
Arduino_BlinkWithoutDelay_RTC sample code ported. It needs an RTC crystal to be soldered on the board.
Fork of RTC_tst by
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut myled(LED1); // Assign LED1 output port 00004 time_t previousSeconds; 00005 long interval = 1; 00006 00007 void setup() 00008 { 00009 // setup time structure for 26 August 2014 00:00:00 00010 struct tm t; 00011 t.tm_sec = 00; // 0-59 00012 t.tm_min = 00; // 0-59 00013 t.tm_hour = 00; // 0-23 00014 t.tm_mday = 26; // 1-31 00015 t.tm_mon = 8; // 0-11 00016 t.tm_year = 114; // year since 1900 00017 00018 time_t seconds = mktime(&t); 00019 set_time(seconds); 00020 previousSeconds = seconds; 00021 } 00022 00023 void loop() 00024 { 00025 time_t currentSeconds = time(NULL); 00026 if(currentSeconds - previousSeconds > interval) { 00027 previousSeconds = currentSeconds; // save the last time you blinked the LED 00028 myled = !myled; 00029 } 00030 } 00031 00032 int main() 00033 { 00034 setup(); 00035 while(1) loop(); 00036 } 00037 00038
Generated on Fri Jul 22 2022 04:29:15 by
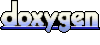