mbed library sources: Modified to operate FRDM-KL25Z at 48MHz from internal 32kHz oscillator (nothing else changed).
Fork of mbed-src by
InterruptIn.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_INTERRUPTIN_H 00017 #define MBED_INTERRUPTIN_H 00018 00019 #include "platform.h" 00020 00021 #if DEVICE_INTERRUPTIN 00022 00023 #include "gpio_api.h" 00024 #include "gpio_irq_api.h" 00025 00026 #include "FunctionPointer.h" 00027 #include "CallChain.h" 00028 00029 namespace mbed { 00030 00031 /** A digital interrupt input, used to call a function on a rising or falling edge 00032 * 00033 * Example: 00034 * @code 00035 * // Flash an LED while waiting for events 00036 * 00037 * #include "mbed.h" 00038 * 00039 * InterruptIn event(p16); 00040 * DigitalOut led(LED1); 00041 * 00042 * void trigger() { 00043 * printf("triggered!\n"); 00044 * } 00045 * 00046 * int main() { 00047 * event.rise(&trigger); 00048 * while(1) { 00049 * led = !led; 00050 * wait(0.25); 00051 * } 00052 * } 00053 * @endcode 00054 */ 00055 class InterruptIn { 00056 00057 public: 00058 00059 /** Create an InterruptIn connected to the specified pin 00060 * 00061 * @param pin InterruptIn pin to connect to 00062 * @param name (optional) A string to identify the object 00063 */ 00064 InterruptIn(PinName pin); 00065 virtual ~InterruptIn(); 00066 00067 int read(); 00068 #ifdef MBED_OPERATORS 00069 operator int(); 00070 00071 #endif 00072 00073 /** Attach a function to call when a rising edge occurs on the input 00074 * 00075 * @param fptr A pointer to a void function, or 0 to set as none 00076 * 00077 * @returns 00078 * The function object created for 'fptr' 00079 */ 00080 pFunctionPointer_t rise(void (*fptr)(void)); 00081 00082 /** Add a function to be called when a rising edge occurs at the end of the call chain 00083 * 00084 * @param fptr the function to add 00085 * 00086 * @returns 00087 * The function object created for 'fptr' 00088 */ 00089 pFunctionPointer_t rise_add(void (*fptr)(void)) { 00090 return rise_add_common(fptr); 00091 } 00092 00093 /** Add a function to be called when a rising edge occurs at the beginning of the call chain 00094 * 00095 * @param fptr the function to add 00096 * 00097 * @returns 00098 * The function object created for 'fptr' 00099 */ 00100 pFunctionPointer_t rise_add_front(void (*fptr)(void)) { 00101 return rise_add_common(fptr, true); 00102 } 00103 00104 /** Attach a member function to call when a rising edge occurs on the input 00105 * 00106 * @param tptr pointer to the object to call the member function on 00107 * @param mptr pointer to the member function to be called 00108 * 00109 * @returns 00110 * The function object created for 'tptr' and 'mptr' 00111 */ 00112 template<typename T> 00113 pFunctionPointer_t rise(T* tptr, void (T::*mptr)(void)) { 00114 _rise.clear(); 00115 pFunctionPointer_t pf = _rise.add(tptr, mptr); 00116 gpio_irq_set(&gpio_irq, IRQ_RISE, 1); 00117 return pf; 00118 } 00119 00120 /** Add a function to be called when a rising edge occurs at the end of the call chain 00121 * 00122 * @param tptr pointer to the object to call the member function on 00123 * @param mptr pointer to the member function to be called 00124 * 00125 * @returns 00126 * The function object created for 'tptr' and 'mptr' 00127 */ 00128 template<typename T> 00129 pFunctionPointer_t rise_add(T* tptr, void (T::*mptr)(void)) { 00130 return rise_add_common(tptr, mptr); 00131 } 00132 00133 /** Add a function to be called when a rising edge occurs at the beginning of the call chain 00134 * 00135 * @param tptr pointer to the object to call the member function on 00136 * @param mptr pointer to the member function to be called 00137 * 00138 * @returns 00139 * The function object created for 'tptr' and 'mptr' 00140 */ 00141 template<typename T> 00142 pFunctionPointer_t rise_add_front(T* tptr, void (T::*mptr)(void)) { 00143 return rise_add_common(tptr, mptr, true); 00144 } 00145 00146 /** Remove a function from the list of functions to be called when a rising edge occurs 00147 * 00148 * @param pf the function object to remove 00149 * 00150 * @returns 00151 * true if the function was found and removed, false otherwise 00152 */ 00153 bool rise_remove(pFunctionPointer_t pf); 00154 00155 /** Attach a function to call when a falling edge occurs on the input 00156 * 00157 * @param fptr A pointer to a void function, or 0 to set as none 00158 * 00159 * @returns 00160 * The function object created for 'fptr' 00161 */ 00162 pFunctionPointer_t fall(void (*fptr)(void)); 00163 00164 /** Add a function to be called when a falling edge occurs at the end of the call chain 00165 * 00166 * @param fptr the function to add 00167 * 00168 * @returns 00169 * The function object created for 'fptr' 00170 */ 00171 pFunctionPointer_t fall_add(void (*fptr)(void)) { 00172 return fall_add_common(fptr); 00173 } 00174 00175 /** Add a function to be called when a falling edge occurs at the beginning of the call chain 00176 * 00177 * @param fptr the function to add 00178 * 00179 * @returns 00180 * The function object created for 'fptr' 00181 */ 00182 pFunctionPointer_t fall_add_front(void (*fptr)(void)) { 00183 return fall_add_common(fptr, true); 00184 } 00185 00186 /** Attach a member function to call when a falling edge occurs on the input 00187 * 00188 * @param tptr pointer to the object to call the member function on 00189 * @param mptr pointer to the member function to be called 00190 * 00191 * @returns 00192 * The function object created for 'tptr' and 'mptr' 00193 */ 00194 template<typename T> 00195 pFunctionPointer_t fall(T* tptr, void (T::*mptr)(void)) { 00196 _fall.clear(); 00197 pFunctionPointer_t pf = _fall.add(tptr, mptr); 00198 gpio_irq_set(&gpio_irq, IRQ_FALL, 1); 00199 return pf; 00200 } 00201 00202 /** Add a function to be called when a falling edge occurs at the end of the call chain 00203 * 00204 * @param tptr pointer to the object to call the member function on 00205 * @param mptr pointer to the member function to be called 00206 * 00207 * @returns 00208 * The function object created for 'tptr' and 'mptr' 00209 */ 00210 template<typename T> 00211 pFunctionPointer_t fall_add(T* tptr, void (T::*mptr)(void)) { 00212 return fall_add_common(tptr, mptr); 00213 } 00214 00215 /** Add a function to be called when a falling edge occurs at the beginning of the call chain 00216 * 00217 * @param tptr pointer to the object to call the member function on 00218 * @param mptr pointer to the member function to be called 00219 * 00220 * @returns 00221 * The function object created for 'tptr' and 'mptr' 00222 */ 00223 template<typename T> 00224 pFunctionPointer_t fall_add_front(T* tptr, void (T::*mptr)(void)) { 00225 return fall_add_common(tptr, mptr, true); 00226 } 00227 00228 /** Remove a function from the list of functions to be called when a falling edge occurs 00229 * 00230 * @param pf the function object to remove 00231 * 00232 * @returns 00233 * true if the function was found and removed, false otherwise 00234 */ 00235 bool fall_remove(pFunctionPointer_t pf); 00236 00237 /** Set the input pin mode 00238 * 00239 * @param mode PullUp, PullDown, PullNone 00240 */ 00241 void mode(PinMode pull); 00242 00243 static void _irq_handler(uint32_t id, gpio_irq_event event); 00244 00245 protected: 00246 pFunctionPointer_t rise_add_common(void (*fptr)(void), bool front=false); 00247 pFunctionPointer_t fall_add_common(void (*fptr)(void), bool front=false); 00248 00249 template<typename T> 00250 pFunctionPointer_t rise_add_common(T* tptr, void (T::*mptr)(void), bool front=false) { 00251 pFunctionPointer_t pf = front ? _rise.add_front(tptr, mptr) : _rise.add(tptr, mptr); 00252 gpio_irq_set(&gpio_irq, IRQ_RISE, 1); 00253 return pf; 00254 } 00255 template<typename T> 00256 pFunctionPointer_t fall_add_common(T* tptr, void (T::*mptr)(void), bool front=false) { 00257 pFunctionPointer_t pf = front ? _fall.add_front(tptr, mptr) : _fall.add(tptr, mptr); 00258 gpio_irq_set(&gpio_irq, IRQ_FALL, 1); 00259 return pf; 00260 } 00261 00262 gpio_t gpio; 00263 gpio_irq_t gpio_irq; 00264 00265 CallChain _rise; 00266 CallChain _fall; 00267 }; 00268 00269 } // namespace mbed 00270 00271 #endif 00272 00273 #endif
Generated on Wed Jul 13 2022 19:29:54 by
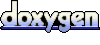