Mbed library for ENC28J60 Ethernet modules. Full support for TCP/IP and UDP Server, Client and HTTP server (webserver). DHCP and DNS is included.
Dependents: mBuino_ENC28_MQTT Nucleo_Web_ENC28J60 Nucleo_Web_ENC28J60_ADC Serial_over_Ethernet ... more
DnsClient.h
00001 // Arduino DNS client for Enc28J60-based Ethernet shield 00002 // (c) Copyright 2009-2010 MCQN Ltd. 00003 // Released under Apache License, version 2.0 00004 #ifndef DNSClient_h 00005 #define DNSClient_h 00006 00007 #include "UdpSocket.h" 00008 #include "IpAddress.h" 00009 00010 class DnsClient 00011 { 00012 public: 00013 // ctor 00014 void begin(const IpAddress& aDNSServer); 00015 00016 /** Convert a numeric IP address string into a four-byte IP address. 00017 @param aIPAddrString IP address to convert 00018 @param aResult IPAddress structure to store the returned IP address 00019 @result 1 if aIPAddrString was successfully converted to an IP address, 00020 else error code 00021 */ 00022 int inet_aton(const char* aIPAddrString, IpAddress& aResult); 00023 00024 /** Resolve the given hostname to an IP address. 00025 @param aHostname Name to be resolved 00026 @param aResult IPAddress structure to store the returned IP address 00027 @result 1 if aIPAddrString was successfully converted to an IP address, 00028 else error code 00029 */ 00030 int getHostByName(const char* aHostname, IpAddress& aResult); 00031 protected: 00032 uint16_t buildRequest(const char* aName); 00033 int16_t processResponse(time_t aTimeout, IpAddress& aAddress); 00034 00035 IpAddress iDNSServer; 00036 uint16_t iRequestId; 00037 UdpSocket iUdp; 00038 }; 00039 #endif
Generated on Tue Jul 12 2022 18:48:00 by
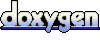