Mbed library for ENC28J60 Ethernet modules. Full support for TCP/IP and UDP Server, Client and HTTP server (webserver). DHCP and DNS is included.
Dependents: mBuino_ENC28_MQTT Nucleo_Web_ENC28J60 Nucleo_Web_ENC28J60_ADC Serial_over_Ethernet ... more
uip_debug.cpp
00001 //#define UIPDEBUG 00002 00003 #if defined(UIPDEBUG) 00004 #include <inttypes.h> 00005 #include "mbed.h" 00006 #include "utility/uip_debug.h" 00007 extern "C" 00008 { 00009 #include "uip.h" 00010 } 00011 struct uip_conn con[UIP_CONNS]; 00012 00013 /** 00014 * @brief 00015 * @note 00016 * @param 00017 * @retval 00018 */ 00019 void UIPDebug::uip_debug_printconns(void) { 00020 for (uint8_t i = 0; i < UIP_CONNS; i++) { 00021 if (uip_debug_printcon(&con[i], &uip_conns[i])) { 00022 printf("connection["); 00023 printf("%d", i); 00024 printf("] changed.\r\n"); 00025 } 00026 } 00027 } 00028 00029 /** 00030 * @brief 00031 * @note 00032 * @param 00033 * @retval 00034 */ 00035 bool UIPDebug::uip_debug_printcon(struct uip_conn* lhs, struct uip_conn* rhs) { 00036 bool changed = false; 00037 if (!uip_ipaddr_cmp(lhs->ripaddr, rhs->ripaddr)) { 00038 printf(" ripaddr: "); 00039 uip_debug_printbytes((const uint8_t*)lhs->ripaddr, 4); 00040 printf(" -> "); 00041 uip_debug_printbytes((const uint8_t*)rhs->ripaddr, 4); 00042 printf("\r\n"); 00043 uip_ipaddr_copy(lhs->ripaddr, rhs->ripaddr); 00044 changed = true; 00045 } 00046 00047 if (lhs->lport != rhs->lport) { 00048 printf(" lport: "); 00049 printf("%d", htons(lhs->lport)); 00050 printf(" -> "); 00051 printf("%d\r\n", htons(rhs->lport)); 00052 lhs->lport = rhs->lport; 00053 changed = true; 00054 } 00055 00056 if (lhs->rport != rhs->rport) { 00057 printf(" rport: "); 00058 printf("%d", htons(lhs->rport)); 00059 printf(" -> "); 00060 printf("%d\r\n", htons(rhs->rport)); 00061 lhs->rport = rhs->rport; 00062 changed = true; 00063 } 00064 00065 if ((uint32_t) lhs->rcv_nxt[0] != (uint32_t) rhs->rcv_nxt[0]) { 00066 printf(" rcv_nxt: "); 00067 uip_debug_printbytes(lhs->rcv_nxt, 4); 00068 printf(" -> "); 00069 uip_debug_printbytes(rhs->rcv_nxt, 4); 00070 *((uint32_t*) &lhs->rcv_nxt[0]) = (uint32_t) rhs->rcv_nxt[0]; 00071 printf("\r\n"); 00072 changed = true; 00073 } 00074 00075 if ((uint32_t) lhs->snd_nxt[0] != (uint32_t) rhs->snd_nxt[0]) { 00076 printf(" snd_nxt: "); 00077 uip_debug_printbytes(lhs->snd_nxt, 4); 00078 printf(" -> "); 00079 uip_debug_printbytes(rhs->snd_nxt, 4); 00080 *((uint32_t*) &lhs->snd_nxt[0]) = (uint32_t) rhs->snd_nxt[0]; 00081 printf("\r\n"); 00082 changed = true; 00083 } 00084 00085 if (lhs->len != rhs->len) { 00086 printf(" len: "); 00087 printf("%d", lhs->len); 00088 printf(" -> "); 00089 printf("%d\r\n", rhs->len); 00090 lhs->len = rhs->len; 00091 changed = true; 00092 } 00093 00094 if (lhs->mss != rhs->mss) { 00095 printf(" mss: "); 00096 printf("%d", lhs->mss); 00097 printf(" -> "); 00098 printf("%d\r\n", rhs->mss); 00099 lhs->mss = rhs->mss; 00100 changed = true; 00101 } 00102 00103 if (lhs->initialmss != rhs->initialmss) { 00104 printf(" initialmss: "); 00105 printf("%d", lhs->initialmss); 00106 printf(" -> "); 00107 printf("%d\r\n", rhs->initialmss); 00108 lhs->initialmss = rhs->initialmss; 00109 changed = true; 00110 } 00111 00112 if (lhs->sa != rhs->sa) { 00113 printf(" sa: "); 00114 printf("%d", lhs->sa); 00115 printf(" -> "); 00116 printf("%d", rhs->sa); 00117 lhs->sa = rhs->sa; 00118 changed = true; 00119 } 00120 00121 if (lhs->sv != rhs->sv) { 00122 printf(" sv: "); 00123 printf("%d", lhs->sv); 00124 printf(" -> "); 00125 printf("%d\r\n", rhs->sv); 00126 lhs->sv = rhs->sv; 00127 changed = true; 00128 } 00129 00130 if (lhs->rto != rhs->rto) { 00131 printf(" rto: "); 00132 printf("%d", lhs->rto); 00133 printf(" -> "); 00134 printf("%d\r\n", rhs->rto); 00135 lhs->rto = rhs->rto; 00136 changed = true; 00137 } 00138 00139 if (lhs->tcpstateflags != rhs->tcpstateflags) { 00140 printf(" tcpstateflags: "); 00141 printf("%d", lhs->tcpstateflags); 00142 printf(" -> "); 00143 printf("%d\r\n", rhs->tcpstateflags); 00144 lhs->tcpstateflags = rhs->tcpstateflags; 00145 changed = true; 00146 } 00147 00148 if (lhs->timer != rhs->timer) { 00149 printf(" timer: "); 00150 printf("%d", lhs->timer); 00151 printf(" -> "); 00152 printf("%d\r\n", rhs->timer); 00153 lhs->timer = rhs->timer; 00154 changed = true; 00155 } 00156 00157 if (lhs->nrtx != rhs->nrtx) { 00158 printf(" nrtx: "); 00159 printf("%d", lhs->nrtx); 00160 printf(" -> "); 00161 printf("%d\r\n", rhs->nrtx); 00162 lhs->nrtx = rhs->nrtx; 00163 changed = true; 00164 } 00165 00166 return changed; 00167 } 00168 00169 /** 00170 * @brief 00171 * @note 00172 * @param 00173 * @retval 00174 */ 00175 void UIPDebug::uip_debug_printbytes(const uint8_t* data, uint8_t len) { 00176 for (uint8_t i = 0; i < len; i++) { 00177 printf("%d", data[i]); 00178 if (i < len - 1) 00179 printf(","); 00180 } 00181 } 00182 #endif
Generated on Tue Jul 12 2022 18:48:00 by
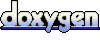