
A blue button is always a nice toy ...
Dependencies: BLE_API X_NUCLEO_IDB0XA1 mbed
Fork of BLE_HeartRate_IDB0XA1 by
blob.h
00001 // blob.h - 'BLOBs' are BLuetooth OBjects 00002 #ifndef _BLOB_H_ 00003 #define _BLOB_H_ 00004 00005 #include "ble/BLE.h" 00006 #include "ble/Gap.h" 00007 00008 #define _ICCC BLE::InitializationCompleteCallbackContext // pure short hand 00009 #define _GDCP Gap::DisconnectionCallbackParams_t // pure short hand 00010 #define _GCCP Gap::ConnectionCallbackParams_t // pure short hand 00011 #define _GWCP GattWriteCallbackParams // pure short hand 00012 00013 class Blob : public BLE 00014 { 00015 public: 00016 const _ICCC *pComplete; // params to _ICCC context 00017 const _GCCP *pConnect; // params to _GCCP context 00018 const _GDCP *pDisconnect; // params to _GDPC context 00019 const _GWCP *pWritten; // params to _GWCP context 00020 00021 public: // construction 00022 Blob() : BLE() // standard constructor 00023 { 00024 pComplete = 0; pDisconnect = 0; 00025 } 00026 }; 00027 00028 00029 // Setup Advertising Name (syntactic sugar) 00030 00031 inline void name(Blob &o, const char *str) 00032 { 00033 o.gap().accumulateAdvertisingPayload( 00034 GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)str, strlen(str)+1); 00035 } 00036 00037 00038 // Setup Device Name (syntactic sugar) 00039 00040 inline void device(Blob &o, const char *text) 00041 { 00042 o.gap().setDeviceName((const uint8_t *)text); 00043 } 00044 00045 00046 // Setup Advertising Data (syntactic sugar) 00047 00048 inline void data(Blob &o, const uint8_t *buf, size_t buflen) 00049 { 00050 o.gap().accumulateAdvertisingPayload(GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA, buf, buflen); 00051 } 00052 00053 inline void data(Blob &o, const char *text) 00054 { 00055 size_t len = strlen(text); 00056 data(o,(const uint8_t*)text,len); 00057 } 00058 00059 #endif // _BLOB_H_
Generated on Wed Jul 20 2022 11:41:13 by
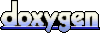