Syslog client for mbed-os 5
Fork of logger by
Embed:
(wiki syntax)
Show/hide line numbers
Logger.cpp
Go to the documentation of this file.
00001 /* 00002 * syslog device library 00003 * Copyright (c) 2011 Hiroshi Suga 00004 * Copyright (c) 2016 Colin Hogben 00005 * Released under the MIT License: http://mbed.org/license/mit 00006 */ 00007 00008 /** @file Logger.cpp 00009 * @brief syslog device (sender/client) 00010 */ 00011 00012 #include "Logger.h" 00013 #include <cstdio> 00014 00015 #ifdef MBED_CONF_LOGGER_LINE_MAX 00016 #define LOG_LEN MBED_CONF_LOGGER_LINE_MAX 00017 #else 00018 #define LOG_LEN 256 00019 #endif 00020 00021 #define LOG_UDPPORT 514 00022 00023 static const char mstr[12][4] = { 00024 "Jan", "Feb", "Mar", "Apr", "May", "Jun", 00025 "Jul", "Aug", "Sep", "Oct", "Nov", "Dec" 00026 }; 00027 00028 Logger::Logger(NetworkInterface *netif, const char *host) { 00029 _Logger(netif, host, netif->get_ip_address()); 00030 } 00031 00032 Logger::Logger(NetworkInterface *netif, const char *host, const char *myname) { 00033 _Logger(netif, host, myname); 00034 } 00035 00036 void Logger::_Logger(NetworkInterface *netif, const char *host, const char *myname) { 00037 _remote = SocketAddress(host, LOG_UDPPORT); 00038 snprintf(_ident, sizeof(_ident), "%s", myname); 00039 int err = _udpsock.open(netif); 00040 if (err) error("Cannot open syslog UDP socket"); 00041 _udpsock.bind(LOG_UDPPORT); 00042 } 00043 00044 void Logger::send(const char *tag, const char *content) { 00045 send(LOG_NOTICE, LOG_USER, tag, content); 00046 } 00047 00048 void Logger::send(LOG_SEVERITY sev, LOG_FACILITY fac, const char *tag, const char *content) { 00049 char logmsg[LOG_LEN]; 00050 00051 time_t ctTime = time(NULL); 00052 struct tm *t = localtime(&ctTime); 00053 int pri = (fac * 8) | sev; 00054 00055 snprintf(logmsg, sizeof(logmsg), 00056 "<%d>%s %2d %02d:%02d:%02d %s %s %s", 00057 pri, 00058 mstr[t->tm_mon], t->tm_mday, 00059 t->tm_hour, t->tm_min, t->tm_sec, 00060 _ident, 00061 tag, 00062 content); 00063 _udpsock.sendto(_remote, logmsg, strlen(logmsg)); 00064 }
Generated on Fri Jul 15 2022 11:28:46 by
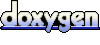