
GIU\ZF
Dependencies: MCP23017 WattBob_TextLCD mbed-rtos mbed
Fork of rtos_basic by
core.h
00001 #pragma once 00002 00003 #include "mbed.h" 00004 #include "rtos.h" 00005 #include "MCP23017.h" 00006 #include "WattBob_TextLCD.h" 00007 #include <string> 00008 #include <sstream> 00009 00010 //Compile Flags 00011 #define DEBUG_MODE 0 00012 00013 //Inputs------------------------- 00014 #define PORT_TURN_SIGNAL_SWITCH_RIGHT p17 00015 #define PORT_TURN_SIGNAL_SWITCH_LEFT p18 00016 #define PORT_ACCEL p19 00017 #define PORT_BRAKE p20 00018 #define PORT_IGNITION p21 00019 00020 //Outputs ---------------- 00021 #define PORT_REDBOX_LED1 p22 00022 #define PORT_REDBOX_LED2 p30 00023 00024 #define PORT_SIDE_LIGHTS LED1 00025 #define IGNITION_LED LED4 00026 #define PORT_TURN_SIGNAL_LED_LEFT LED2 00027 #define PORT_TURN_SIGNAL_LED_RIGHT LED2 00028 00029 //#define PORT_SIDE_LIGHTS LED1 00030 //#define IGNITION_LED LED4 00031 //#define PORT_TURN_SIGNAL_LED_LEFT LED2 00032 //#define PORT_TURN_SIGNAL_LED_RIGHT LED3 00033 00034 namespace runTimeParams{ 00035 extern Mutex liveAccess; 00036 extern float brakeForce; 00037 extern float accelForce; 00038 extern float avgSpeed; 00039 extern float odometer; 00040 extern float speed[3]; 00041 #if DEBUG_MODE 00042 extern Mutex debugAccess; 00043 extern string debugLogBuffer1; 00044 extern string debugLogBuffer2 ; 00045 extern string * debugLog ; 00046 #endif 00047 } 00048 00049 00050 00051 00052 namespace task_group_1{ 00053 //Display, 00054 extern Thread thread; 00055 void runTask(); 00056 } 00057 namespace task_group_2{ 00058 //Read Accel/Brake, carSimulator 00059 extern Thread thread; 00060 void runTask(); 00061 } 00062 00063 namespace mailData{ 00064 typedef struct{ 00065 float speed; 00066 float accel; 00067 float brake; 00068 } mail_t; 00069 extern Mail<mail_t, 100> mailBox; 00070 } 00071 00072 namespace enqueueMail{ 00073 //Send speed, accelerometer and brake values to a 100 element 00074 //MAIL queue 00075 extern Thread thread; 00076 void runTask(); 00077 } 00078 namespace dequeueMail{ 00079 //Send speed, accelerometer and brake values to a 100 element 00080 //MAIL queue 00081 extern Thread thread; 00082 extern const float freq; 00083 void runTask(); 00084 } 00085 00086 00087 template <typename T> 00088 static inline std::string to_string(T value) 00089 { 00090 std::ostringstream os ; 00091 os << value ; 00092 return os.str() ; 00093 }
Generated on Mon Jul 25 2022 05:11:11 by
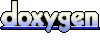