
Demo program for the [[http://mbed.org/users/hlipka/libraries/pop3|pop3 library]].
Dependencies: mbed TcpLineStream NetServicesMin pop3 DNSResolver
main.cpp
00001 #include "EthernetNetIf.h" 00002 00003 #include "mbed.h" 00004 00005 #include "pop3.h" 00006 00007 main() 00008 { 00009 EthernetNetIf eth; 00010 EthernetErr ethErr; 00011 printf("Setting up...\n"); 00012 do { 00013 ethErr = eth.setup(); 00014 if (ethErr) printf("waiting for network...\n", ethErr); 00015 } while (ethErr != ETH_OK); 00016 00017 Pop3 *p3=new Pop3("mail.server","mail.user","mail.password"); 00018 00019 bool r=p3->init(); 00020 00021 printf("init ok=%i\n",r); 00022 00023 if(r) 00024 { 00025 list<string> *ids=p3->getMessages(); 00026 list<string>::iterator it; 00027 for ( it=ids->begin() ; it != ids->end(); it++ ) 00028 { 00029 printf("id=%s\n",(*it).c_str()); 00030 00031 Pop3Message *msg=p3->getMessage(*it); 00032 if (NULL==msg) 00033 continue; 00034 printf("from %s\n",msg->from.c_str()); 00035 printf("subj %s\n",msg->subject.c_str()); 00036 00037 p3->deleteMessage(*it); 00038 00039 delete msg; 00040 00041 } 00042 delete ids; 00043 } 00044 p3->close(); 00045 delete p3; 00046 }
Generated on Fri Jul 15 2022 06:02:33 by
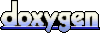