Dependencies: AmmoPusher AmmoSupplier Shooter
ShootingSystem.cpp
00001 #include "mbed.h" 00002 #include "ShootingSystem.h" 00003 #include "Command.h" 00004 #include "I2CServo.h" 00005 #include "Shooter.h" 00006 #include "AmmoPusher.h" 00007 #include "AmmoSupplier.h" 00008 00009 bool ShootingSystem::mIsShootable = false; 00010 00011 ShootingSystem::ShootingSystem( 00012 I2CServo* angleManagerServo, AmmoPusher* ammoPusher, 00013 AmmoSupplier* ammoSupplier, Shooter* shooter, 00014 I2CServo* positionManager ){ 00015 00016 mShooterAngleServo = angleManagerServo; 00017 mShooter = shooter; 00018 mAmmoPusher = ammoPusher; 00019 mAmmoSupplier = ammoSupplier; 00020 mPositionManager = positionManager; 00021 mState = SHOOT_PREPARATION; 00022 } 00023 00024 void ShootingSystem::update( Command command ){ 00025 bool isChangeable = ( mState != SHOOTING ); 00026 isChangeable &= ( mState != SHOOT_PREPARATION ); 00027 00028 if ( isChangeable ){ 00029 // 発射中でないなら角度と電圧が変更可能 00030 mShooterAngleServo->setTargetPosition( command.getShootingAngleAnalog() ); 00031 mPositionManager->setTargetPosition( command.getShooterPosition() ); 00032 mShooter->setVoltage( command.getShootVoltage() ); 00033 } 00034 00035 mIsShootable = ( mState == WAITING ); 00036 00037 switch ( mState ){ 00038 case WAITING: 00039 waiting( command ); 00040 break; 00041 00042 case SHOOTING: 00043 shooting(); 00044 break; 00045 00046 case SHOOT_WAITING: 00047 shootWaiting(); 00048 break; 00049 00050 case SHOOT_PREPARATION: 00051 shootPreparation(); 00052 break; 00053 00054 case AMMO_SUPPLYING: 00055 ammoSupply(); 00056 break; 00057 00058 default: 00059 break; 00060 } 00061 } 00062 00063 ShootingSystem::~ShootingSystem(){ 00064 delete mShooterAngleServo; 00065 delete mAmmoPusher; 00066 delete mAmmoSupplier; 00067 delete mShooter; 00068 delete mPositionManager; 00069 } 00070 00071 void ShootingSystem::waiting( Command command ){ 00072 if ( command.isSupplying() ){ 00073 mAmmoSupplier->supply(); 00074 mState = AMMO_SUPPLYING; 00075 } else if ( command.isShooting() ){ 00076 mState = SHOOTING; 00077 } 00078 } 00079 00080 void ShootingSystem::shooting(){ 00081 mShooter->launch(); 00082 mAmmoPusher->push(); 00083 00084 if ( mAmmoPusher->hasPushed() ){ 00085 mState = SHOOT_WAITING; 00086 mTimer.reset(); 00087 mTimer.start(); 00088 } 00089 } 00090 00091 void ShootingSystem::shootWaiting(){ 00092 mAmmoPusher->draw(); 00093 00094 if ( mTimer.read() > 0.7f ){ 00095 mShooter->stop(); 00096 mState = SHOOT_PREPARATION; 00097 } 00098 } 00099 00100 void ShootingSystem::shootPreparation(){ 00101 if ( mAmmoPusher->hasDrawn() ){ 00102 mState = WAITING; 00103 } 00104 } 00105 00106 void ShootingSystem::ammoSupply(){ 00107 if ( mAmmoSupplier->hasSupplied() ){ 00108 mState = WAITING; 00109 } 00110 }
Generated on Mon Aug 1 2022 21:30:00 by
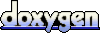