Mbed library for ENC28J60 Ethernet modules. Full support for TCP/IP and UDP Server, Client and HTTP server (webserver). DHCP and DNS is included.
Dependents: mBuino_ENC28_MQTT Nucleo_Web_ENC28J60 Nucleo_Web_ENC28J60_ADC Serial_over_Ethernet ... more
nsapi_types.h
00001 00002 /** \addtogroup netsocket */ 00003 /** @{*/ 00004 /* nsapi.h - The network socket API 00005 * Copyright (c) 2015 ARM Limited 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 */ 00019 00020 #ifndef NSAPI_TYPES_H 00021 #define NSAPI_TYPES_H 00022 00023 #include <stdint.h> 00024 00025 #ifdef __cplusplus 00026 extern "C" { 00027 #endif 00028 00029 00030 /** Enum of standardized error codes 00031 * 00032 * Valid error codes have negative values and may 00033 * be returned by any network operation. 00034 * 00035 * @enum nsapi_error 00036 */ 00037 enum nsapi_error { 00038 NSAPI_ERROR_OK = 0, /*!< no error */ 00039 NSAPI_ERROR_WOULD_BLOCK = -3001, /*!< no data is not available but call is non-blocking */ 00040 NSAPI_ERROR_UNSUPPORTED = -3002, /*!< unsupported functionality */ 00041 NSAPI_ERROR_PARAMETER = -3003, /*!< invalid configuration */ 00042 NSAPI_ERROR_NO_CONNECTION = -3004, /*!< not connected to a network */ 00043 NSAPI_ERROR_NO_SOCKET = -3005, /*!< socket not available for use */ 00044 NSAPI_ERROR_NO_ADDRESS = -3006, /*!< IP address is not known */ 00045 NSAPI_ERROR_NO_MEMORY = -3007, /*!< memory resource not available */ 00046 NSAPI_ERROR_NO_SSID = -3008, /*!< ssid not found */ 00047 NSAPI_ERROR_DNS_FAILURE = -3009, /*!< DNS failed to complete successfully */ 00048 NSAPI_ERROR_DHCP_FAILURE = -3010, /*!< DHCP failed to complete successfully */ 00049 NSAPI_ERROR_AUTH_FAILURE = -3011, /*!< connection to access point failed */ 00050 NSAPI_ERROR_DEVICE_ERROR = -3012, /*!< failure interfacing with the network processor */ 00051 NSAPI_ERROR_IN_PROGRESS = -3013, /*!< operation (eg connect) in progress */ 00052 NSAPI_ERROR_ALREADY = -3014, /*!< operation (eg connect) already in progress */ 00053 NSAPI_ERROR_IS_CONNECTED = -3015, /*!< socket is already connected */ 00054 NSAPI_ERROR_CONNECTION_LOST = -3016, /*!< connection lost */ 00055 NSAPI_ERROR_CONNECTION_TIMEOUT = -3017, /*!< connection timed out */ 00056 NSAPI_ERROR_ADDRESS_IN_USE = -3018, /*!< Address already in use */ 00057 NSAPI_ERROR_TIMEOUT = -3019, /*!< operation timed out */ 00058 NSAPI_ERROR_BUSY = -3020, /*!< device is busy and cannot accept new operation */ 00059 }; 00060 00061 00062 /** Enum of connection status types 00063 * 00064 * Valid error codes have negative values. 00065 * 00066 * @enum nsapi_connection_status 00067 */ 00068 typedef enum nsapi_connection_status { 00069 NSAPI_STATUS_LOCAL_UP = 0, /*!< local IP address set */ 00070 NSAPI_STATUS_GLOBAL_UP = 1, /*!< global IP address set */ 00071 NSAPI_STATUS_DISCONNECTED = 2, /*!< no connection to network */ 00072 NSAPI_STATUS_CONNECTING = 3, /*!< connecting to network */ 00073 NSAPI_STATUS_ERROR_UNSUPPORTED = NSAPI_ERROR_UNSUPPORTED 00074 } nsapi_connection_status_t; 00075 00076 00077 /** Enum of event types 00078 * 00079 * Event callbacks are accompanied with an event-dependent parameter passed as an intptr_t. 00080 * 00081 * @enum nsapi_event 00082 */ 00083 typedef enum nsapi_event { 00084 NSAPI_EVENT_CONNECTION_STATUS_CHANGE = 0, /*!< network connection status has changed, the parameter = new status (nsapi_connection_status_t) */ 00085 NSAPI_EVENT_CELLULAR_STATUS_BASE = 0x1000, /*!< Cellular modem status has changed, See the enum values from enum cellular_connection_status_t in /features/cellular/framework/common/CellularCommon.h */ 00086 NSAPI_EVENT_CELLULAR_STATUS_END = 0x1FFF /*!< cellular modem status has changed, See the enum values from enum cellular_connection_status_t in /features/cellular/framework/common/CellularCommon.h */ 00087 } nsapi_event_t; 00088 00089 00090 /** Type used to represent error codes 00091 * 00092 * This is a separate type from enum nsapi_error to avoid breaking 00093 * compatibility in type-sensitive overloads 00094 */ 00095 typedef signed int nsapi_error_t; 00096 00097 /** Type used to represent the size of data passed through sockets 00098 */ 00099 typedef unsigned int nsapi_size_t; 00100 00101 /** Type used to represent either a size or error passed through sockets 00102 * 00103 * A valid nsapi_size_or_error_t is either a non-negative size or a 00104 * negative error code from the nsapi_error_t 00105 */ 00106 typedef signed int nsapi_size_or_error_t; 00107 00108 /** Type used to represent either a value or error 00109 * 00110 * A valid nsapi_value_or_error_t is either a non-negative value or a 00111 * negative error code from the nsapi_error_t 00112 */ 00113 typedef signed int nsapi_value_or_error_t; 00114 00115 /** Enum of encryption types 00116 * 00117 * The security type specifies a particular security to use when 00118 * connected to a WiFi network 00119 */ 00120 typedef enum nsapi_security { 00121 NSAPI_SECURITY_NONE = 0x0, /*!< open access point */ 00122 NSAPI_SECURITY_WEP = 0x1, /*!< phrase conforms to WEP */ 00123 NSAPI_SECURITY_WPA = 0x2, /*!< phrase conforms to WPA */ 00124 NSAPI_SECURITY_WPA2 = 0x3, /*!< phrase conforms to WPA2 */ 00125 NSAPI_SECURITY_WPA_WPA2 = 0x4, /*!< phrase conforms to WPA/WPA2 */ 00126 NSAPI_SECURITY_PAP = 0x5, /*!< phrase conforms to PPP authentication context */ 00127 NSAPI_SECURITY_CHAP = 0x6, /*!< phrase conforms to PPP authentication context */ 00128 NSAPI_SECURITY_EAP_TLS = 0x7, /*!< phrase conforms to EAP-TLS */ 00129 NSAPI_SECURITY_PEAP = 0x8, /*!< phrase conforms to PEAP */ 00130 NSAPI_SECURITY_UNKNOWN = 0xFF, /*!< unknown/unsupported security in scan results */ 00131 } nsapi_security_t; 00132 00133 /** Size of 2 char network interface name from driver 00134 */ 00135 #define NSAPI_INTERFACE_PREFIX_SIZE 2 00136 00137 /** Maximum size of network interface name 00138 */ 00139 #define NSAPI_INTERFACE_NAME_MAX_SIZE 6 00140 00141 /** Maximum size of IP address representation 00142 */ 00143 #define NSAPI_IP_SIZE NSAPI_IPv6_SIZE 00144 00145 /** Maximum number of bytes for IP address 00146 */ 00147 #define NSAPI_IP_BYTES NSAPI_IPv6_BYTES 00148 00149 /** Maximum size of MAC address representation 00150 */ 00151 #define NSAPI_MAC_SIZE 18 00152 00153 /** Maximum number of bytes for MAC address 00154 */ 00155 #define NSAPI_MAC_BYTES 6 00156 00157 /** Size of IPv4 representation 00158 */ 00159 #define NSAPI_IPv4_SIZE 16 00160 00161 /** Number of bytes in IPv4 address 00162 */ 00163 #define NSAPI_IPv4_BYTES 4 00164 00165 /** Size of IPv6 representation 00166 */ 00167 #define NSAPI_IPv6_SIZE 40 00168 00169 /** Number of bytes in IPv6 address 00170 */ 00171 #define NSAPI_IPv6_BYTES 16 00172 00173 /** Enum of IP address versions 00174 * 00175 * The IP version specifies the type of an IP address. 00176 * 00177 * @enum nsapi_version 00178 */ 00179 typedef enum nsapi_version { 00180 NSAPI_UNSPEC , /*!< Address is unspecified */ 00181 NSAPI_IPv4 , /*!< Address is IPv4 */ 00182 NSAPI_IPv6 , /*!< Address is IPv6 */ 00183 } nsapi_version_t; 00184 00185 /** IP address structure for passing IP addresses by value 00186 */ 00187 typedef struct nsapi_addr { 00188 /** IP version 00189 * - NSAPI_IPv4 00190 * - NSAPI_IPv6 00191 * - NSAPI_UNSPEC 00192 */ 00193 nsapi_version_t version; 00194 00195 /** IP address 00196 * The raw bytes of the IP address stored in big-endian format 00197 */ 00198 uint8_t bytes[NSAPI_IP_BYTES]; 00199 } nsapi_addr_t; 00200 00201 00202 /** Opaque handle for network sockets 00203 */ 00204 typedef void *nsapi_socket_t; 00205 00206 00207 /** Enum of socket protocols 00208 * 00209 * The socket protocol specifies a particular protocol to 00210 * be used with a newly created socket. 00211 * 00212 * @enum nsapi_protocol 00213 */ 00214 typedef enum nsapi_protocol { 00215 NSAPI_TCP , /*!< Socket is of TCP type */ 00216 NSAPI_UDP , /*!< Socket is of UDP type */ 00217 } nsapi_protocol_t; 00218 00219 /** Enum of standardized stack option levels 00220 * for use with NetworkStack::setstackopt and getstackopt. 00221 * 00222 * @enum nsapi_stack_level 00223 */ 00224 typedef enum nsapi_stack_level { 00225 NSAPI_STACK = 5000, /*!< Stack option level - see nsapi_stack_option_t for options */ 00226 } nsapi_stack_level_t; 00227 00228 /** Enum of standardized stack option names for level NSAPI_STACK 00229 * of NetworkStack::setstackopt and getstackopt. 00230 * 00231 * These options may not be supported on all stacks, in which 00232 * case NSAPI_ERROR_UNSUPPORTED may be returned. 00233 * 00234 * @enum nsapi_stack_option 00235 */ 00236 typedef enum nsapi_stack_option { 00237 NSAPI_IPV4_MRU , /*!< Sets/gets size of largest IPv4 fragmented datagram to reassemble */ 00238 NSAPI_IPV6_MRU , /*!< Sets/gets size of largest IPv6 fragmented datagram to reassemble */ 00239 } nsapi_stack_option_t; 00240 00241 /** Enum of standardized socket option levels 00242 * for use with Socket::setsockopt and getsockopt. 00243 * 00244 * @enum nsapi_socket_level 00245 */ 00246 typedef enum nsapi_socket_level { 00247 NSAPI_SOCKET = 7000, /*!< Socket option level - see nsapi_socket_option_t for options */ 00248 } nsapi_socket_level_t; 00249 00250 /** Enum of standardized socket option names for level NSAPI_SOCKET 00251 * of Socket::setsockopt and getsockopt. 00252 * 00253 * These options may not be supported on all stacks, in which 00254 * case NSAPI_ERROR_UNSUPPORTED may be returned. 00255 * 00256 * @enum nsapi_socket_option 00257 */ 00258 typedef enum nsapi_socket_option { 00259 NSAPI_REUSEADDR , /*!< Allow bind to reuse local addresses */ 00260 NSAPI_KEEPALIVE , /*!< Enables sending of keepalive messages */ 00261 NSAPI_KEEPIDLE , /*!< Sets timeout value to initiate keepalive */ 00262 NSAPI_KEEPINTVL , /*!< Sets timeout value for keepalive */ 00263 NSAPI_LINGER , /*!< Keeps close from returning until queues empty */ 00264 NSAPI_SNDBUF , /*!< Sets send buffer size */ 00265 NSAPI_RCVBUF , /*!< Sets recv buffer size */ 00266 NSAPI_ADD_MEMBERSHIP , /*!< Add membership to multicast address */ 00267 NSAPI_DROP_MEMBERSHIP , /*!< Drop membership to multicast address */ 00268 NSAPI_BIND_TO_DEVICE , /*!< Bind socket network interface name*/ 00269 } nsapi_socket_option_t; 00270 00271 /** Supported IP protocol versions of IP stack 00272 * 00273 * @enum nsapi_ip_stack 00274 */ 00275 typedef enum nsapi_ip_stack { 00276 DEFAULT_STACK = 0, 00277 IPV4_STACK, 00278 IPV6_STACK, 00279 IPV4V6_STACK 00280 } nsapi_ip_stack_t; 00281 00282 /* Backwards compatibility - previously didn't distinguish stack and socket options */ 00283 typedef nsapi_socket_level_t nsapi_level_t; 00284 typedef nsapi_socket_option_t nsapi_option_t; 00285 00286 /** nsapi_wifi_ap structure 00287 * 00288 * Structure representing a WiFi Access Point 00289 */ 00290 typedef struct nsapi_wifi_ap { 00291 char ssid[33]; /* 32 is what 802.11 defines as longest possible name; +1 for the \0 */ 00292 uint8_t bssid[6]; 00293 nsapi_security_t security; 00294 int8_t rssi; 00295 uint8_t channel; 00296 } nsapi_wifi_ap_t; 00297 00298 00299 /** nsapi_stack structure 00300 * 00301 * Stack structure representing a specific instance of a stack. 00302 */ 00303 typedef struct nsapi_stack { 00304 /** Network stack operation table 00305 * 00306 * Provides access to the underlying api of the stack. This is not 00307 * flattened into the nsapi_stack to allow allocation in read-only 00308 * memory. 00309 */ 00310 const struct nsapi_stack_api *stack_api; 00311 00312 /** Opaque handle for network stacks 00313 */ 00314 void *stack; 00315 00316 // Internal nsapi buffer 00317 unsigned _stack_buffer[16]; 00318 } nsapi_stack_t; 00319 00320 /** nsapi_ip_mreq structure 00321 */ 00322 typedef struct nsapi_ip_mreq { 00323 nsapi_addr_t imr_multiaddr; /* IP multicast address of group */ 00324 nsapi_addr_t imr_interface; /* local IP address of interface */ 00325 } nsapi_ip_mreq_t; 00326 00327 /** nsapi_stack_api structure 00328 * 00329 * Common api structure for network stack operations. A network stack 00330 * can provide a nsapi_stack_api structure filled out with the 00331 * appropriate implementation. 00332 * 00333 * Unsupported operations can be left as null pointers. 00334 */ 00335 typedef struct nsapi_stack_api { 00336 /** Get the local IP address 00337 * 00338 * @param stack Stack handle 00339 * @return Local IP Address or null address if not connected 00340 */ 00341 nsapi_addr_t (*get_ip_address)(nsapi_stack_t *stack); 00342 00343 /** Translates a hostname to an IP address 00344 * 00345 * The hostname may be either a domain name or an IP address. If the 00346 * hostname is an IP address, no network transactions will be performed. 00347 * 00348 * If no stack-specific DNS resolution is provided, the hostname 00349 * will be resolve using a UDP socket on the stack. 00350 * 00351 * @param stack Stack handle 00352 * @param addr Destination for the host IP address 00353 * @param host Hostname to resolve 00354 * @param version Address family 00355 * @return 0 on success, negative error code on failure 00356 */ 00357 nsapi_error_t (*gethostbyname)(nsapi_stack_t *stack, const char *host, nsapi_addr_t *addr, nsapi_version_t version); 00358 00359 /** Add a domain name server to list of servers to query 00360 * 00361 * @param addr Destination for the host address 00362 * @return 0 on success, negative error code on failure 00363 */ 00364 nsapi_error_t (*add_dns_server)(nsapi_stack_t *stack, nsapi_addr_t addr); 00365 00366 /** Set stack-specific stack options 00367 * 00368 * The setstackopt allow an application to pass stack-specific hints 00369 * to the underlying stack. For unsupported options, 00370 * NSAPI_ERROR_UNSUPPORTED is returned and the stack is unmodified. 00371 * 00372 * @param stack Stack handle 00373 * @param level Stack-specific protocol level 00374 * @param optname Stack-specific option identifier 00375 * @param optval Option value 00376 * @param optlen Length of the option value 00377 * @return 0 on success, negative error code on failure 00378 */ 00379 nsapi_error_t (*setstackopt)(nsapi_stack_t *stack, int level, 00380 int optname, const void *optval, unsigned optlen); 00381 00382 /** Get stack-specific stack options 00383 * 00384 * The getstackopt allow an application to retrieve stack-specific hints 00385 * from the underlying stack. For unsupported options, 00386 * NSAPI_ERROR_UNSUPPORTED is returned and optval is unmodified. 00387 * 00388 * @param stack Stack handle 00389 * @param level Stack-specific protocol level 00390 * @param optname Stack-specific option identifier 00391 * @param optval Destination for option value 00392 * @param optlen Length of the option value 00393 * @return 0 on success, negative error code on failure 00394 */ 00395 nsapi_error_t (*getstackopt)(nsapi_stack_t *stack, int level, 00396 int optname, void *optval, unsigned *optlen); 00397 00398 /** Opens a socket 00399 * 00400 * Creates a network socket and stores it in the specified handle. 00401 * The handle must be passed to following calls on the socket. 00402 * 00403 * A stack may have a finite number of sockets, in this case 00404 * NSAPI_ERROR_NO_SOCKET is returned if no socket is available. 00405 * 00406 * @param stack Stack context 00407 * @param socket Destination for the handle to a newly created socket 00408 * @param proto Protocol of socket to open, NSAPI_TCP or NSAPI_UDP 00409 * @return 0 on success, negative error code on failure 00410 */ 00411 nsapi_error_t (*socket_open)(nsapi_stack_t *stack, nsapi_socket_t *socket, 00412 nsapi_protocol_t proto); 00413 00414 /** Close the socket 00415 * 00416 * Closes any open connection and deallocates any memory associated 00417 * with the socket. 00418 * 00419 * @param stack Stack handle 00420 * @param socket Socket handle 00421 * @return 0 on success, negative error code on failure 00422 */ 00423 nsapi_error_t (*socket_close)(nsapi_stack_t *stack, nsapi_socket_t socket); 00424 00425 /** Bind a specific address to a socket 00426 * 00427 * Binding a socket specifies the address and port on which to receive 00428 * data. If the IP address is zeroed, only the port is bound. 00429 * 00430 * @param stack Stack handle 00431 * @param socket Socket handle 00432 * @param addr Local address to bind, may be null 00433 * @param port Local port to bind 00434 * @return 0 on success, negative error code on failure. 00435 */ 00436 nsapi_error_t (*socket_bind)(nsapi_stack_t *stack, nsapi_socket_t socket, 00437 nsapi_addr_t addr, uint16_t port); 00438 00439 /** Listen for connections on a TCP socket 00440 * 00441 * Marks the socket as a passive socket that can be used to accept 00442 * incoming connections. 00443 * 00444 * @param stack Stack handle 00445 * @param socket Socket handle 00446 * @param backlog Number of pending connections that can be queued 00447 * simultaneously 00448 * @return 0 on success, negative error code on failure 00449 */ 00450 nsapi_error_t (*socket_listen)(nsapi_stack_t *stack, nsapi_socket_t socket, int backlog); 00451 00452 /** Connects TCP socket to a remote host 00453 * 00454 * Initiates a connection to a remote server specified by the 00455 * indicated address. 00456 * 00457 * @param stack Stack handle 00458 * @param socket Socket handle 00459 * @param addr The address of the remote host 00460 * @param port The port of the remote host 00461 * @return 0 on success, negative error code on failure 00462 */ 00463 nsapi_error_t (*socket_connect)(nsapi_stack_t *stack, nsapi_socket_t socket, 00464 nsapi_addr_t addr, uint16_t port); 00465 00466 /** Accepts a connection on a TCP socket 00467 * 00468 * The server socket must be bound and set to listen for connections. 00469 * On a new connection, creates a network socket and stores it in the 00470 * specified handle. The handle must be passed to following calls on 00471 * the socket. 00472 * 00473 * A stack may have a finite number of sockets, in this case 00474 * NSAPI_ERROR_NO_SOCKET is returned if no socket is available. 00475 * 00476 * This call is non-blocking. If accept would block, 00477 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00478 * 00479 * @param stack Stack handle 00480 * @param server Socket handle to server to accept from 00481 * @param socket Destination for a handle to the newly created socket 00482 * @param addr Destination for the address of the remote host 00483 * @param port Destination for the port of the remote host 00484 * @return 0 on success, negative error code on failure 00485 */ 00486 nsapi_error_t (*socket_accept)(nsapi_stack_t *stack, nsapi_socket_t server, 00487 nsapi_socket_t *socket, nsapi_addr_t *addr, uint16_t *port); 00488 00489 /** Send data over a TCP socket 00490 * 00491 * The socket must be connected to a remote host. Returns the number of 00492 * bytes sent from the buffer. 00493 * 00494 * This call is non-blocking. If send would block, 00495 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00496 * 00497 * @param stack Stack handle 00498 * @param socket Socket handle 00499 * @param data Buffer of data to send to the host 00500 * @param size Size of the buffer in bytes 00501 * @return Number of sent bytes on success, negative error 00502 * code on failure 00503 */ 00504 nsapi_size_or_error_t (*socket_send)(nsapi_stack_t *stack, nsapi_socket_t socket, 00505 const void *data, nsapi_size_t size); 00506 00507 /** Receive data over a TCP socket 00508 * 00509 * The socket must be connected to a remote host. Returns the number of 00510 * bytes received into the buffer. 00511 * 00512 * This call is non-blocking. If recv would block, 00513 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00514 * 00515 * @param stack Stack handle 00516 * @param socket Socket handle 00517 * @param data Destination buffer for data received from the host 00518 * @param size Size of the buffer in bytes 00519 * @return Number of received bytes on success, negative error 00520 * code on failure 00521 */ 00522 nsapi_size_or_error_t (*socket_recv)(nsapi_stack_t *stack, nsapi_socket_t socket, 00523 void *data, nsapi_size_t size); 00524 00525 /** Send a packet over a UDP socket 00526 * 00527 * Sends data to the specified address. Returns the number of bytes 00528 * sent from the buffer. 00529 * 00530 * This call is non-blocking. If sendto would block, 00531 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00532 * 00533 * @param stack Stack handle 00534 * @param socket Socket handle 00535 * @param addr The address of the remote host 00536 * @param port The port of the remote host 00537 * @param data Buffer of data to send to the host 00538 * @param size Size of the buffer in bytes 00539 * @return Number of sent bytes on success, negative error 00540 * code on failure 00541 */ 00542 nsapi_size_or_error_t (*socket_sendto)(nsapi_stack_t *stack, nsapi_socket_t socket, 00543 nsapi_addr_t addr, uint16_t port, const void *data, nsapi_size_t size); 00544 00545 /** Receive a packet over a UDP socket 00546 * 00547 * Receives data and stores the source address in address if address 00548 * is not NULL. Returns the number of bytes received into the buffer. 00549 * 00550 * This call is non-blocking. If recvfrom would block, 00551 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00552 * 00553 * @param stack Stack handle 00554 * @param socket Socket handle 00555 * @param addr Destination for the address of the remote host 00556 * @param port Destination for the port of the remote host 00557 * @param data Destination buffer for data received from the host 00558 * @param size Size of the buffer in bytes 00559 * @return Number of received bytes on success, negative error 00560 * code on failure 00561 */ 00562 nsapi_size_or_error_t (*socket_recvfrom)(nsapi_stack_t *stack, nsapi_socket_t socket, 00563 nsapi_addr_t *addr, uint16_t *port, void *buffer, nsapi_size_t size); 00564 00565 /** Register a callback on state change of the socket 00566 * 00567 * The specified callback will be called on state changes such as when 00568 * the socket can recv/send/accept successfully and on when an error 00569 * occurs. The callback may also be called spuriously without reason. 00570 * 00571 * The callback may be called in an interrupt context and should not 00572 * perform expensive operations such as recv/send calls. 00573 * 00574 * @param stack Stack handle 00575 * @param socket Socket handle 00576 * @param callback Function to call on state change 00577 * @param data Argument to pass to callback 00578 */ 00579 void (*socket_attach)(nsapi_stack_t *stack, nsapi_socket_t socket, 00580 void (*callback)(void *), void *data); 00581 00582 /** Set stack-specific socket options 00583 * 00584 * The setsockopt allow an application to pass stack-specific hints 00585 * to the underlying stack. For unsupported options, 00586 * NSAPI_ERROR_UNSUPPORTED is returned and the socket is unmodified. 00587 * 00588 * @param stack Stack handle 00589 * @param socket Socket handle 00590 * @param level Stack-specific protocol level 00591 * @param optname Stack-specific option identifier 00592 * @param optval Option value 00593 * @param optlen Length of the option value 00594 * @return 0 on success, negative error code on failure 00595 */ 00596 nsapi_error_t (*setsockopt)(nsapi_stack_t *stack, nsapi_socket_t socket, int level, 00597 int optname, const void *optval, unsigned optlen); 00598 00599 /** Get stack-specific socket options 00600 * 00601 * The getstackopt allow an application to retrieve stack-specific hints 00602 * from the underlying stack. For unsupported options, 00603 * NSAPI_ERROR_UNSUPPORTED is returned and optval is unmodified. 00604 * 00605 * @param stack Stack handle 00606 * @param socket Socket handle 00607 * @param level Stack-specific protocol level 00608 * @param optname Stack-specific option identifier 00609 * @param optval Destination for option value 00610 * @param optlen Length of the option value 00611 * @return 0 on success, negative error code on failure 00612 */ 00613 nsapi_error_t (*getsockopt)(nsapi_stack_t *stack, nsapi_socket_t socket, int level, 00614 int optname, void *optval, unsigned *optlen); 00615 } nsapi_stack_api_t; 00616 00617 00618 #ifdef __cplusplus 00619 } 00620 #endif 00621 00622 #endif 00623 00624 /** @}*/
Generated on Tue Jul 12 2022 18:48:00 by
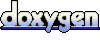