Mbed library for ENC28J60 Ethernet modules. Full support for TCP/IP and UDP Server, Client and HTTP server (webserver). DHCP and DNS is included.
Dependents: mBuino_ENC28_MQTT Nucleo_Web_ENC28J60 Nucleo_Web_ENC28J60_ADC Serial_over_Ethernet ... more
SocketAddress.cpp
00001 /* Socket 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed_version.h" 00018 00019 #if MBED_MAJOR_VERSION == 2 00020 00021 #include "SocketAddress.h" 00022 //#include "NetworkInterface.h" 00023 //#include "NetworkStack.h" 00024 #include <string.h> 00025 #include <stdio.h> 00026 #include "ip4string.h" 00027 #include "ip6string.h" 00028 00029 /** 00030 * @brief 00031 * @note 00032 * @param 00033 * @retval 00034 */ 00035 SocketAddress::SocketAddress(nsapi_addr_t addr, uint16_t port) 00036 { 00037 mem_init(); 00038 _ip_address = NULL; 00039 set_addr(addr); 00040 set_port(port); 00041 } 00042 00043 /** 00044 * @brief 00045 * @note 00046 * @param 00047 * @retval 00048 */ 00049 SocketAddress::SocketAddress(const char* addr, uint16_t port) 00050 { 00051 mem_init(); 00052 _ip_address = NULL; 00053 set_ip_address(addr); 00054 set_port(port); 00055 } 00056 00057 /** 00058 * @brief 00059 * @note 00060 * @param 00061 * @retval 00062 */ 00063 SocketAddress::SocketAddress(const void* bytes, nsapi_version_t version, uint16_t port) 00064 { 00065 mem_init(); 00066 _ip_address = NULL; 00067 set_ip_bytes(bytes, version); 00068 set_port(port); 00069 } 00070 00071 /** 00072 * @brief 00073 * @note 00074 * @param 00075 * @retval 00076 */ 00077 SocketAddress::SocketAddress(const SocketAddress& addr) 00078 { 00079 mem_init(); 00080 _ip_address = NULL; 00081 set_addr(addr.get_addr()); 00082 set_port(addr.get_port()); 00083 } 00084 00085 /** 00086 * @brief 00087 * @note 00088 * @param 00089 * @retval 00090 */ 00091 void SocketAddress::mem_init(void) 00092 { 00093 _addr.version = NSAPI_UNSPEC ; 00094 memset(_addr.bytes, 0, NSAPI_IP_BYTES); 00095 _port = 0; 00096 } 00097 00098 /** 00099 * @brief 00100 * @note 00101 * @param 00102 * @retval 00103 */ 00104 bool SocketAddress::set_ip_address(const char* addr) 00105 { 00106 delete[] _ip_address; 00107 _ip_address = NULL; 00108 00109 if (addr && stoip4(addr, strlen(addr), _addr.bytes)) { 00110 _addr.version = NSAPI_IPv4 ; 00111 return true; 00112 } 00113 else 00114 if (addr && stoip6(addr, strlen(addr), _addr.bytes)) { 00115 _addr.version = NSAPI_IPv6 ; 00116 return true; 00117 } 00118 else { 00119 _addr = nsapi_addr_t(); 00120 return false; 00121 } 00122 } 00123 00124 /** 00125 * @brief 00126 * @note 00127 * @param 00128 * @retval 00129 */ 00130 void SocketAddress::set_ip_bytes(const void* bytes, nsapi_version_t version) 00131 { 00132 nsapi_addr_t addr; 00133 00134 addr = nsapi_addr_t(); 00135 addr.version = version; 00136 if (version == NSAPI_IPv6 ) { 00137 memcpy(addr.bytes, bytes, NSAPI_IPv6_BYTES); 00138 } 00139 else 00140 if (version == NSAPI_IPv4 ) { 00141 memcpy(addr.bytes, bytes, NSAPI_IPv4_BYTES); 00142 } 00143 00144 set_addr(addr); 00145 } 00146 00147 /** 00148 * @brief 00149 * @note 00150 * @param 00151 * @retval 00152 */ 00153 void SocketAddress::set_addr(nsapi_addr_t addr) 00154 { 00155 delete[] _ip_address; 00156 _ip_address = NULL; 00157 _addr = addr; 00158 } 00159 00160 /** 00161 * @brief 00162 * @note 00163 * @param 00164 * @retval 00165 */ 00166 void SocketAddress::set_port(uint16_t port) 00167 { 00168 _port = port; 00169 } 00170 00171 /** 00172 * @brief 00173 * @note 00174 * @param 00175 * @retval 00176 */ 00177 const char* SocketAddress::get_ip_address() const 00178 { 00179 if (_addr.version == NSAPI_UNSPEC ) { 00180 return NULL; 00181 } 00182 00183 if (!_ip_address) { 00184 _ip_address = new char[NSAPI_IP_SIZE]; 00185 if (_addr.version == NSAPI_IPv4 ) { 00186 ip4tos(_addr.bytes, _ip_address); 00187 } 00188 else 00189 if (_addr.version == NSAPI_IPv6 ) { 00190 ip6tos(_addr.bytes, _ip_address); 00191 } 00192 } 00193 00194 return _ip_address; 00195 } 00196 00197 /** 00198 * @brief 00199 * @note 00200 * @param 00201 * @retval 00202 */ 00203 const void* SocketAddress::get_ip_bytes() const 00204 { 00205 return _addr.bytes; 00206 } 00207 00208 /** 00209 * @brief 00210 * @note 00211 * @param 00212 * @retval 00213 */ 00214 nsapi_version_t SocketAddress::get_ip_version() const 00215 { 00216 return _addr.version; 00217 } 00218 00219 /** 00220 * @brief 00221 * @note 00222 * @param 00223 * @retval 00224 */ 00225 nsapi_addr_t SocketAddress::get_addr() const 00226 { 00227 return _addr; 00228 } 00229 00230 /** 00231 * @brief 00232 * @note 00233 * @param 00234 * @retval 00235 */ 00236 uint16_t SocketAddress::get_port() const 00237 { 00238 return _port; 00239 } 00240 00241 /** 00242 * @brief 00243 * @note 00244 * @param 00245 * @retval 00246 */ 00247 SocketAddress::operator bool() const 00248 { 00249 if (_addr.version == NSAPI_IPv4 ) { 00250 for (int i = 0; i < NSAPI_IPv4_BYTES; i++) { 00251 if (_addr.bytes[i]) { 00252 return true; 00253 } 00254 } 00255 00256 return false; 00257 } 00258 else 00259 if (_addr.version == NSAPI_IPv6 ) { 00260 for (int i = 0; i < NSAPI_IPv6_BYTES; i++) { 00261 if (_addr.bytes[i]) { 00262 return true; 00263 } 00264 } 00265 00266 return false; 00267 } 00268 else { 00269 return false; 00270 } 00271 } 00272 00273 /** 00274 * @brief 00275 * @note 00276 * @param 00277 * @retval 00278 */ 00279 SocketAddress &SocketAddress::operator=(const SocketAddress& addr) 00280 { 00281 delete[] _ip_address; 00282 _ip_address = NULL; 00283 set_addr(addr.get_addr()); 00284 set_port(addr.get_port()); 00285 return *this; 00286 } 00287 00288 /** 00289 * @brief 00290 * @note 00291 * @param 00292 * @retval 00293 */ 00294 bool operator==(const SocketAddress& a, const SocketAddress& b) 00295 { 00296 if (!a && !b) { 00297 return true; 00298 } 00299 else 00300 if (a._addr.version != b._addr.version) { 00301 return false; 00302 } 00303 else 00304 if (a._addr.version == NSAPI_IPv4 ) { 00305 return memcmp(a._addr.bytes, b._addr.bytes, NSAPI_IPv4_BYTES) == 0; 00306 } 00307 else 00308 if (a._addr.version == NSAPI_IPv6 ) { 00309 return memcmp(a._addr.bytes, b._addr.bytes, NSAPI_IPv6_BYTES) == 0; 00310 } 00311 00312 MBED_UNREACHABLE; 00313 } 00314 00315 /** 00316 * @brief 00317 * @note 00318 * @param 00319 * @retval 00320 */ 00321 bool operator!=(const SocketAddress& a, const SocketAddress& b) 00322 { 00323 return !(a == b); 00324 } 00325 00326 //void SocketAddress::_SocketAddress(NetworkStack *iface, const char *host, uint16_t port) 00327 //{ 00328 // _ip_address = NULL; 00329 // // gethostbyname must check for literals, so can call it directly 00330 // int err = iface->gethostbyname(host, this); 00331 // _port = port; 00332 // if (err) { 00333 // _addr = nsapi_addr_t(); 00334 // _port = 0; 00335 // } 00336 //} 00337 00338 /** 00339 * @brief 00340 * @note 00341 * @param 00342 * @retval 00343 */ 00344 SocketAddress::~SocketAddress() 00345 { 00346 delete[] _ip_address; 00347 } 00348 00349 #endif
Generated on Tue Jul 12 2022 18:48:00 by
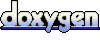