Mbed library for ENC28J60 Ethernet modules. Full support for TCP/IP and UDP Server, Client and HTTP server (webserver). DHCP and DNS is included.
Dependents: mBuino_ENC28_MQTT Nucleo_Web_ENC28J60 Nucleo_Web_ENC28J60_ADC Serial_over_Ethernet ... more
IpAddress.h
00001 /* 00002 IpAddress.h - Base class that provides IPAddress 00003 Copyright (c) 2011 Adrian McEwen. All right reserved. 00004 00005 Modified (ported to mbed) by Zoltan Hudak <hudakz@inbox.com> 00006 00007 This library is free software; you can redistribute it and/or 00008 modify it under the terms of the GNU Lesser General Public 00009 License as published by the Free Software Foundation; either 00010 version 2.1 of the License, or (at your option) any later version. 00011 00012 This library is distributed in the hope that it will be useful, 00013 but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00015 Lesser General Public License for more details. 00016 00017 You should have received a copy of the GNU Lesser General Public 00018 License along with this library; if not, write to the Free Software 00019 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00020 */ 00021 #ifndef IPADDRESS_h 00022 #define IPADDRESS_h 00023 00024 #include <stdio.h> 00025 #include "SocketAddress.h" 00026 00027 // A class to make it easier to handle and pass around IP addresses 00028 00029 class IpAddress 00030 { 00031 private: 00032 uint8_t _address[4]; // IPv4 address 00033 00034 public: 00035 // Constructors 00036 IpAddress(void); 00037 IpAddress(uint8_t octet1, uint8_t octet2, uint8_t octet3, uint8_t octet4); 00038 IpAddress(uint32_t address); 00039 IpAddress(const uint8_t address[4]); 00040 IpAddress(const char *str, size_t len); 00041 00042 // Overloaded cast operator to allow IPAddress objects to be used where a pointer 00043 // to a four-byte uint8_t array is expected 00044 operator uint32_t(void) const { return *((uint32_t*)_address); } 00045 bool operator==(const IpAddress& addr) const { return(*((uint32_t*)_address)) == (*((uint32_t*)addr._address)); } 00046 bool operator==(const uint8_t* addr) const; 00047 00048 // Overloaded index operator to allow getting and setting individual octets of the address 00049 uint8_t operator[](int index) const { return _address[index]; } 00050 uint8_t &operator[](int index) { return _address[index]; } 00051 00052 // Overloaded copy operators to allow initialisation of IPAddress objects from other types 00053 IpAddress &operator =(uint32_t address); 00054 IpAddress &operator =(const uint8_t* address); 00055 00056 // Returns IP Address as string of char 00057 const char* toString(char* buf); 00058 00059 void toSocketAddress(SocketAddress* sockAddr) { sockAddr->set_ip_bytes((const void*)_address, NSAPI_IPv4 ); } 00060 00061 // Access the raw byte array containing the address. Because this returns a pointer 00062 // to the internal structure rather than a copy of the address this function should only 00063 // be used when you know that the usage of the returned uint8_t* will be transient and not 00064 // stored. 00065 uint8_t* rawAddress(void) { return _address; } 00066 00067 friend class UIPEthernet; 00068 friend class UdpSocket; 00069 friend class TcpClient; 00070 friend class TcpServer; 00071 friend class DhcpClient; 00072 friend class DnsClient; 00073 }; 00074 00075 const IpAddress INADDR_NONE(0, 0, 0, 0); 00076 #endif
Generated on Tue Jul 12 2022 18:48:00 by
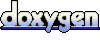