Mbed library for ENC28J60 Ethernet modules. Full support for TCP/IP and UDP Server, Client and HTTP server (webserver). DHCP and DNS is included.
Dependents: mBuino_ENC28_MQTT Nucleo_Web_ENC28J60 Nucleo_Web_ENC28J60_ADC Serial_over_Ethernet ... more
IpAddress.cpp
00001 /* 00002 IPAddress.cpp - Base class that provides IPAddress 00003 Copyright (c) 2011 Adrian McEwen. All right reserved. 00004 00005 Modified (ported to mbed) by Zoltan Hudak <hudakz@inbox.com> 00006 00007 This library is free software; you can redistribute it and/or 00008 modify it under the terms of the GNU Lesser General Public 00009 License as published by the Free Software Foundation; either 00010 version 2.1 of the License, or (at your option) any later version. 00011 00012 This library is distributed in the hope that it will be useful, 00013 but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00015 Lesser General Public License for more details. 00016 00017 You should have received a copy of the GNU Lesser General Public 00018 License along with this library; if not, write to the Free Software 00019 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00020 */ 00021 #include <stdio.h> 00022 #include "mbed.h" 00023 #include "IpAddress.h" 00024 00025 /** 00026 * @brief 00027 * @note 00028 * @param 00029 * @retval 00030 */ 00031 IpAddress::IpAddress(void) 00032 { 00033 memset(_address, 0, sizeof(_address)); 00034 } 00035 00036 /** 00037 * @brief 00038 * @note 00039 * @param 00040 * @retval 00041 */ 00042 IpAddress::IpAddress(uint8_t octet1, uint8_t octet2, uint8_t octet3, uint8_t octet4) 00043 { 00044 _address[0] = octet1; 00045 _address[1] = octet2; 00046 _address[2] = octet3; 00047 _address[3] = octet4; 00048 } 00049 00050 /** 00051 * @brief 00052 * @note 00053 * @param 00054 * @retval 00055 */ 00056 IpAddress::IpAddress(uint32_t address) 00057 { 00058 memcpy(_address, &address, sizeof(_address)); 00059 } 00060 00061 /** 00062 * @brief 00063 * @note 00064 * @param 00065 * @retval 00066 */ 00067 IpAddress::IpAddress(const uint8_t address[4]) 00068 { 00069 memcpy(_address, address, sizeof(_address)); 00070 } 00071 00072 /** 00073 * @brief 00074 * @note 00075 * @param 00076 * @retval 00077 */ 00078 IpAddress::IpAddress(const char* str, size_t len) 00079 { 00080 uint8_t pos = 0; 00081 uint8_t byte; 00082 uint8_t i = 0; 00083 00084 if (len > 16) 00085 return; 00086 00087 while (true) { 00088 if (pos == len || str[pos] < '0' || str[pos] > '9') { 00089 return; 00090 } 00091 00092 byte = 0; 00093 while (pos < len && str[pos] >= '0' && str[pos] <= '9') { 00094 byte *= 10; 00095 byte += str[pos++] - '0'; 00096 } 00097 00098 _address[i++] = byte; 00099 00100 if (i == 4) { 00101 return; 00102 } 00103 00104 if (pos == len || str[pos++] != '.') { 00105 return; 00106 } 00107 } 00108 } 00109 00110 /** 00111 * @brief 00112 * @note 00113 * @param 00114 * @retval 00115 */ 00116 IpAddress &IpAddress::operator=(const uint8_t* address) { 00117 memcpy(_address, address, sizeof(_address)); 00118 return *this; 00119 } 00120 00121 /** 00122 * @brief 00123 * @note 00124 * @param 00125 * @retval 00126 */ 00127 IpAddress &IpAddress::operator=(uint32_t address) 00128 { 00129 memcpy(_address, (const uint8_t*) &address, sizeof(_address)); 00130 return *this; 00131 } 00132 00133 /** 00134 * @brief 00135 * @note 00136 * @param 00137 * @retval 00138 */ 00139 bool IpAddress::operator==(const uint8_t* addr) const 00140 { 00141 return memcmp(addr, _address, sizeof(_address)) == 0; 00142 } 00143 00144 /** 00145 * @brief Returns IP Address as string of char 00146 * @note 00147 * @param 00148 * @retval 00149 */ 00150 const char* IpAddress::toString(char* buf) 00151 { 00152 uint8_t i = 0; 00153 uint8_t j = 0; 00154 00155 for (i = 0; i < 3; i++) { 00156 j += sprintf(&buf[j], "%d", _address[i]); 00157 buf[j++] = '.'; 00158 } 00159 00160 j += sprintf(&buf[j], "%d", _address[i]); 00161 buf[j] = '\0'; 00162 return buf; 00163 }
Generated on Tue Jul 12 2022 18:48:00 by
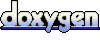