
A blue button is always a nice toy ...
Dependencies: BLE_API X_NUCLEO_IDB0XA1 mbed
Fork of BLE_HeartRate_IDB0XA1 by
collection.h
00001 // collection.h - used to setup a collection of characteristics in order to 00002 // setup a service. 00003 // 00004 // Description: 00005 // Collections are used to setup a service comprising a collection of 00006 // characteristics. The setup sequence starts with declaring a collection 00007 // (this constructs an empty collection) while passing the collection in- 00008 // stance repeatedly to a constructor of a characteristic, which auto-adds 00009 // the new constructed characteristics to the collection. Finally, when the 00010 // collection instance is passed to a service constructor, the service con- 00011 // structor is able to access all characteristics which have been collected 00012 // previously. 00013 // 00014 // Example 1: A protocol might be declared as a class as follows 00015 // 00016 // Collection col; // collection used during setup 00017 // 00018 // Characteristic<ObjectId> id(col,0x2AC3,"rw","ID"); 00019 // Characteristic<ObjectName> name(col,0x2ABE,"rw","Name"); 00020 // Characteristic<Digital> presence(col,0x2A56,"r","Presence"); 00021 // 00022 // Service presenceDetection(col,0xA001); // instantiate service 00023 // 00024 // onSetup(Blob &blue) 00025 // { 00026 // blue.service(presenceDetection); // add service 00027 // } 00028 // 00029 // Example 2: service definition by means of a service definition class 00030 // 00031 // class PresenceDetector 00032 // { 00033 // public: 00034 // Collection col; // collection used during setup 00035 // 00036 // Characteristic<ObjectId> id; // ID of presence detector 00037 // Characteristic<ObjectName> name; // name of presence detector 00038 // Characteristic<Digital> presence; // digital presence value 00039 // Characteristic<DateTime> timestamp; // last detection change's time 00040 // Characteristic<ObjectName> layout; // name of model railway layout 00041 // 00042 // Service presenceDetection; // the service 00043 // 00044 // public: 00045 // PresenceDetector(Blob &blue, cost UUID uuid) : 00046 // list; // init service list 00047 // id(list,0x2AC3,"rw","ID"), // instantiate characteristic 00048 // name(list,0x2ABE,"rw","Name"), // instantiate characteristic 00049 // presence(list,0x2A56,"r","Presence"),// instantiate characteristic 00050 // timestamp(list,0x2A08,"r","Timestamp"),// instantiate characteristic 00051 // layout(list,0x2ABE,"rw","Layout"), // instantiate characteristic 00052 // presenceDetection(list,uuid) // instantiate service 00053 // { 00054 // blue.service(presenceDetection); // add service 00055 // } 00056 // }; 00057 // 00058 #ifndef _COLLECTION_H_ 00059 #define _COLLECTION_H_ 00060 00061 #include "ble/BLE.h" 00062 #include "ble/Gap.h" 00063 00064 class Collection 00065 { 00066 private: 00067 typedef GattCharacteristic *GattCharPtr; 00068 00069 public: 00070 GattCharacteristic **plist; 00071 int count; 00072 00073 Collection() // construct empty collection 00074 { 00075 plist = 0; count = 0; // init properties 00076 } 00077 00078 void add(GattCharPtr p) 00079 { 00080 GattCharPtr *pnew = new GattCharPtr[count+1]; 00081 pnew[count] = p; // add new characteristic 00082 00083 if (count > 0) 00084 { for (int i=0; i < count; i++) 00085 pnew[i] = plist[i]; 00086 delete plist; 00087 } 00088 plist = pnew; // update list pointer 00089 count++; 00090 } 00091 }; 00092 00093 #endif // _COLLECTION_H_
Generated on Wed Jul 20 2022 11:41:13 by
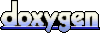