Low level MQTTSN packet library, part of the Eclipse Paho project: http://eclipse.org/paho
Dependents: MQTTSN sara-n200-hello-mqtt-sn MQTTSN_2
MQTTSNPacket.h
00001 /******************************************************************************* 00002 * Copyright (c) 2014 IBM Corp. 00003 * 00004 * All rights reserved. This program and the accompanying materials 00005 * are made available under the terms of the Eclipse Public License v1.0 00006 * and Eclipse Distribution License v1.0 which accompany this distribution. 00007 * 00008 * The Eclipse Public License is available at 00009 * http://www.eclipse.org/legal/epl-v10.html 00010 * and the Eclipse Distribution License is available at 00011 * http://www.eclipse.org/org/documents/edl-v10.php. 00012 * 00013 * Contributors: 00014 * Ian Craggs - initial API and implementation and/or initial documentation 00015 *******************************************************************************/ 00016 00017 #ifndef MQTTSNPACKET_H_ 00018 #define MQTTSNPACKET_H_ 00019 00020 #if defined(__cplusplus) /* If this is a C++ compiler, use C linkage */ 00021 extern "C" { 00022 #endif 00023 00024 enum MQTTSN_errors 00025 { 00026 MQTTSNPACKET_BUFFER_TOO_SHORT = -2, 00027 MQTTSNPACKET_READ_ERROR = -1, 00028 MQTTSNPACKET_READ_COMPLETE, 00029 }; 00030 00031 #define MQTTSN_PROTOCOL_VERSION 0x01 00032 00033 enum MQTTSN_connackCodes 00034 { 00035 MQTTSN_RC_ACCEPTED, 00036 MQTTSN_RC_REJECTED_CONGESTED, 00037 MQTTSN_RC_REJECTED_INVALID_TOPIC_ID, 00038 }; 00039 00040 enum MQTTSN_topicTypes 00041 { 00042 MQTTSN_TOPIC_TYPE_NORMAL, /* topic id in publish, topic name in subscribe */ 00043 MQTTSN_TOPIC_TYPE_PREDEFINED, 00044 MQTTSN_TOPIC_TYPE_SHORT, 00045 }; 00046 00047 00048 enum MQTTSN_msgTypes 00049 { 00050 MQTTSN_ADVERTISE, MQTTSN_SEARCHGW, MQTTSN_GWINFO, MQTTSN_RESERVED1, 00051 MQTTSN_CONNECT, MQTTSN_CONNACK, 00052 MQTTSN_WILLTOPICREQ, MQTTSN_WILLTOPIC, MQTTSN_WILLMSGREQ, MQTTSN_WILLMSG, 00053 MQTTSN_REGISTER, MQTTSN_REGACK, 00054 MQTTSN_PUBLISH, MQTTSN_PUBACK, MQTTSN_PUBCOMP, MQTTSN_PUBREC, MQTTSN_PUBREL, MQTTSN_RESERVED2, 00055 MQTTSN_SUBSCRIBE, MQTTSN_SUBACK, MQTTSN_UNSUBSCRIBE, MQTTSN_UNSUBACK, 00056 MQTTSN_PINGREQ, MQTTSN_PINGRESP, 00057 MQTTSN_DISCONNECT, MQTTSN_RESERVED3, 00058 MQTTSN_WILLTOPICUPD, MQTTSN_WILLTOPICRESP, MQTTSN_WILLMSGUPD, MQTTSN_WILLMSGRESP, 00059 }; 00060 00061 typedef struct 00062 { 00063 enum MQTTSN_topicTypes type; 00064 union 00065 { 00066 unsigned short id; 00067 char short_name[2]; 00068 struct 00069 { 00070 char* name; 00071 int len; 00072 } long_; 00073 } data; 00074 } MQTTSN_topicid; 00075 00076 00077 /** 00078 * Bitfields for the MQTT-SN flags byte. 00079 */ 00080 typedef union 00081 { 00082 unsigned char all; 00083 #if defined(REVERSED) 00084 struct 00085 { 00086 int dup: 1; 00087 unsigned int QoS : 2; 00088 unsigned int retain : 1; 00089 unsigned int will : 1; 00090 unsigned int cleanSession : 1; 00091 unsigned int topicIdType : 2; 00092 } bits; 00093 #else 00094 struct 00095 { 00096 unsigned int topicIdType : 2; 00097 unsigned int cleanSession : 1; 00098 unsigned int will : 1; 00099 unsigned int retain : 1; 00100 unsigned int QoS : 2; 00101 int dup: 1; 00102 } bits; 00103 #endif 00104 } MQTTSNFlags; 00105 00106 00107 typedef struct 00108 { 00109 int len; 00110 char* data; 00111 } MQTTSNLenString; 00112 00113 typedef struct 00114 { 00115 char* cstring; 00116 MQTTSNLenString lenstring; 00117 } MQTTSNString; 00118 00119 #define MQTTSNString_initializer {NULL, {0, NULL}} 00120 00121 int MQTTSNstrlen(MQTTSNString mqttsnstring); 00122 00123 #include "MQTTSNConnect.h" 00124 #include "MQTTSNPublish.h" 00125 #include "MQTTSNSubscribe.h" 00126 #include "MQTTSNUnsubscribe.h" 00127 #include "MQTTSNSearch.h" 00128 00129 char* MQTTSNPacket_name(int ptype); 00130 int MQTTSNPacket_len(int length); 00131 00132 int MQTTSNPacket_encode(unsigned char* buf, int length); 00133 int MQTTSNPacket_decode(unsigned char* buf, int buflen, int* value); 00134 00135 int MQTTSNPacket_readInt(unsigned char** pptr); 00136 char MQTTSNPacket_readChar(unsigned char** pptr); 00137 void MQTTSNPacket_writeChar(unsigned char** pptr, char c); 00138 void MQTTSNPacket_writeInt(unsigned char** pptr, int anInt); 00139 int readMQTTSNString(MQTTSNString* mqttstring, unsigned char** pptr, unsigned char* enddata); 00140 void MQTTSNPacket_writeCString(unsigned char** pptr, char* string); 00141 void writeMQTTSNString(unsigned char** pptr, MQTTSNString mqttstring); 00142 00143 int MQTTSNPacket_read(unsigned char* buf, int buflen, int (*getfn)(unsigned char*, int)); 00144 00145 #ifdef __cplusplus /* If this is a C++ compiler, use C linkage */ 00146 } 00147 #endif 00148 00149 00150 #endif /* MQTTSNPACKET_H_ */ 00151
Generated on Tue Jul 12 2022 20:35:37 by
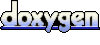