
This is the codebase provided for CIS541 - Homework MQTT.
Embed:
(wiki syntax)
Show/hide line numbers
MQTTmbed.h
00001 // hot fix for NonCopyable error 00002 // @Hung Nguyen 00003 00004 #if !defined(MQTT_MBED_H) 00005 #define MQTT_MBED_H 00006 00007 #include "mbed.h" 00008 00009 class Countdown 00010 { 00011 public: 00012 Countdown() { 00013 t = new Timer(); 00014 } 00015 00016 Countdown(int ms) { 00017 t = new Timer(); 00018 countdown_ms(ms); 00019 } 00020 00021 ~Countdown() { 00022 delete t; 00023 } 00024 00025 00026 bool expired() { 00027 return t->read_ms() >= interval_end_ms; 00028 } 00029 00030 void countdown_ms(unsigned long ms) { 00031 t->stop(); 00032 interval_end_ms = ms; 00033 t->reset(); 00034 t->start(); 00035 } 00036 00037 void countdown(int seconds) { 00038 countdown_ms((unsigned long)seconds * 1000L); 00039 } 00040 00041 int left_ms() { 00042 return interval_end_ms - t->read_ms(); 00043 } 00044 00045 private: 00046 Timer* t; 00047 unsigned long interval_end_ms; 00048 }; 00049 00050 #endif
Generated on Thu Aug 18 2022 19:36:41 by
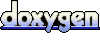