Mbed library for ENC28J60 Ethernet modules. Full support for TCP/IP and UDP Server, Client and HTTP server (webserver). DHCP and DNS is included.
Dependents: mBuino_ENC28_MQTT Nucleo_Web_ENC28J60 Nucleo_Web_ENC28J60_ADC Serial_over_Ethernet ... more
SocketAddress.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 /** @file SocketAddress.h SocketAddress class */ 00018 /** \addtogroup netsocket 00019 * @{*/ 00020 00021 #ifndef SOCKET_ADDRESS_H 00022 #define SOCKET_ADDRESS_H 00023 00024 #include "nsapi_types.h" 00025 #include "mbed_toolchain.h" 00026 00027 // Predeclared classes 00028 class NetworkStack; 00029 class NetworkInterface; 00030 00031 /** SocketAddress class 00032 * 00033 * Representation of an IP address and port pair. 00034 */ 00035 class SocketAddress { 00036 public: 00037 /** Create a SocketAddress from a hostname and port 00038 * 00039 * The hostname may be either a domain name or an IP address. If the 00040 * hostname is an IP address, no network transactions will be performed. 00041 * 00042 * On failure, the IP address and port will be set to zero 00043 * 00044 * @tparam S Type of the Network stack 00045 * @param stack Network stack to use for DNS resolution 00046 * @param host Hostname to resolve 00047 * @param port Optional 16-bit port, defaults to 0 00048 * @deprecated 00049 * Constructors hide possible errors. Replaced by 00050 * NetworkInterface::gethostbyname. 00051 */ 00052 template <typename S> 00053 MBED_DEPRECATED_SINCE("mbed-os-5.1.3", 00054 "Constructors hide possible errors. Replaced by " 00055 "NetworkInterface::gethostbyname.") 00056 SocketAddress(S *stack, const char *host, uint16_t port = 0) 00057 { 00058 _SocketAddress(nsapi_create_stack(stack), host, port); 00059 } 00060 00061 /** Create a SocketAddress from a raw IP address and port 00062 * 00063 * @note To construct from a host name, use NetworkInterface::gethostbyname 00064 * 00065 * @param addr Raw IP address 00066 * @param port Optional 16-bit port, defaults to 0 00067 */ 00068 SocketAddress(nsapi_addr_t addr = nsapi_addr_t(), uint16_t port = 0); 00069 00070 /** Create a SocketAddress from an IP address and port 00071 * 00072 * @param addr Null-terminated representation of the IP address 00073 * @param port Optional 16-bit port, defaults to 0 00074 */ 00075 SocketAddress(const char *addr, uint16_t port = 0); 00076 00077 /** Create a SocketAddress from raw IP bytes, IP version, and port 00078 * 00079 * @param bytes Raw IP address in big-endian order 00080 * @param version IP address version, NSAPI_IPv4 or NSAPI_IPv6 00081 * @param port Optional 16-bit port, defaults to 0 00082 */ 00083 SocketAddress(const void *bytes, nsapi_version_t version, uint16_t port = 0); 00084 00085 /** Create a SocketAddress from another SocketAddress 00086 * 00087 * @param addr SocketAddress to copy 00088 */ 00089 SocketAddress(const SocketAddress &addr); 00090 00091 /** Destructor */ 00092 ~SocketAddress(); 00093 00094 /** Set the IP address 00095 * 00096 * @param addr Null-terminated represention of the IP address 00097 * @return True if address is a valid representation of an IP address, 00098 * otherwise False and SocketAddress is set to null 00099 */ 00100 bool set_ip_address(const char *addr); 00101 00102 /** Set the raw IP bytes and IP version 00103 * 00104 * @param bytes Raw IP address in big-endian order 00105 * @param version IP address version, NSAPI_IPv4 or NSAPI_IPv6 00106 */ 00107 void set_ip_bytes(const void *bytes, nsapi_version_t version); 00108 00109 /** Set the raw IP address 00110 * 00111 * @param addr Raw IP address 00112 */ 00113 void set_addr(nsapi_addr_t addr); 00114 00115 /** Set the port 00116 * 00117 * @param port 16-bit port 00118 */ 00119 void set_port(uint16_t port); 00120 00121 /** Get the human-readable IP address 00122 * 00123 * Allocates memory for a string and converts binary address to 00124 * human-readable format. String is freed in the destructor. 00125 * 00126 * @return Null-terminated representation of the IP Address 00127 */ 00128 const char *get_ip_address() const; 00129 00130 /** Get the raw IP bytes 00131 * 00132 * @return Raw IP address in big-endian order 00133 */ 00134 const void *get_ip_bytes() const; 00135 00136 /** Get the IP address version 00137 * 00138 * @return IP address version, NSAPI_IPv4 or NSAPI_IPv6 00139 */ 00140 nsapi_version_t get_ip_version() const; 00141 00142 /** Get the raw IP address 00143 * 00144 * @return Raw IP address 00145 */ 00146 nsapi_addr_t get_addr() const; 00147 00148 /** Get the port 00149 * 00150 * @return The 16-bit port 00151 */ 00152 uint16_t get_port() const; 00153 00154 /** Test if address is zero 00155 * 00156 * @return True if address is not zero 00157 */ 00158 operator bool() const; 00159 00160 /** Copy address from another SocketAddress 00161 * 00162 * @param addr SocketAddress to copy 00163 */ 00164 SocketAddress &operator=(const SocketAddress &addr); 00165 00166 /** Compare two addresses for equality 00167 * 00168 * @return True if both addresses are equal 00169 */ 00170 friend bool operator==(const SocketAddress &a, const SocketAddress &b); 00171 00172 /** Compare two addresses for equality 00173 * 00174 * @return True if both addresses are not equal 00175 */ 00176 friend bool operator!=(const SocketAddress &a, const SocketAddress &b); 00177 00178 private: 00179 //void _SocketAddress(NetworkStack *iface, const char *host, uint16_t port); 00180 00181 /** Initialize memory */ 00182 void mem_init(void); 00183 00184 mutable char *_ip_address; 00185 nsapi_addr_t _addr; 00186 uint16_t _port; 00187 }; 00188 00189 00190 #endif 00191 00192 /** @}*/
Generated on Tue Jul 12 2022 18:48:00 by
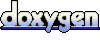