
A blue button is always a nice toy ...
Dependencies: BLE_API X_NUCLEO_IDB0XA1 mbed
Fork of BLE_HeartRate_IDB0XA1 by
blinker.cpp
00001 // blinker.cpp - send a morse pattern to LED1 00002 // 00003 // Function morse() is one way for running LED1 with a blinking sequence using 00004 // a busy wait, until the sequence is completed. 00005 // 00006 // Blinker b; 00007 // b.morse(" x xxx x "); send one time morse sequence, interval = 0.2 00008 // b.morse(" x xxx x ",0.5); send one time morse sequence, interval = 0.5 00009 // 00010 // Function morse(o) got the additional feature to stop an ongoing timer based 00011 // blinking sequence. 00012 // 00013 // The alternative is to setup an ever repeating blink sequence via LED1 using 00014 // function blink(), which is non waiting. 00015 // 00016 // Blinker b; 00017 // b.blink(" x xxx x "); repeating blink sequence, interval = 0.2 00018 // b.blink(" x xxx x ",0.5); repeating blink sequence, interval = 0.5 00019 // b.blink(); stops blinking sequence 00020 // 00021 00022 #include "bricks/target.h" 00023 #include "bricks/blinker.h" 00024 00025 #ifndef LED_INVERTED 00026 # define LED_ON 1 00027 # define LED_OFF 0 00028 #else 00029 # define LED_ON 0 00030 # define LED_OFF 1 00031 #endif 00032 00033 #ifndef BLINK_LED 00034 # define BLINK_LED LED1 00035 #endif 00036 00037 static DigitalOut led(BLINK_LED); // being used for morse sequence 00038 static Ticker ticker; // triggers periodic callbacks 00039 static const char *pointer = 0; // 0 means morse activity disabled 00040 static const char *sequence = 0; // next morse sequence for repeats 00041 00042 void Blinker::morse(const char *pattern, double interval) 00043 { 00044 pointer = 0; // disable ticker based blinking 00045 sequence = 0; // set also empty sequence 00046 00047 for (; *pattern; pattern++) 00048 { 00049 led = (*pattern == ' ') ? LED_OFF : LED_ON; 00050 wait(interval); // busy waiting for interval time 00051 } 00052 } 00053 00054 // callback for LED1 ticker controlled blinking 00055 00056 static void cbBlinker(void) // blinker callback 00057 { 00058 if (pointer != 0) 00059 { 00060 if (*pointer == 0) 00061 { 00062 pointer = sequence; // reset pointer to followup sequence 00063 } 00064 00065 if (*pointer) 00066 { 00067 led = (*pointer++ == ' ') ? LED_OFF : LED_ON; 00068 } 00069 } 00070 } 00071 00072 void Blinker::blink(const char *pattern, const char* next, double interval) 00073 { 00074 pointer = 0; // stop current activities 00075 led = LED_OFF; // reset led with LED_OFF 00076 00077 sequence = next; // init morse sequence 00078 00079 ticker.attach(cbBlinker,interval);// next LED state after every interval 00080 pointer = pattern; // enable callback activty 00081 } 00082 00083 void Blinker::blink(const char *pattern, double interval) 00084 { 00085 blink(pattern,pattern,interval); 00086 } 00087 00088
Generated on Wed Jul 20 2022 11:41:13 by
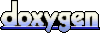