mbed support for STM32F103C8T6 (Blue Pill) boards
Dependents: STM32F103C8T6_nRF24L01P_Hello_World Serial_over_Ethernet STM32F103C8T6_LoRaWAN-lmic-app STM32F103C8T6_WebUSBDFU ... more
SysClockConf.cpp
00001 /* 00002 ****************************************************************************** 00003 * @file SysClockConf.c 00004 * @author Zoltan Hudak 00005 * @version 00006 * @date 05-July-2016 00007 * @brief System Clock configuration for STM32F103C8T6 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 Zoltan Hudak <hudakz@outlook.com> 00012 * 00013 * All rights reserved. 00014 00015 This program is free software: you can redistribute it and/or modify 00016 it under the terms of the GNU General Public License as published by 00017 the Free Software Foundation, either version 3 of the License, or 00018 (at your option) any later version. 00019 00020 This program is distributed in the hope that it will be useful, 00021 but WITHOUT ANY WARRANTY; without even the implied warranty of 00022 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00023 GNU General Public License for more details. 00024 00025 You should have received a copy of the GNU General Public License 00026 along with this program. If not, see <http://www.gnu.org/licenses/>. 00027 */ 00028 00029 #include "SysClockConf.h" 00030 #include "mbed.h" 00031 00032 void HSE_SystemClock_Config(void) { 00033 RCC_OscInitTypeDef RCC_OscInitStruct; 00034 RCC_ClkInitTypeDef RCC_ClkInitStruct; 00035 RCC_PeriphCLKInitTypeDef PeriphClkInit; 00036 00037 RCC_OscInitStruct.OscillatorType = RCC_OSCILLATORTYPE_HSE; 00038 RCC_OscInitStruct.HSEState = RCC_HSE_ON; 00039 RCC_OscInitStruct.HSEPredivValue = RCC_HSE_PREDIV_DIV1; 00040 RCC_OscInitStruct.PLL.PLLState = RCC_PLL_ON; 00041 RCC_OscInitStruct.PLL.PLLSource = RCC_PLLSOURCE_HSE; 00042 RCC_OscInitStruct.PLL.PLLMUL = RCC_PLL_MUL9; 00043 HAL_RCC_OscConfig(&RCC_OscInitStruct); 00044 RCC_ClkInitStruct.ClockType = RCC_CLOCKTYPE_HCLK|RCC_CLOCKTYPE_SYSCLK|RCC_CLOCKTYPE_PCLK1|RCC_CLOCKTYPE_PCLK2; 00045 RCC_ClkInitStruct.SYSCLKSource = RCC_SYSCLKSOURCE_PLLCLK; 00046 RCC_ClkInitStruct.AHBCLKDivider = RCC_SYSCLK_DIV1; 00047 RCC_ClkInitStruct.APB1CLKDivider = RCC_HCLK_DIV2; 00048 RCC_ClkInitStruct.APB2CLKDivider = RCC_HCLK_DIV1; 00049 HAL_RCC_ClockConfig(&RCC_ClkInitStruct, FLASH_LATENCY_2); 00050 PeriphClkInit.PeriphClockSelection = RCC_PERIPHCLK_ADC|RCC_PERIPHCLK_USB; 00051 PeriphClkInit.AdcClockSelection = RCC_ADCPCLK2_DIV6; 00052 PeriphClkInit.UsbClockSelection = RCC_USBCLKSOURCE_PLL_DIV1_5; 00053 HAL_RCCEx_PeriphCLKConfig(&PeriphClkInit); 00054 } 00055 00056 void confSysClock(void) { 00057 HAL_RCC_DeInit(); 00058 HSE_SystemClock_Config(); 00059 SystemCoreClockUpdate(); 00060 }
Generated on Thu Jul 14 2022 18:46:55 by
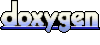