
NXP's driver library for LPC17xx, ported to mbed's online compiler. Not tested! I had to fix a lot of warings and found a couple of pretty obvious bugs, so the chances are there are more. Original: http://ics.nxp.com/support/documents/microcontrollers/zip/lpc17xx.cmsis.driver.library.zip
lpc_types.h
00001 /***********************************************************************//** 00002 * @file : lpc_types.h 00003 * @brief : 00004 * Contains the NXP ABL typedefs for C standard types. 00005 * It is intended to be used in ISO C conforming development 00006 * environments and checks for this insofar as it is possible 00007 * to do so. 00008 * @version : 1.0 00009 * @date : 27 Jul. 2008 00010 * @author : wellsk 00011 ************************************************************************** 00012 * Software that is described herein is for illustrative purposes only 00013 * which provides customers with programming information regarding the 00014 * products. This software is supplied "AS IS" without any warranties. 00015 * NXP Semiconductors assumes no responsibility or liability for the 00016 * use of the software, conveys no license or title under any patent, 00017 * copyright, or mask work right to the product. NXP Semiconductors 00018 * reserves the right to make changes in the software without 00019 * notification. NXP Semiconductors also make no representation or 00020 * warranty that such application will be suitable for the specified 00021 * use without further testing or modification. 00022 **************************************************************************/ 00023 00024 /* Type group ----------------------------------------------------------- */ 00025 /** @defgroup LPC_Types 00026 * @ingroup LPC1700CMSIS_FwLib_Drivers 00027 * @{ 00028 */ 00029 00030 #ifndef LPC_TYPES_H 00031 #define LPC_TYPES_H 00032 00033 /* Includes ------------------------------------------------------------------- */ 00034 #include <stdint.h> 00035 00036 00037 /* Public Types --------------------------------------------------------------- */ 00038 /** @defgroup LPC_Types_Public_Types 00039 * @{ 00040 */ 00041 00042 /** 00043 * @brief Boolean Type definition 00044 */ 00045 typedef enum {FALSE = 0, TRUE = !FALSE} Bool; 00046 00047 /** 00048 * @brief Flag Status and Interrupt Flag Status type definition 00049 */ 00050 typedef enum {RESET = 0, SET = !RESET} FlagStatus, IntStatus, SetState; 00051 #define PARAM_SETSTATE(State) ((State==RESET) || (State==SET)) 00052 00053 /** 00054 * @brief Functional State Definition 00055 */ 00056 typedef enum {DISABLE = 0, ENABLE = !DISABLE} FunctionalState; 00057 #define PARAM_FUNCTIONALSTATE(State) ((State==DISABLE) || (State==ENABLE)) 00058 00059 /** 00060 * @ Status type definition 00061 */ 00062 typedef enum {ERROR = 0, SUCCESS = !ERROR} Status; 00063 00064 00065 /** 00066 * Read/Write transfer type mode (Block or non-block) 00067 */ 00068 typedef enum 00069 { 00070 NONE_BLOCKING = 0, /**< None Blocking type */ 00071 BLOCKING, /**< Blocking type */ 00072 } TRANSFER_BLOCK_Type; 00073 00074 00075 /** Pointer to Function returning Void (any number of parameters) */ 00076 typedef void (*PFV)(); 00077 00078 /** Pointer to Function returning int32_t (any number of parameters) */ 00079 typedef int32_t(*PFI)(); 00080 00081 /** 00082 * @} 00083 */ 00084 00085 00086 /* Public Macros -------------------------------------------------------------- */ 00087 /** @defgroup LPC_Types_Public_Macros 00088 * @{ 00089 */ 00090 00091 /* _BIT(n) sets the bit at position "n" 00092 * _BIT(n) is intended to be used in "OR" and "AND" expressions: 00093 * e.g., "(_BIT(3) | _BIT(7))". 00094 */ 00095 #undef _BIT 00096 /* Set bit macro */ 00097 #define _BIT(n) (1UL<<n) 00098 00099 /* _SBF(f,v) sets the bit field starting at position "f" to value "v". 00100 * _SBF(f,v) is intended to be used in "OR" and "AND" expressions: 00101 * e.g., "((_SBF(5,7) | _SBF(12,0xF)) & 0xFFFF)" 00102 */ 00103 #undef _SBF 00104 /* Set bit field macro */ 00105 #define _SBF(f,v) (v<<f) 00106 00107 /* _BITMASK constructs a symbol with 'field_width' least significant 00108 * bits set. 00109 * e.g., _BITMASK(5) constructs '0x1F', _BITMASK(16) == 0xFFFF 00110 * The symbol is intended to be used to limit the bit field width 00111 * thusly: 00112 * <a_register> = (any_expression) & _BITMASK(x), where 0 < x <= 32. 00113 * If "any_expression" results in a value that is larger than can be 00114 * contained in 'x' bits, the bits above 'x - 1' are masked off. When 00115 * used with the _SBF example above, the example would be written: 00116 * a_reg = ((_SBF(5,7) | _SBF(12,0xF)) & _BITMASK(16)) 00117 * This ensures that the value written to a_reg is no wider than 00118 * 16 bits, and makes the code easier to read and understand. 00119 */ 00120 #undef _BITMASK 00121 /* Bitmask creation macro */ 00122 #define _BITMASK(field_width) ( _BIT(field_width) - 1) 00123 00124 /* NULL pointer */ 00125 #ifndef NULL 00126 #ifdef __cplusplus 00127 #define NULL 0 00128 #else 00129 #define NULL ((void *)0) 00130 #endif 00131 #endif 00132 00133 /* Number of elements in an array */ 00134 #define NELEMENTS(array) (sizeof (array) / sizeof (array[0])) 00135 00136 /* Static data/function define */ 00137 #define STATIC static 00138 /* External data/function define */ 00139 #define EXTERN extern 00140 00141 #define MAX(a, b) (((a) > (b)) ? (a) : (b)) 00142 #define MIN(a, b) (((a) < (b)) ? (a) : (b)) 00143 00144 /** 00145 * @} 00146 */ 00147 00148 00149 /* Old Type Definition compatibility ------------------------------------------ */ 00150 /** @addtogroup LPC_Types_Public_Types 00151 * @{ 00152 */ 00153 00154 /** SMA type for character type */ 00155 typedef char CHAR; 00156 00157 /** SMA type for 8 bit unsigned value */ 00158 typedef uint8_t UNS_8; 00159 00160 /** SMA type for 8 bit signed value */ 00161 typedef int8_t INT_8; 00162 00163 /** SMA type for 16 bit unsigned value */ 00164 typedef uint16_t UNS_16; 00165 00166 /** SMA type for 16 bit signed value */ 00167 typedef int16_t INT_16; 00168 00169 /** SMA type for 32 bit unsigned value */ 00170 typedef uint32_t UNS_32; 00171 00172 /** SMA type for 32 bit signed value */ 00173 typedef int32_t INT_32; 00174 00175 /** SMA type for 64 bit signed value */ 00176 typedef int64_t INT_64; 00177 00178 /** SMA type for 64 bit unsigned value */ 00179 typedef uint64_t UNS_64; 00180 00181 /** 32 bit boolean type */ 00182 typedef Bool BOOL_32; 00183 00184 /** 16 bit boolean type */ 00185 typedef Bool BOOL_16; 00186 00187 /** 8 bit boolean type */ 00188 typedef Bool BOOL_8; 00189 00190 /** 00191 * @} 00192 */ 00193 00194 00195 #endif /* LPC_TYPES_H */ 00196 00197 /** 00198 * @} 00199 */ 00200 00201 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 17:06:02 by
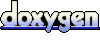