
File System Example - Writing a text file on FRMD-K64F board using 4GB Kingston SDHC
Dependencies: SDFileSystem mbed
Fork of FRDM_K64F-SDCard by
main.cpp
00001 /* 00002 ******************************************************************************* 00003 * HTTP://WWW.CERESCONTROLS.COM 00004 * PANAMÁ, REPÚBLICA DE PANAMÁ 00005 * 00006 * File : main.cpp 00007 * Programer(s) : Rangel Alvarado 00008 * Language : C/C++ 00009 * Description : Simple SD Card File System Write 00010 * 00011 * Notes : Using standard mbed classes to write information data in 00012 * the SD Card. 00013 * http://cache.freescale.com/files/32bit/doc/user_guide/FRDMK64FUG.pdf 00014 * 00015 ******************************************************************************* 00016 */ 00017 00018 #include "mbed.h" // mbed application libraries 00019 #include "SDFileSystem.h" // SD File System functions 00020 00021 #define DAT0 PTE3 // MOSI 00022 #define CMD PTE1 // MISO 00023 #define CLK PTE2 // SCLK 00024 #define CD PTE4 // CS 00025 00026 SDFileSystem sd(DAT0, CMD, CLK, CD, "sd"); // MOSI, MISO, SCLK, CS 00027 Serial pc(USBTX, USBRX); // Virtual COM Port 00028 00029 int main() { 00030 FILE *File = fopen("/sd/sdfile.txt", "w"); // open file 00031 if(File == NULL) { // check if present 00032 pc.printf("No SD Card or bad format\n"); // print message 00033 } else { // otherwise 00034 pc.printf("Ready to write\n"); // message preparing to write 00035 } 00036 fprintf(File, "FRDM-K64F"); // write data 00037 fclose(File); // close file on SD 00038 }
Generated on Wed Jul 13 2022 15:17:55 by
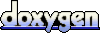