Nanopb is a plain-C implementation of Google's Protocol Buffers data format. It is targeted at 32 bit microcontrollers, but is also fit for other embedded systems with tight (2-10 kB ROM, <1 kB RAM) memory constraints.
Dependents: FBRLogger Dumb_box_rev2
pb.h
00001 #ifndef _PB_H_ 00002 #define _PB_H_ 00003 00004 /* pb.h: Common parts for nanopb library. 00005 * Most of these are quite low-level stuff. For the high-level interface, 00006 * see pb_encode.h or pb_decode.h 00007 */ 00008 00009 #define NANOPB_VERSION nanopb-0.1.9 00010 00011 #include <stdint.h> 00012 #include <stddef.h> 00013 #include <stdbool.h> 00014 00015 #ifdef __GNUC__ 00016 /* This just reduces memory requirements, but is not required. */ 00017 #define pb_packed __attribute__((packed)) 00018 #else 00019 #define pb_packed 00020 #endif 00021 00022 /* Handly macro for suppressing unreferenced-parameter compiler warnings. */ 00023 #ifndef UNUSED 00024 #define UNUSED(x) (void)(x) 00025 #endif 00026 00027 /* Compile-time assertion, used for checking compatible compilation options. 00028 * If this fails on your compiler for some reason, use #define STATIC_ASSERT 00029 * to disable it. */ 00030 #ifndef STATIC_ASSERT 00031 #define STATIC_ASSERT(COND,MSG) typedef char STATIC_ASSERT_MSG(MSG, __LINE__, __COUNTER__)[(COND)?1:-1]; 00032 #define STATIC_ASSERT_MSG(MSG, LINE, COUNTER) STATIC_ASSERT_MSG_(MSG, LINE, COUNTER) 00033 #define STATIC_ASSERT_MSG_(MSG, LINE, COUNTER) static_assertion_##MSG##LINE##COUNTER 00034 #endif 00035 00036 /* Number of required fields to keep track of 00037 * (change here or on compiler command line). */ 00038 #ifndef PB_MAX_REQUIRED_FIELDS 00039 #define PB_MAX_REQUIRED_FIELDS 64 00040 #endif 00041 00042 #if PB_MAX_REQUIRED_FIELDS < 64 00043 #error You should not lower PB_MAX_REQUIRED_FIELDS from the default value (64). 00044 #endif 00045 00046 /* List of possible field types. These are used in the autogenerated code. 00047 * Least-significant 4 bits tell the scalar type 00048 * Most-significant 4 bits specify repeated/required/packed etc. 00049 * 00050 * INT32 and UINT32 are treated the same, as are (U)INT64 and (S)FIXED* 00051 * These types are simply casted to correct field type when they are 00052 * assigned to the memory pointer. 00053 * SINT* is different, though, because it is zig-zag coded. 00054 */ 00055 00056 typedef enum { 00057 /************************ 00058 * Field contents types * 00059 ************************/ 00060 00061 /* Numeric types */ 00062 PB_LTYPE_VARINT = 0x00, /* int32, uint32, int64, uint64, bool, enum */ 00063 PB_LTYPE_SVARINT = 0x01, /* sint32, sint64 */ 00064 PB_LTYPE_FIXED32 = 0x02, /* fixed32, sfixed32, float */ 00065 PB_LTYPE_FIXED64 = 0x03, /* fixed64, sfixed64, double */ 00066 00067 /* Marker for last packable field type. */ 00068 PB_LTYPE_LAST_PACKABLE = 0x03, 00069 00070 /* Byte array with pre-allocated buffer. 00071 * data_size is the length of the allocated PB_BYTES_ARRAY structure. */ 00072 PB_LTYPE_BYTES = 0x04, 00073 00074 /* String with pre-allocated buffer. 00075 * data_size is the maximum length. */ 00076 PB_LTYPE_STRING = 0x05, 00077 00078 /* Submessage 00079 * submsg_fields is pointer to field descriptions */ 00080 PB_LTYPE_SUBMESSAGE = 0x06, 00081 00082 /* Number of declared LTYPES */ 00083 PB_LTYPES_COUNT = 7, 00084 PB_LTYPE_MASK = 0x0F, 00085 00086 /****************** 00087 * Modifier flags * 00088 ******************/ 00089 00090 /* Just the basic, write data at data_offset */ 00091 PB_HTYPE_REQUIRED = 0x00, 00092 00093 /* Write true at size_offset */ 00094 PB_HTYPE_OPTIONAL = 0x10, 00095 00096 /* Read to pre-allocated array 00097 * Maximum number of entries is array_size, 00098 * actual number is stored at size_offset */ 00099 PB_HTYPE_ARRAY = 0x20, 00100 00101 /* Works for all required/optional/repeated fields. 00102 * data_offset points to pb_callback_t structure. 00103 * LTYPE should be valid or 0 (it is ignored, but 00104 * sometimes used to speculatively index an array). */ 00105 PB_HTYPE_CALLBACK = 0x30, 00106 00107 PB_HTYPE_MASK = 0xF0 00108 } pb_packed pb_type_t; 00109 00110 #define PB_HTYPE(x) ((x) & PB_HTYPE_MASK) 00111 #define PB_LTYPE(x) ((x) & PB_LTYPE_MASK) 00112 00113 /* This structure is used in auto-generated constants 00114 * to specify struct fields. 00115 * You can change field sizes if you need structures 00116 * larger than 256 bytes or field tags larger than 256. 00117 * The compiler should complain if your .proto has such 00118 * structures. Fix that by defining PB_FIELD_16BIT or 00119 * PB_FIELD_32BIT. 00120 */ 00121 typedef struct _pb_field_t pb_field_t; 00122 struct _pb_field_t { 00123 00124 #if !defined(PB_FIELD_16BIT) && !defined(PB_FIELD_32BIT) 00125 uint8_t tag; 00126 pb_type_t type; 00127 uint8_t data_offset; /* Offset of field data, relative to previous field. */ 00128 int8_t size_offset; /* Offset of array size or has-boolean, relative to data */ 00129 uint8_t data_size; /* Data size in bytes for a single item */ 00130 uint8_t array_size; /* Maximum number of entries in array */ 00131 #elif defined(PB_FIELD_16BIT) && !defined(PB_FIELD_32BIT) 00132 uint16_t tag; 00133 pb_type_t type; 00134 uint8_t data_offset; 00135 int8_t size_offset; 00136 uint16_t data_size; 00137 uint16_t array_size; 00138 #else 00139 uint32_t tag; 00140 pb_type_t type; 00141 uint8_t data_offset; 00142 int8_t size_offset; 00143 uint32_t data_size; 00144 uint32_t array_size; 00145 #endif 00146 00147 /* Field definitions for submessage 00148 * OR default value for all other non-array, non-callback types 00149 * If null, then field will zeroed. */ 00150 const void *ptr; 00151 } pb_packed; 00152 00153 /* This structure is used for 'bytes' arrays. 00154 * It has the number of bytes in the beginning, and after that an array. 00155 * Note that actual structs used will have a different length of bytes array. 00156 */ 00157 struct _pb_bytes_array_t { 00158 size_t size; 00159 uint8_t bytes[1]; 00160 }; 00161 00162 typedef struct _pb_bytes_array_t pb_bytes_array_t; 00163 00164 /* This structure is used for giving the callback function. 00165 * It is stored in the message structure and filled in by the method that 00166 * calls pb_decode. 00167 * 00168 * The decoding callback will be given a limited-length stream 00169 * If the wire type was string, the length is the length of the string. 00170 * If the wire type was a varint/fixed32/fixed64, the length is the length 00171 * of the actual value. 00172 * The function may be called multiple times (especially for repeated types, 00173 * but also otherwise if the message happens to contain the field multiple 00174 * times.) 00175 * 00176 * The encoding callback will receive the actual output stream. 00177 * It should write all the data in one call, including the field tag and 00178 * wire type. It can write multiple fields. 00179 * 00180 * The callback can be null if you want to skip a field. 00181 */ 00182 typedef struct _pb_istream_t pb_istream_t; 00183 typedef struct _pb_ostream_t pb_ostream_t; 00184 typedef struct _pb_callback_t pb_callback_t; 00185 struct _pb_callback_t { 00186 union { 00187 bool (*decode)(pb_istream_t *stream, const pb_field_t *field, void *arg); 00188 bool (*encode)(pb_ostream_t *stream, const pb_field_t *field, const void *arg); 00189 } funcs; 00190 00191 /* Free arg for use by callback */ 00192 void *arg; 00193 }; 00194 00195 /* Wire types. Library user needs these only in encoder callbacks. */ 00196 typedef enum { 00197 PB_WT_VARINT = 0, 00198 PB_WT_64BIT = 1, 00199 PB_WT_STRING = 2, 00200 PB_WT_32BIT = 5 00201 } pb_wire_type_t; 00202 00203 /* These macros are used to declare pb_field_t's in the constant array. */ 00204 #define pb_membersize(st, m) (sizeof ((st*)0)->m) 00205 #define pb_arraysize(st, m) (pb_membersize(st, m) / pb_membersize(st, m[0])) 00206 #define pb_delta(st, m1, m2) ((int)offsetof(st, m1) - (int)offsetof(st, m2)) 00207 #define pb_delta_end(st, m1, m2) (offsetof(st, m1) - offsetof(st, m2) - pb_membersize(st, m2)) 00208 #define PB_LAST_FIELD {0,(pb_type_t) 0,0,0,0,0,0} 00209 00210 /* These macros are used for giving out error messages. 00211 * They are mostly a debugging aid; the main error information 00212 * is the true/false return value from functions. 00213 * Some code space can be saved by disabling the error 00214 * messages if not used. 00215 */ 00216 #ifdef PB_NO_ERRMSG 00217 #define PB_RETURN_ERROR(stream,msg) return false 00218 #define PB_GET_ERROR(stream) "(errmsg disabled)" 00219 #else 00220 #define PB_RETURN_ERROR(stream,msg) \ 00221 do {\ 00222 if ((stream)->errmsg == NULL) \ 00223 (stream)->errmsg = (msg); \ 00224 return false; \ 00225 } while(0) 00226 #define PB_GET_ERROR(stream) ((stream)->errmsg ? (stream)->errmsg : "(none)") 00227 #endif 00228 00229 #endif
Generated on Tue Jul 12 2022 21:22:20 by
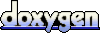