This code uses a uLCD-144-G2 128 by 128 Smart Color LCD to display the humidity and temperature in celcius, kelvin, and fahrenheit.
main.cpp
00001 /** Temperature program to output to ULCD 00002 * 00003 * @author Alan Lai & Nelson Diaz 00004 * The Georgia Institute of Technology 00005 * ECE 4180 Embeded Systems 00006 * Professor Hamblen 00007 * 03/28/2014 00008 * 00009 * @section LICENSE 00010 * ---------------------------------------------------------------------------- 00011 * "THE BEER-WARE LICENSE" (Revision 42): 00012 * <alanhlai91@gmail.com> wrote this file. As long as you retain this notice you 00013 * can do whatever you want with this stuff. If we meet some day, and you think 00014 * this stuff is worth it, you can buy me a beer in return. 00015 * ---------------------------------------------------------------------------- 00016 * 00017 * 00018 * @section DESCRIPTION 00019 * 00020 * Honeywell HTU21D Humidity and Temperature sensor. 00021 * 00022 * Datasheet, specs, and information: 00023 * 00024 * https://www.sparkfun.com/products/12064 00025 */ 00026 00027 00028 #include "mbed.h" 00029 #include "uLCD_4DGL.h" 00030 #include "rtos.h" 00031 #include "HTU21D.h" 00032 00033 00034 Semaphore four_slots(1); //Activate semaphore 00035 00036 uLCD_4DGL uLCD(p28,p27,p29); // serial tx, serial rx, reset pin; 00037 HTU21D temphumid(p9, p10); // Temp Module || sda, SCL 00038 00039 void sample_humidity(void const *args) { //line 2 00040 while(true){ 00041 00042 four_slots.wait(); 00043 uLCD.color(0x0000FF); 00044 uLCD.locate(1,2); //col,row 00045 wait(0.1); 00046 uLCD.printf("Humidy Is: %d %%", temphumid.sample_humid()); 00047 four_slots.release(); 00048 Thread::wait(250); 00049 } 00050 } 00051 00052 00053 void sample_temperature(void const *args) { // line 4 00054 while(true){ 00055 four_slots.wait(); 00056 uLCD.color(0xFF0000); 00057 uLCD.locate(1,4); //col,row 00058 wait(0.1); 00059 uLCD.printf("Temp Is: %d° C", temphumid.sample_ctemp()); 00060 uLCD.color(0xFFFF00); 00061 uLCD.locate(1,6); 00062 wait(0.1); 00063 uLCD.printf("Temp Is: %d° F", temphumid.sample_ftemp()); 00064 uLCD.color(0xC0C0C0); 00065 uLCD.locate(1,8); 00066 wait(0.1); 00067 uLCD.printf("Temp Is: %d° k", temphumid.sample_ktemp()); 00068 four_slots.release(); 00069 Thread::wait(1000); 00070 } 00071 } 00072 00073 00074 int main() { 00075 uLCD.baudrate(3000000); 00076 Thread t1(sample_humidity); 00077 Thread t2(sample_temperature); 00078 while(1){ //While so program doesn't end 00079 00080 } 00081 }
Generated on Sun Jul 17 2022 23:12:07 by
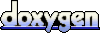