Simple Interface for Toshiba's TB6612FNG H-Bridge Motor Driver
Embed:
(wiki syntax)
Show/hide line numbers
TB6612FNG.h
00001 #ifndef TB6612FNG_H 00002 #define TB6612FNG_H 00003 00004 #include "mbed.h" 00005 00006 /// Simple Interface for Toshiba's TB6612FNG H-Bridge Motor Driver 00007 class TB6612FNG 00008 { 00009 PwmOut m_pwm; 00010 DigitalOut m_ctrl1; 00011 DigitalOut m_ctrl2; 00012 int m_pw; // PWM pulse width setting 00013 bool m_on; // motor satus 00014 bool m_brakeOnZeroDC; // behaviour for zero duty cycle 00015 int m_period; // PWM period setting 00016 00017 public: 00018 /** Create TB6612FNG object connected to the specified mbed pins 00019 * @param pwm PwmOut pin name 00020 * @param ctrl1/ctrl2 DigitalOut control pin name 00021 * @param pwmPeriod sets the PWM period (µs) 00022 * @param brakeOnZeroDC true: The motor brakes on zero pulse width setting. 00023 * false: The motor output is set to high impedance 00024 */ 00025 TB6612FNG(PinName pwm, PinName ctrl1, PinName ctrl2, int pwmPeriod=100, bool brakeOnZeroDC=true); 00026 00027 /// Sets the PWM pulse width 00028 /// @param pw is the PWM pulse width setting in µs. 00029 /// The sign of the given pulsewidth defines the direction. 00030 /// With the default PWM period of 100µs (f=10kHz) this value is equivalent to the duty cycle. 00031 void setPulseWidth(int pw); 00032 00033 /// activates the motor at the duty cycle selected with setPulseWidth() 00034 void on(); 00035 00036 /// deactivates the motor (sets the motor output to high impedance, no short brake) 00037 void off(); 00038 00039 /// brakes the motor by shorting it 00040 void brake(); 00041 00042 /// set behaviour for zero DC setting 00043 /// @param brakeOnZeroDC true: The motor brakes on zero pulse width setting. 00044 /// false: The motor output is set to high impedance 00045 void setZeroDCReaction(bool brakeOnZeroDC); 00046 00047 /// pulse width assignment 00048 /// @param pw is the PWM pulse width setting in µs 00049 /// @see setPulseWidth(int pw) 00050 void operator=(int pw) { 00051 setPulseWidth(pw); 00052 } 00053 }; 00054 00055 #endif
Generated on Wed Jul 13 2022 12:40:45 by
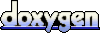