
Simple DS1820 sensor demo showing how to use the DS1820 library [https://developer.mbed.org/users/hudakz/code/DS1820/]
main.cpp
00001 /* 00002 * Simple example program 00003 * 00004 * Note: Don't forget to connect a 4.7k Ohm resistor 00005 * between the DS1820's data pin and the +3.3V pin 00006 * 00007 */ 00008 00009 /* Single DS1820 sensor: */ 00010 /* 00011 #include "mbed.h" 00012 #include "DS1820.h" 00013 00014 Serial pc(USBTX, USBRX); 00015 DigitalOut led(LED1); 00016 DS1820 ds1820(D8); // substitute D8 with the actual pin name connected to the DS1820 sensor 00017 float temp = 0; 00018 int result = 0; 00019 00020 int main() 00021 { 00022 if (ds1820.begin()) { 00023 while (1) { 00024 ds1820.startConversion(); // start temperature conversion from analog to digital 00025 ThisThread::sleep_for(1000);// let DS1820 complete the temperature conversion 00026 result = ds1820.read(temp); // read temperature from DS1820 and perform cyclic redundancy check (CRC) 00027 switch (result) { 00028 case 0: // no errors -> 'temp' contains the value of measured temperature 00029 pc.printf("temp = %3.1f%cC\r\n", temp, 176); 00030 break; 00031 00032 case 1: // no sensor present -> 'temp' was not updated 00033 pc.printf("no sensor present\n\r"); 00034 break; 00035 00036 case 2: // CRC error -> 'temp' was not updated 00037 pc.printf("CRC error\r\n"); 00038 } 00039 00040 led = !led; 00041 } 00042 } 00043 else 00044 pc.printf("No DS1820 sensor found!\r\n"); 00045 } 00046 */ 00047 00048 00049 /*Several DS1820 sensors connected to the 1-wire bus:*/ 00050 #include "mbed.h" 00051 #include "DS1820.h" 00052 00053 #define MAX_SENSOSRS 32 // max number of DS1820 sensors to be connected to the 1-wire bus (max 256) 00054 00055 DS1820* ds1820[MAX_SENSOSRS]; 00056 Serial pc(USBTX, USBRX); 00057 DigitalOut led(LED1); 00058 OneWire oneWire(D8); // substitute D8 with the actual pin name connected to the 1-wire bus 00059 int sensorsFound = 0; // counts the actually found DS1820 sensors 00060 00061 int main() 00062 { 00063 pc.printf("\r\n--Starting--\r\n"); 00064 00065 //Enumerate (i.e. detect) DS1820 sensors on the 1-wire bus 00066 for (sensorsFound = 0; sensorsFound < MAX_SENSOSRS; sensorsFound++) { 00067 ds1820[sensorsFound] = new DS1820(&oneWire); 00068 if (!ds1820[sensorsFound]->begin()) { 00069 delete ds1820[sensorsFound]; 00070 break; 00071 } 00072 } 00073 00074 switch (sensorsFound) { 00075 case 0: 00076 pc.printf("No DS1820 sensor found!\r\n"); 00077 return -1; 00078 00079 case 1: 00080 pc.printf("One DS1820 sensor found.\r\n"); 00081 break; 00082 00083 default: 00084 pc.printf("Found %d DS1820 sensors.\r\n", sensorsFound); 00085 } 00086 00087 while (1) { 00088 pc.printf("----------------\r\n"); 00089 for (int i = 0; i < sensorsFound; i++) 00090 ds1820[i]->startConversion(); // start temperature conversion from analog to digital 00091 ThisThread::sleep_for(1000); // let DS1820 sensors complete the temperature conversion 00092 for (int i = 0; i < sensorsFound; i++) { 00093 if (ds1820[i]->isPresent()) 00094 pc.printf("temp[%d] = %3.1f%cC\r\n", i, ds1820[i]->read(), 176); // read temperature 00095 } 00096 } 00097 }
Generated on Thu Jul 14 2022 17:27:24 by
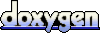