hej
Embed:
(wiki syntax)
Show/hide line numbers
hack_motor.cpp
00001 #include "mbed.h" 00002 #include "hack_motor.h" 00003 #include "PwmOut.h" 00004 00005 Wheel::Wheel() : M1A(PTC8), M1B(PTC10), M2A(PTC9), M2B(PTC11) 00006 { 00007 init(); 00008 } 00009 00010 00011 void Wheel::init() //Initialize the driver pwm to 150Hz 00012 { 00013 M1A.period(0.0066); 00014 M2A.period(0.0066); 00015 speed = 0.0; 00016 } 00017 00018 void Wheel::FW() 00019 { 00020 fw = 0.5+(0.5*speed); //forward lies in the upper 50% of the duty cycle 00021 M1A.write(fw); //Set the duty cycle to the wanted percent, from speed variable 00022 M2A.write(fw); // -//- 00023 M1B = 0; 00024 M2B = 0; 00025 wait_ms(1); 00026 } 00027 00028 void Wheel::BW() 00029 { 00030 bw = 0.5-(0.5*speed); //Backward lies within the lower 50% of the duty cycle 00031 M1A.write(bw); //Set the duty cycle to the wanted percent, from speed variable 00032 M2A.write(bw); // -//- 00033 M1B = 1; 00034 M2B = 1; 00035 } 00036 00037 void Wheel::right() 00038 { 00039 M1A.write(0.75); //Left side forward 50% 00040 M2A.write(0.25); //Right side backwards 50% 00041 M1B = 0; 00042 M2B = 1; 00043 } 00044 00045 void Wheel::left() 00046 { 00047 M1A.write(0.25); //Right side forward 50% 00048 M2A.write(0.75); //Left side backwards 50% 00049 M1B = 1; 00050 M2B = 0; 00051 } 00052 00053 void Wheel::stop() 00054 { 00055 M1A.write(0.0); //Pin A's set low 00056 M2A.write(0.0); 00057 M1B = 0; 00058 M2B = 0; //Pin B's set high 00059 }
Generated on Sat Jul 16 2022 01:46:21 by
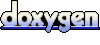