Embed:
(wiki syntax)
Show/hide line numbers
AmmoPusher.h
00001 #ifndef INCLUDED_AMMO_PUSHER_H 00002 #define INCLUDED_AMMO_PUSHER_H 00003 00004 #include "mbed.h" 00005 #include "I2CDevice.h" 00006 00007 class AmmoPusher : public I2CDevice{ 00008 public: 00009 enum ActionType{ 00010 NO_OPERATION, 00011 DRAWING, 00012 PUSHING 00013 }; 00014 enum State{ 00015 BETWEEN, 00016 HAS_FINISHED_DRAWING, 00017 HAS_FINISHED_PUSHING 00018 }; 00019 00020 AmmoPusher( char address ); 00021 00022 void push(){ 00023 setActionType( PUSHING ); 00024 } 00025 void draw(){ 00026 setActionType( DRAWING ); 00027 } 00028 void setActionType( ActionType action ){ 00029 mActionType = action; 00030 } 00031 State getState(){ 00032 return mState; 00033 } 00034 bool isWorking(){ 00035 return ( ( mState == BETWEEN ) && ( mActionType != NO_OPERATION ) ); 00036 } 00037 bool hasPushed(){ 00038 return ( mState == HAS_FINISHED_PUSHING ); 00039 } 00040 bool hasDrawn(){ 00041 return ( mState == HAS_FINISHED_DRAWING ); 00042 } 00043 00044 virtual int write(); 00045 virtual int read(); 00046 00047 private: 00048 ActionType mActionType; 00049 State mState; 00050 }; 00051 00052 #endif
Generated on Thu Jul 14 2022 05:47:12 by
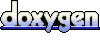