struct etharp_hdr { 00116 PACK_STRUCT_FIELD(u16_t hwtype); 00117 PACK_STRUCT_FIELD(u16_t proto); 00118 PACK_STRUCT_FIELD(u16_t _hwlen_protolen); 00119 PACK_STRUCT_FIELD(u16_t opcode); 00120 PACK_STRUCT_FIELD(struct eth_addr shwaddr); 00121 PACK_STRUCT_FIELD(struct ip_addr2 sipaddr); 00122 PACK_STRUCT_FIELD(struct eth_addr dhwaddr); 00123 PACK_STRUCT_FIELD(struct ip_addr2 dipaddr); 00124 } PACK_STRUCT_STRUCT; 00125 PACK_STRUCT_END 00126 #ifdef PACK_STRUCT_USE_INCLUDES 00127 # include "arch/epstruct.h" 00128 #endif 00129 00130 #define SIZEOF_ETHARP_HDR 28 00131 #define SIZEOF_ETHARP_PACKET (SIZEOF_ETH_HDR + SIZEOF_ETHARP_HDR) 00132 00133 /** 5 seconds period */ 00134 #define ARP_TMR_INTERVAL 5000 00135 00136 #define ETHTYPE_ARP 0x0806 00137 #define ETHTYPE_IP 0x0800 00138 #define ETHTYPE_VLAN 0x8100 00139 #define ETHTYPE_PPPOEDISC 0x8863 /* PPP Over Ethernet Discovery Stage */ 00140 #define ETHTYPE_PPPOE 0x8864 /* PPP Over Ethernet Session Stage */ 00141 00142 #if LWIP_ARP /* don't build if not configured for use in lwipopts.h */ 00143 00144 /** ARP message types (opcodes) */ 00145 #define ARP_REQUEST 1 00146 #define ARP_REPLY 2 00147 00148 #if ARP_QUEUEING 00149 /** struct for queueing outgoing packets for unknown address 00150 * defined here to be accessed by memp.h 00151 */ 00152 struct etharp_q_entry { 00153 struct etharp_q_entry *next; 00154 struct pbuf *p; 00155 }; 00156 #endif /* ARP_QUEUEING */ 00157 00158 #define etharp_init() /* Compatibility define, not init needed. */ 00159 void etharp_tmr(void); 00160 s8_t etharp_find_addr(struct netif *netif, ip_addr_t *ipaddr, 00161 struct eth_addr **eth_ret, ip_addr_t **ip_ret); 00162 err_t etharp_output(struct netif *netif, struct pbuf *q, ip_addr_t *ipaddr); 00163 err_t etharp_query(struct netif *netif, ip_addr_t *ipaddr, struct pbuf *q); 00164 err_t etharp_request(struct netif *netif, ip_addr_t *ipaddr); 00165 /** For Ethernet network interfaces, we might want to send "gratuitous ARP"; 00166 * this is an ARP packet sent by a node in order to spontaneously cause other 00167 * nodes to update an entry in their ARP cache. 00168 * From RFC 3220 "IP Mobility Support for IPv4" section 4.6. */ 00169 #define etharp_gratuitous(netif) etharp_request((netif), &(netif)->ip_addr) 00170 00171 #if LWIP_AUTOIP 00172 err_t etharp_raw(struct netif *netif, const struct eth_addr *ethsrc_addr, 00173 const struct eth_addr *ethdst_addr, 00174 const struct eth_addr *hwsrc_addr, const ip_addr_t *ipsrc_addr, 00175 const struct eth_addr *hwdst_addr, const ip_addr_t *ipdst_addr, 00176 const u16_t opcode); 00177 #endif /* LWIP_AUTOIP */ 00178 00179 00180 #endif /* LWIP_ARP */ 00181 00182 err_t ethernet_input(struct pbuf *p, struct netif *netif); 00183 00184 #define eth_addr_cmp(addr1, addr2) (memcmp((addr1)->addr, (addr2)->addr, ETHARP_HWADDR_LEN) == 0) 00185 00186 extern const struct eth_addr ethbroadcast, ethzero; 00187 00188 #ifdef __cplusplus 00189 } 00190 #endif 00191 00192 #endif /* LWIP_ARP || LWIP_ETHERNET */ 00193 00194 #endif /* __NETIF_ARP_H__ */
Generated on Tue Jul 12 2022 21:10:25 by
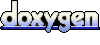