Low level MQTTSN packet library, part of the Eclipse Paho project: http://eclipse.org/paho
Dependents: MQTTSN sara-n200-hello-mqtt-sn MQTTSN_2
MQTTSNSubscribeServer.c
00001 /******************************************************************************* 00002 * Copyright (c) 2014 IBM Corp. 00003 * 00004 * All rights reserved. This program and the accompanying materials 00005 * are made available under the terms of the Eclipse Public License v1.0 00006 * and Eclipse Distribution License v1.0 which accompany this distribution. 00007 * 00008 * The Eclipse Public License is available at 00009 * http://www.eclipse.org/legal/epl-v10.html 00010 * and the Eclipse Distribution License is available at 00011 * http://www.eclipse.org/org/documents/edl-v10.php. 00012 * 00013 * Contributors: 00014 * Ian Craggs - initial API and implementation and/or initial documentation 00015 *******************************************************************************/ 00016 00017 #include "StackTrace.h" 00018 #include "MQTTSNPacket.h" 00019 #include <string.h> 00020 00021 00022 /** 00023 * Deserializes the supplied (wire) buffer into subscribe data 00024 * @param dup the returned MQTT-SN dup flag 00025 * @param qos the returned qos 00026 * @param packetid returned - the same value as the one contained in the corresponding SUBSCRIBE 00027 * @param topicFilter returned - the topic filter - normal, predefined or short 00028 * @param buf the raw buffer data, of the correct length determined by the remaining length field 00029 * @param buflen the length in bytes of the data in the supplied buffer 00030 * @return error code. 1 is success 00031 */ 00032 int MQTTSNDeserialize_subscribe(unsigned char* dup, int* qos, unsigned short* packetid, 00033 MQTTSN_topicid* topicFilter, unsigned char* buf, int buflen) 00034 { 00035 MQTTSNFlags flags = {0}; 00036 unsigned char* curdata = buf; 00037 unsigned char* enddata = NULL; 00038 int rc = 0; 00039 int mylen = 0; 00040 00041 FUNC_ENTRY; 00042 curdata += (rc = MQTTSNPacket_decode(curdata, buflen, &mylen)); /* read length */ 00043 enddata = buf + mylen; 00044 if (enddata - curdata > buflen) 00045 goto exit; 00046 00047 if (MQTTSNPacket_readChar(&curdata) != MQTTSN_SUBSCRIBE) 00048 goto exit; 00049 00050 flags.all = MQTTSNPacket_readChar(&curdata); 00051 *dup = flags.bits.dup; 00052 *qos = flags.bits.QoS; 00053 00054 *packetid = MQTTSNPacket_readInt(&curdata); 00055 00056 topicFilter->type = flags.bits.topicIdType; 00057 00058 if (topicFilter->type == MQTTSN_TOPIC_TYPE_NORMAL) 00059 { 00060 topicFilter->data.long_.len = enddata - curdata; 00061 topicFilter->data.long_.name = (char*)curdata; 00062 } 00063 else if (topicFilter->type == MQTTSN_TOPIC_TYPE_PREDEFINED) 00064 topicFilter->data.id = MQTTSNPacket_readInt(&curdata); 00065 else if (topicFilter->type == MQTTSN_TOPIC_TYPE_SHORT) 00066 { 00067 topicFilter->data.short_name[0] = MQTTSNPacket_readChar(&curdata); 00068 topicFilter->data.short_name[1] = MQTTSNPacket_readChar(&curdata); 00069 } 00070 00071 rc = 1; 00072 exit: 00073 FUNC_EXIT_RC(rc); 00074 return rc; 00075 } 00076 00077 00078 /** 00079 * Serializes the supplied suback data into the supplied buffer, ready for sending 00080 * @param buf the buffer into which the packet will be serialized 00081 * @param buflen the length in bytes of the supplied buffer 00082 * @param qos integer - the MQTT-SN QoS value 00083 * @param topicid if "accepted" the value which will be used by the gateway in subsequent PUBLISH packets 00084 * @param packetid integer - the MQTT-SN packet identifier 00085 * @param returncode returned - "accepted" or rejection reason 00086 * @return the length of the serialized data. <= 0 indicates error 00087 */ 00088 int MQTTSNSerialize_suback(unsigned char* buf, int buflen, int qos, unsigned short topicid, unsigned short packetid, 00089 unsigned char returncode) 00090 { 00091 MQTTSNFlags flags = {0}; 00092 unsigned char *ptr = buf; 00093 int len = 0; 00094 int rc = 0; 00095 00096 FUNC_ENTRY; 00097 if ((len = MQTTSNPacket_len(7)) > buflen) 00098 { 00099 rc = MQTTSNPACKET_BUFFER_TOO_SHORT; 00100 goto exit; 00101 } 00102 ptr += MQTTSNPacket_encode(ptr, len); /* write length */ 00103 MQTTSNPacket_writeChar(&ptr, MQTTSN_SUBACK); /* write message type */ 00104 00105 flags.all = 0; 00106 flags.bits.QoS = qos; 00107 MQTTSNPacket_writeChar(&ptr, flags.all); 00108 00109 MQTTSNPacket_writeInt(&ptr, topicid); 00110 MQTTSNPacket_writeInt(&ptr, packetid); 00111 MQTTSNPacket_writeChar(&ptr, returncode); 00112 00113 rc = ptr - buf; 00114 exit: 00115 FUNC_EXIT_RC(rc); 00116 return rc; 00117 } 00118 00119 00120
Generated on Tue Jul 12 2022 20:35:37 by
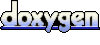