
A blue button is always a nice toy ...
Dependencies: BLE_API X_NUCLEO_IDB0XA1 mbed
Fork of BLE_HeartRate_IDB0XA1 by
main.cpp
00001 // S15_Blue_Button - GATT Detector setup with 00002 // Blue Button connected to Presence characteristic 00003 00004 #include "bricks/bricks.h" 00005 00006 Blinker blink; 00007 00008 //============================================================================== 00009 // GATT Database Setup 00010 //============================================================================== 00011 00012 // Detection Service 00013 00014 Service detection(0xA010,"Detection"); // Detection Service 00015 Characteristic<Bool> chrPresence(detection,0xA011, "n", "Presence"); 00016 00017 // Debug Service 00018 00019 Service debug(0xA030,"Debug"); // Debug Service 00020 Characteristic<Bool> chrTest (debug, 0xA031, "w", "Test"); 00021 00022 static void cbWritten(Blob &o) // handle updates 00023 { 00024 Bool value; 00025 00026 if (updated(o,chrTest)) // has chrTest been updated? 00027 { 00028 get(o,chrTest,value); // get value of chrTest 00029 set(o,chrPresence,value); // and store this value to chrPresence 00030 } 00031 } 00032 00033 void services(O&o) 00034 { 00035 enroll(o,detection); // enroll detection service 00036 enroll(o,debug); // enroll debug service 00037 00038 onWritten(o,cbWritten); // setup 'data written' callback 00039 } 00040 00041 //============================================================================== 00042 // Button Functionality 00043 //============================================================================== 00044 00045 typedef enum state { OFF, ON, IDLE } State; 00046 00047 State state = IDLE; 00048 00049 static void cbRise(void) 00050 { 00051 O o; // declare a blob (BLE OBject) 00052 Bool value = 0; 00053 00054 if (o.hasInitialized()) 00055 { 00056 state = OFF; 00057 set(o,chrPresence,value); // and store this value to chrPresence 00058 } 00059 } 00060 00061 static void cbFall(void) 00062 { 00063 O o; // declare a blob (BLE OBject) 00064 Bool value = 1; 00065 00066 if (o.hasInitialized()) 00067 { 00068 state = ON; 00069 set(o,chrPresence,value); // and store this value to chrPresence 00070 } 00071 } 00072 00073 //============================================================================== 00074 // Callbacks 00075 //============================================================================== 00076 00077 void cbError(O&o) // Error Reporting Callback 00078 { 00079 blink.error(); // 'error' blink sequence 00080 } 00081 00082 void cbConnect(O&o) // Connection Callback 00083 { 00084 blink.connected(); // 'error' blink sequence 00085 } 00086 00087 void cbDisconnect(O&o) // Disconnection Callback 00088 { 00089 advertise(o); // start advertising on client disconnect 00090 blink.advertise(); // 'advertise' blink sequence 00091 } 00092 00093 void cbSetup(O&o) // Immediately After Initializing BLE 00094 { 00095 services(o); // enroll all services & setup callbacks 00096 00097 onConnect(o,cbConnect); // setup connection callback 00098 onDisconnect(o,cbDisconnect); // setup disconnection callback 00099 00100 device(o,"S15#2.0 Blue"); // setup device name 00101 name(o,"Detector"); // setup advertising name 00102 data(o,"My Layout"); // setup advertising data 00103 00104 advertise(o,"C:ng",100); // start advertising @ 100 msec interval 00105 blink.advertise(); // 'advertise' blink sequence 00106 } 00107 00108 //============================================================================== 00109 // Main Program 00110 //============================================================================== 00111 00112 int main(void) 00113 { 00114 O o; // declare a blob (BLE OBject) 00115 verbose(o); // enable all trace messages 00116 blink.idle(); // idle blinking - just started! 00117 00118 InterruptIn button(USER_BUTTON); // declare blue user button 00119 button.rise(&cbRise); // interrupt callback setup 00120 button.fall(&cbFall); // interrupt callback setup 00121 00122 init(o,cbSetup,cbError); // init BLE base layer, always do first 00123 00124 while (true) // Infinite loop waiting for BLE events 00125 { 00126 sleep(o); // low power waiting for BLE events 00127 00128 if (state == ON) // detection active ? 00129 blink.blink("xxx "); 00130 00131 if (state == OFF) // detection inactive ? 00132 blink.blink("x "); 00133 00134 state = IDLE; // reset to IDLE state 00135 } 00136 }
Generated on Wed Jul 20 2022 11:41:13 by
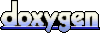