BASIC LIBRARY TO INTERFACE WITH HTU21D TEMPERATURE AND HUMIDITY SENSOR
Dependents: Natural_Calamities_Monitoring_System Nucleo_HTU21D-F Nucleo_motors lpc1768_blinky ... more
HTU21D.h
00001 /** 00002 * @author Alan Lai & Nelson Diaz 00003 * The Georgia Institute of Technology 00004 * ECE 4180 Embeded Systems 00005 * Professor Hamblen 00006 * 03/28/2014 00007 * 00008 * @section LICENSE 00009 * ---------------------------------------------------------------------------- 00010 * "THE BEER-WARE LICENSE" (Revision 42): 00011 * <alanhlai91@gmail.com> wrote this file. As long as you retain this notice you 00012 * can do whatever you want with this stuff. If we meet some day, and you think 00013 * this stuff is worth it, you can buy me a beer in return. 00014 * ---------------------------------------------------------------------------- 00015 * 00016 * 00017 * @section DESCRIPTION 00018 * 00019 * Honeywell HTU21D Humidity and Temperature sensor. 00020 * 00021 * Datasheet, specs, and information: 00022 * 00023 * https://www.sparkfun.com/products/12064 00024 */ 00025 00026 #ifndef HTU21D_H 00027 #define HTU21D_H 00028 00029 /** 00030 * Includes 00031 */ 00032 #include "mbed.h" 00033 00034 /** 00035 * Defines 00036 */ 00037 // Acquired from Datasheet. 00038 00039 #define HTU21D_I2C_ADDRESS 0x40 00040 #define TRIGGER_TEMP_MEASURE 0xE3 00041 #define TRIGGER_HUMD_MEASURE 0xE5 00042 00043 00044 //Commands. 00045 #define HTU21D_EEPROM_WRITE 0x80 00046 #define HTU21D_EEPROM_READ 0x81 00047 00048 00049 /** 00050 * Honeywell HTU21D digital humidity and temperature sensor. 00051 */ 00052 class HTU21D { 00053 00054 public: 00055 00056 /** 00057 * Constructor. 00058 * 00059 * @param sda mbed pin to use for SDA line of I2C interface. 00060 * @param scl mbed pin to use for SCL line of I2C interface. 00061 */ 00062 HTU21D(PinName sda, PinName scl); 00063 00064 00065 //Samples the temperature, input void, outputs an int in celcius. 00066 int sample_ctemp(void); 00067 00068 //Samples the temperature, input void, outputs an int in fahrenheit. 00069 int sample_ftemp(void); 00070 00071 //Samples the temperature, input void, outputs an int in kelvin. 00072 int sample_ktemp(void); 00073 00074 //Samples the humidity, input void, outputs and int. 00075 int sample_humid(void); 00076 00077 00078 00079 private: 00080 00081 I2C* i2c_; 00082 00083 /** 00084 * Write to EEPROM or RAM on the device. 00085 * 00086 * @param EepromOrRam 0x80 -> Writing to EEPROM 00087 * @param address Address to write to. 00088 * @param data Data to write. 00089 */ 00090 void write(int EepromOrRam, int address, int data); 00091 00092 /** 00093 * Read EEPROM or RAM on the device. 00094 * 00095 * @param EepromOrRam 0x81 -> Reading from EEPROM 00096 * @param address Address to read from. 00097 * @return The contents of the memory address. 00098 */ 00099 int read(int EepromOrRam, int address); 00100 00101 }; 00102 00103 #endif /* HTU21D_H */
Generated on Thu Jul 14 2022 21:36:55 by
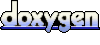