
student project
Embed:
(wiki syntax)
Show/hide line numbers
I2C_SSD1306Z.cpp
00001 // 00002 // SSD1306Z LCD Driver: 0.96" lcd LY096BG30 00003 // 00004 // Interface: I2C 00005 // pin1: Vcc 00006 // pin2: Gnd 00007 // pin3: SCL 00008 // pin4: SDA 00009 00010 #include "mbed.h" 00011 #include "I2C_SSD1306Z.h" 00012 #include "Font8x16.h" 00013 #include "Font5x7.h" 00014 00015 00016 //I2C i2c(I2C_SDA, I2C_SCL); 00017 extern I2C i2c; 00018 00019 char DisplayBuffer[128*8]; 00020 00021 #if 1 00022 00023 void lcdWriteCommand(uint8_t lcd_Command) 00024 { 00025 00026 char data[2]; 00027 data[0]=0x0; 00028 data[1]=lcd_Command; 00029 // I2C_writeBytes(LCD_I2C_PORT, LCD_I2C_SLA, 0x00, 1, data); 00030 // i2c_write_data_block(LCD_I2C_SLA, 0x00, data,1); 00031 i2c.write(LCD_I2C_SLA, data, 2, 0); 00032 00033 } 00034 00035 void lcdWriteData(uint8_t lcd_Data) 00036 { 00037 00038 char data[2]; 00039 data[0]=0x40; 00040 data[1]=lcd_Data; 00041 // I2C_writeBytes(LCD_I2C_PORT, LCD_I2C_SLA, 0x40, 1, data); 00042 //i2c_write_data_block(LCD_I2C_SLA, 0x40,data,1); 00043 i2c.write(LCD_I2C_SLA, data, 2, 0); 00044 } 00045 00046 #else 00047 00048 void lcdWriteCommand(uint8_t lcd_Command) 00049 { 00050 uint8_t data[1]; 00051 data[0]=lcd_Command; 00052 // I2C_writeBytes(LCD_I2C_PORT, LCD_I2C_SLA, 0x00, 1, data); 00053 i2c_write_data_block(LCD_I2C_SLA, 0x00, data,1); 00054 00055 } 00056 00057 void lcdWriteData(uint8_t lcd_Data) 00058 { 00059 uint8_t data[1]; 00060 data[0]=lcd_Data; 00061 // I2C_writeBytes(LCD_I2C_PORT, LCD_I2C_SLA, 0x40, 1, data); 00062 i2c_write_data_block(LCD_I2C_SLA, 0x40,data,1); 00063 } 00064 00065 #endif 00066 00067 void lcdSetAddr(uint8_t column, uint8_t page) 00068 { 00069 lcdWriteCommand(0xb0+page); // set page address 00070 lcdWriteCommand(0x10 | ((column & 0xf0) >> 4)); // set column address MSB 00071 lcdWriteCommand(0x00 | (column & 0x0f) ); // set column address LSB 00072 } 00073 00074 void Init_LCD(void) 00075 { 00076 lcdWriteCommand(0xae); //display off 00077 lcdWriteCommand(0x20); //Set Memory Addressing Mode 00078 lcdWriteCommand(0x10); //00,Horizontal Addressing Mode;01,Vertical Addressing Mode;10,Page Addressing Mode (RESET);11,Invalid 00079 lcdWriteCommand(0xb0); //Set Page Start Address for Page Addressing Mode,0-7 00080 lcdWriteCommand(0xc8); //Set COM Output Scan Direction 00081 lcdWriteCommand(0x00);//---set low column address 00082 lcdWriteCommand(0x10);//---set high column address 00083 lcdWriteCommand(0x40);//--set start line address 00084 lcdWriteCommand(0x81);//--set contrast control register 00085 lcdWriteCommand(0x7f); 00086 lcdWriteCommand(0xa1);//--set segment re-map 0 to 127 00087 lcdWriteCommand(0xa6);//--set normal display 00088 lcdWriteCommand(0xa8);//--set multiplex ratio(1 to 64) 00089 lcdWriteCommand(0x3F);// 00090 lcdWriteCommand(0xa4);//0xa4,Output follows RAM content;0xa5,Output ignores RAM content 00091 lcdWriteCommand(0xd3);//-set display offset 00092 lcdWriteCommand(0x00);//-not offset 00093 lcdWriteCommand(0xd5);//--set display clock divide ratio/oscillator frequency 00094 lcdWriteCommand(0xf0);//--set divide ratio 00095 lcdWriteCommand(0xd9);//--set pre-charge period 00096 lcdWriteCommand(0x22); // 00097 lcdWriteCommand(0xda);//--set com pins hardware configuration 00098 lcdWriteCommand(0x12); 00099 lcdWriteCommand(0xdb);//--set vcomh 00100 lcdWriteCommand(0x20);//0x20,0.77xVcc 00101 lcdWriteCommand(0x8d);//--set DC-DC enable 00102 lcdWriteCommand(0x14);// 00103 lcdWriteCommand(0xaf);//--turn on lcd panel 00104 } 00105 00106 void clear_LCD(void) 00107 { 00108 int16_t x, Y; 00109 for (Y=0;Y<LCD_Ymax/8;Y++) 00110 { 00111 lcdSetAddr(0, Y); 00112 for (x=0;x<LCD_Xmax;x++) 00113 lcdWriteData(0x00); 00114 } 00115 } 00116 00117 // print char function using Font5x7 00118 void printC_5x7 (int16_t x, int16_t y, unsigned char ascii_code) 00119 { 00120 uint8_t i; 00121 if (x<(LCD_Xmax-5) && y<(LCD_Ymax-7)) { 00122 if (ascii_code<0x20) ascii_code=0x20; 00123 else if (ascii_code>0x7F) ascii_code=0x20; 00124 for (i=0;i<5;i++) { 00125 lcdSetAddr((LCD_Xmax+1-x-i), (y/8)); 00126 lcdWriteData(Font5x7[(ascii_code-0x20)*5+5-i]); 00127 } 00128 } 00129 } 00130 00131 void print_C(uint8_t Line, uint8_t Col, char ascii) 00132 { 00133 uint8_t j, i, tmp; 00134 for (j=0;j<2;j++) { 00135 lcdSetAddr(Col*8, Line*2+j); 00136 for (i=0;i<8;i++) { 00137 tmp=Font8x16[(ascii-0x20)*16+j*8+i]; 00138 lcdWriteData(tmp); 00139 } 00140 } 00141 } 00142 00143 void print_Line(uint8_t line, char text[]) 00144 { 00145 uint8_t Col; 00146 for (Col=0; Col<strlen(text); Col++) 00147 print_C(line, Col, text[Col]); 00148 } 00149 00150 void printS(int16_t x, int16_t y, char text[]) 00151 { 00152 int8_t i; 00153 for (i=0;i<strlen(text);i++) 00154 print_C(x+i*8, y,text[i]); 00155 } 00156 00157 void printS_5x7(int16_t x, int16_t y, char text[]) 00158 { 00159 uint8_t i; 00160 for (i=0;i<strlen(text);i++) { 00161 printC_5x7(x,y,text[i]); 00162 x=x+5; 00163 } 00164 } 00165 00166 void draw_Pixel(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor) 00167 { 00168 if (fgColor!=0) 00169 DisplayBuffer[x+y/8*LCD_Xmax] |= (0x01<<(y%8)); 00170 else 00171 DisplayBuffer[x+y/8*LCD_Xmax] &= (0xFE<<(y%8)); 00172 00173 lcdSetAddr(x, y/8); 00174 lcdWriteData(DisplayBuffer[x+y/8*LCD_Xmax]); 00175 } 00176 00177 void draw_Bmp8x8(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00178 { 00179 uint8_t t,i,k, kx,ky; 00180 if (x<(LCD_Xmax-7) && y<(LCD_Ymax-7)) // boundary check 00181 for (i=0;i<8;i++){ 00182 kx=x+i; 00183 t=bitmap[i]; 00184 for (k=0;k<8;k++) { 00185 ky=y+k; 00186 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00187 } 00188 } 00189 } 00190 00191 void draw_Bmp32x8(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00192 { 00193 uint8_t t,i,k, kx,ky; 00194 if (x<(LCD_Xmax-7) && y<(LCD_Ymax-7)) // boundary check 00195 for (i=0;i<32;i++){ 00196 kx=x+i; 00197 t=bitmap[i]; 00198 for (k=0;k<8;k++) { 00199 ky=y+k; 00200 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00201 } 00202 } 00203 } 00204 00205 void draw_Bmp120x8(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00206 { 00207 uint8_t t,i,k, kx,ky; 00208 if (x<(LCD_Xmax-7) && y<(LCD_Ymax-7)) // boundary check 00209 for (i=0;i<120;i++){ 00210 kx=x+i; 00211 t=bitmap[i]; 00212 for (k=0;k<8;k++) { 00213 ky=y+k; 00214 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00215 } 00216 } 00217 } 00218 00219 void draw_Bmp8x16(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00220 { 00221 uint8_t t,i,k, kx,ky; 00222 if (x<(LCD_Xmax-7) && y<(LCD_Ymax-7)) // boundary check 00223 for (i=0;i<8;i++){ 00224 kx=x+i; 00225 t=bitmap[i]; 00226 for (k=0;k<8;k++) { 00227 ky=y+k; 00228 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00229 } 00230 t=bitmap[i+8]; 00231 for (k=0;k<8;k++) { 00232 ky=y+k+8; 00233 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00234 } 00235 } 00236 } 00237 00238 void draw_Bmp16x8(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00239 { 00240 uint8_t t,i,k,kx,ky; 00241 if (x<(LCD_Xmax-15) && y<(LCD_Ymax-7)) // boundary check 00242 for (i=0;i<16;i++) 00243 { 00244 kx=x+i; 00245 t=bitmap[i]; 00246 for (k=0;k<8;k++) { 00247 ky=y+k; 00248 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00249 } 00250 } 00251 } 00252 00253 void draw_Bmp16x16(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00254 { 00255 uint8_t t,i,j,k, kx,ky; 00256 if (x<(LCD_Xmax-15) && y<(LCD_Ymax-15)) // boundary check 00257 for (j=0;j<2; j++){ 00258 for (i=0;i<16;i++) { 00259 kx=x+i; 00260 t=bitmap[i+j*16]; 00261 for (k=0;k<8;k++) { 00262 ky=y+j*8+k; 00263 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00264 } 00265 } 00266 } 00267 } 00268 00269 void draw_Bmp16x24(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00270 { 00271 uint8_t t,i,j,k, kx,ky; 00272 if (x<(LCD_Xmax-15) && y<(LCD_Ymax-15)) // boundary check 00273 for (j=0;j<3; j++){ 00274 for (i=0;i<16;i++) { 00275 kx=x+i; 00276 t=bitmap[i+j*16]; 00277 for (k=0;k<8;k++) { 00278 ky=y+j*8+k; 00279 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00280 } 00281 } 00282 } 00283 } 00284 00285 void draw_Bmp16x32(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00286 { 00287 uint8_t t, i,j,k, kx,ky; 00288 if (x<(LCD_Xmax-15) && y<(LCD_Ymax-31)) // boundary check 00289 for (j=0;j<4; j++) { 00290 for (i=0;i<16;i++) { 00291 kx=x+i; 00292 t=bitmap[i+j*16]; 00293 for (k=0;k<8;k++) { 00294 ky=y+j*8+k; 00295 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00296 } 00297 } 00298 } 00299 } 00300 00301 void draw_Bmp16x40(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00302 { 00303 uint8_t t, i,j,k, kx,ky; 00304 if (x<(LCD_Xmax-15) && y<(LCD_Ymax-31)) // boundary check 00305 for (j=0;j<5; j++) { 00306 for (i=0;i<16;i++) { 00307 kx=x+i; 00308 t=bitmap[i+j*16]; 00309 for (k=0;k<8;k++) { 00310 ky=y+j*8+k; 00311 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00312 } 00313 } 00314 } 00315 } 00316 00317 void draw_Bmp16x48(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00318 { 00319 uint8_t t,i,j,k,kx,ky; 00320 if (x<(LCD_Xmax-15) && y<(LCD_Ymax-47)) // boundary check 00321 for (j=0;j<6; j++) { 00322 k=x; 00323 for (i=0;i<16;i++) { 00324 kx=x+i; 00325 t=bitmap[i+j*16]; 00326 for (k=0;k<8;k++) { 00327 ky=y+j*8+k; 00328 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00329 } 00330 } 00331 } 00332 } 00333 00334 void draw_Bmp16x64(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00335 { 00336 uint8_t t,i,j,k,kx,ky; 00337 if (x<(LCD_Xmax-15) && y==0) // boundary check 00338 for (j=0;j<8; j++) { 00339 k=x; 00340 for (i=0;i<16;i++) { 00341 kx=x+i; 00342 t=bitmap[i+j*16]; 00343 for (k=0;k<8;k++) { 00344 ky=y+j*8+k; 00345 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00346 } 00347 } 00348 } 00349 } 00350 00351 void draw_Bmp32x16(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00352 { 00353 uint8_t t,i,jx,jy,k,kx,ky; 00354 if (x<(LCD_Xmax-31) && y<(LCD_Ymax-15)) // boundary check 00355 for (jy=0;jy<2;jy++) 00356 for (jx=0;jx<2;jx++) { 00357 k=x; 00358 for (i=0;i<16;i++) { 00359 kx=x+jx*16+i; 00360 t=bitmap[i+jx*16+jy*32]; 00361 for (k=0;k<8;k++) { 00362 ky=y+jy*8+k; 00363 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00364 } 00365 } 00366 } 00367 } 00368 00369 void draw_Bmp32x32(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00370 { 00371 uint8_t t,i,jx,jy,k, kx,ky; 00372 if (x<(LCD_Xmax-31) && y<(LCD_Ymax-31)) // boundary check 00373 for (jy=0;jy<4;jy++) 00374 for (jx=0;jx<2;jx++) { 00375 k=x; 00376 for (i=0;i<16;i++) { 00377 kx=x+jx*16+i; 00378 t=bitmap[i+jx*16+jy*32]; 00379 for (k=0;k<8;k++) { 00380 ky=y+jy*8+k; 00381 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00382 } 00383 } 00384 } 00385 } 00386 00387 void draw_Bmp32x48(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00388 { 00389 uint8_t t,i,jx,jy,k, kx,ky; 00390 if (x<(LCD_Xmax-31) && y<(LCD_Ymax-47)) // boundary check 00391 for (jy=0;jy<6;jy++) 00392 for (jx=0;jx<2;jx++) { 00393 k=x; 00394 for (i=0;i<16;i++) { 00395 kx=x+jx*16+i; 00396 t=bitmap[i+jx*16+jy*32]; 00397 for (k=0;k<8;k++) { 00398 ky=y+jy*8+k; 00399 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00400 } 00401 } 00402 } 00403 } 00404 00405 void draw_Bmp32x64(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00406 { 00407 uint8_t t,i,jx,jy,k, kx,ky; 00408 if (x<(LCD_Xmax-31) && y==0) // boundary check 00409 for (jy=0;jy<8;jy++) 00410 for (jx=0;jx<2;jx++) { 00411 k=x; 00412 for (i=0;i<16;i++) { 00413 kx=x+jx*16+i; 00414 t=bitmap[i+jx*16+jy*32]; 00415 for (k=0;k<8;k++) { 00416 ky=y+jy*8+k; 00417 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00418 } 00419 } 00420 } 00421 } 00422 00423 void draw_Bmp64x64(int16_t x, int16_t y, uint16_t fgColor, uint16_t bgColor, unsigned char bitmap[]) 00424 { 00425 uint8_t t, i,jx,jy,k, kx,ky; 00426 if (x<(LCD_Xmax-63) && y==0) // boundary check 00427 for (jy=0;jy<8;jy++) 00428 for (jx=0;jx<4;jx++) { 00429 k=x; 00430 for (i=0;i<16;i++) { 00431 kx=x+jx*16+i; 00432 t=bitmap[i+jx*16+jy*64]; 00433 for (k=0;k<8;k++) { 00434 ky=y+jy*8+k; 00435 if (t&(0x01<<k)) draw_Pixel(kx,ky,fgColor,bgColor); 00436 } 00437 } 00438 } 00439 } 00440 00441 void draw_LCD(unsigned char *buffer) 00442 { 00443 uint8_t x,y; 00444 for (x=0; x<LCD_Xmax; x++) { 00445 for (y=0; y<(LCD_Ymax/8); y++) { 00446 lcdSetAddr(x ,y); 00447 lcdWriteData(buffer[x+y*LCD_Xmax]); 00448 } 00449 } 00450 } 00451
Generated on Wed Jul 20 2022 20:28:00 by
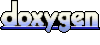