Syslog client for mbed-os 5
Fork of logger by
Embed:
(wiki syntax)
Show/hide line numbers
Logger.h
Go to the documentation of this file.
00001 /* 00002 * syslog device library 00003 * Copyright (c) 2011 Hiroshi Suga 00004 * Copyright (c) 2016 Colin Hogben 00005 * Released under the MIT License: http://mbed.org/license/mit 00006 */ 00007 00008 /** @file Logger.h 00009 * @brief syslog device (sender/client) 00010 */ 00011 00012 #ifndef LOGGER_H 00013 #define LOGGER_H 00014 00015 #include "mbed.h" 00016 #include "NetworkInterface.h" 00017 #include "UDPSocket.h" 00018 00019 /** 00020 * @enum Severity of priority 00021 */ 00022 enum LOG_SEVERITY { 00023 LOG_EMERG = 0, /* system is unusable */ 00024 LOG_ALERT = 1, /* action must be taken immediately */ 00025 LOG_CRIT = 2, /* critical conditions */ 00026 LOG_ERR = 3, /* error conditions */ 00027 LOG_WARNING = 4, /* warning conditions */ 00028 LOG_NOTICE = 5, /* normal but significant condition */ 00029 LOG_INFO = 6, /* informational */ 00030 LOG_DEBUG = 7, /* debug-level messages */ 00031 }; 00032 00033 /** 00034 * @enum Facility of priority 00035 */ 00036 enum LOG_FACILITY { 00037 LOG_KERN = 0, /* kernel messages */ 00038 LOG_USER = 1, /* user-level messages */ 00039 LOG_MAIL = 2, /* mail system */ 00040 LOG_DAEMON = 3, /* system daemons */ 00041 LOG_AUTH = 4, /* authorization messages */ 00042 LOG_SYSLOG = 5, /* messages generated internally by syslogd */ 00043 LOG_LPR = 6, /* line printer subsystem */ 00044 LOG_NEWS = 7, /* network news subsystem */ 00045 LOG_UUCP = 8, /* UUCP subsystem */ 00046 LOG_CRON = 9, /* clock daemon */ 00047 LOG_AUTHPRIV = 10, /* authorization messages = private */ 00048 LOG_FTP = 11, /* ftp daemon */ 00049 LOG_NTP = 12, /* NTP subsystem */ 00050 LOG_SECURITY = 13, /* security subsystems (audit) */ 00051 LOG_CONSOLE = 14, /* /dev/console output (alert) */ 00052 LOG_CLOCK = 15, /* clock daemon */ 00053 LOG_LOCAL0 = 16, /* reserved for local use */ 00054 LOG_LOCAL1 = 17, /* reserved for local use */ 00055 LOG_LOCAL2 = 18, /* reserved for local use */ 00056 LOG_LOCAL3 = 19, /* reserved for local use */ 00057 LOG_LOCAL4 = 20, /* reserved for local use */ 00058 LOG_LOCAL5 = 21, /* reserved for local use */ 00059 LOG_LOCAL6 = 22, /* reserved for local use */ 00060 LOG_LOCAL7 = 23, /* reserved for local use */ 00061 }; 00062 00063 /** brief syslog device (sender/client) 00064 */ 00065 class Logger { 00066 public: 00067 /** init logger class 00068 * @param p_eth EthernetNetIf class 00069 * @param host syslog collctor (server) 00070 */ 00071 Logger(NetworkInterface *, const char *); 00072 00073 /** init logger class 00074 * @param p_eth EthernetNetIf class 00075 * @param host syslog collctor (server) hostname or IP address 00076 * @param myname My hostname or IP address 00077 */ 00078 Logger(NetworkInterface *, const char *, const char *); 00079 00080 /** Send the message 00081 * @param tag Process name 00082 * @param content Message 00083 */ 00084 void send(LOG_SEVERITY, LOG_FACILITY, const char *, const char *); 00085 00086 /** Send the message 00087 * @param sev Severity 00088 * @param fac Facility 00089 * @param tag Process name 00090 * @param content Message 00091 */ 00092 void send(const char *, const char *); 00093 00094 private: 00095 void _Logger(NetworkInterface *netif, const char *host, const char *myname); 00096 00097 private: 00098 UDPSocket _udpsock; 00099 SocketAddress _remote; 00100 char _ident[32]; 00101 }; 00102 00103 #endif 00104
Generated on Fri Jul 15 2022 11:28:46 by
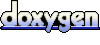