
A blue button is always a nice toy ...
Dependencies: BLE_API X_NUCLEO_IDB0XA1 mbed
Fork of BLE_HeartRate_IDB0XA1 by
get.h
00001 // get.h - get data from a characteristics 00002 // 00003 // Synopsis: 00004 // 00005 // Get data from a 'characteristic' into a 'data' variable 00006 // 00007 // get(characteristic,data) 00008 // 00009 // The 'get' function (overloaded function family) is implemented for all 00010 // types defined in "bricks/types.h". 00011 // 00012 // See also: CHARACTERISTIC, SET 00013 // 00014 #ifndef _GET_H_ 00015 #define _GET_H_ 00016 00017 #include "ble/BLE.h" 00018 #include "ble/Gap.h" 00019 #include "ble/GattClient.h" 00020 #include "bricks/o.h" 00021 #include "bricks/types.h" 00022 #include "bricks/characteristic.h" 00023 00024 inline void get(O&o, Characteristic<Bool> &chr, Bool &data) 00025 { 00026 uint16_t size = sizeof(Bool)/sizeof(uint8_t); 00027 o.gattServer().read(chr.getValueHandle(), (uint8_t*)&data,&size); 00028 } 00029 00030 inline void get(O&o, Characteristic<ObjectId> &chr, ObjectId &data) 00031 { 00032 uint16_t size = sizeof(ObjectId)/sizeof(uint8_t); 00033 o.gattServer().read(chr.getValueHandle(), (uint8_t*)&data,&size); 00034 } 00035 00036 inline void get(O&o, Characteristic<Buffer> &chr, Buffer &data) 00037 { 00038 uint16_t size = sizeof(Buffer)/sizeof(uint8_t); 00039 o.gattServer().read(chr.getValueHandle(), (uint8_t*)&data,&size); 00040 } 00041 00042 // we provide also some GET methods for GattCharacteristics. However the use 00043 // of these methods are more dangerous, because a GattCharacteristics can be 00044 // of any type and the compiler cannot help us to check !!! 00045 00046 inline void get(O&o,GattCharacteristic &chr, Bool &data) 00047 { 00048 uint16_t size = sizeof(Bool)/sizeof(uint8_t); 00049 o.gattServer().read(chr.getValueHandle(), (uint8_t*)&data, &size); 00050 } 00051 00052 #endif // _GET_H_
Generated on Wed Jul 20 2022 11:41:13 by
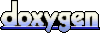