This library takes the current time (which must be set to UTC, e.g. via an NTP call), and applies a timezone definition (loaded at startup) to calculate the local time. This includes the handling of daylight saving. See http://mbed.org/users/hlipka/notebook/time-zone-handling/ for more information (esp. how to get a time zone definition file).
Dependents: CubiScan 000-FIN_youcef 005_ESSAI_youcef
Time.h
00001 /* 00002 * TimeZone library 00003 * Copyright (c) 2010 Hendrik Lipka 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 */ 00023 00024 #ifndef __TIME_H_ 00025 #define __TIME_H_ 00026 00027 #include <time.h> 00028 #include "stdio.h" 00029 #include "string.h" 00030 #include <list> 00031 00032 class Time; 00033 00034 /** 00035 This class encapsulates a point in time - which means it will not change after it has been created. 00036 */ 00037 class TimeStamp { 00038 friend class Time; 00039 public: 00040 /** 00041 @returns the year of the time stamp 00042 */ 00043 int getYear () { 00044 return _year+1900; 00045 }; 00046 00047 /** 00048 @returns the month of the time stamp (January is 1) 00049 */ 00050 int getMonth () { 00051 return _mon+1; 00052 }; 00053 00054 int getDay () { 00055 /** 00056 @returns the day-of-the-month of the time stamp (starting with 1) 00057 */ 00058 return _mday; 00059 }; 00060 00061 /** 00062 @returns the hour of the time stamp (0-23) 00063 */ 00064 int getHour () { 00065 return _hour; 00066 }; 00067 00068 /** 00069 @returns the minute of the time stamp (0-59) 00070 */ 00071 int getMinute () { 00072 return _min; 00073 }; 00074 00075 /** 00076 @returns the second of the time stamp (0-59) 00077 */ 00078 int getSecond () { 00079 return _sec; 00080 }; 00081 00082 /** 00083 get the day of the week - monday is 0 00084 @returns the day-of-the-week of the time stamp 00085 */ 00086 int getDayOfWeek() { 00087 int dow=_wday; 00088 dow--; 00089 if (dow==-1) 00090 dow=6; 00091 return dow; 00092 }; 00093 00094 /** 00095 @returns the day-of-the-year of the time stamp 00096 */ 00097 /* 00098 int getDayOfYear() { 00099 return _yday; 00100 }; 00101 */ 00102 /** 00103 creates a new time stamp based on the UNIX time stamp (seconds since 1970) 00104 */ 00105 TimeStamp(time_t unixTime) { 00106 updateTime(unixTime); 00107 }; 00108 00109 TimeStamp(int year, int mon, int day, int hour, int min, int sec, int wday) 00110 { 00111 _year=(char)(year-1900); 00112 _mon=(char)(mon-1); 00113 _mday=(char)day; 00114 _hour=(char)hour; 00115 _min=(char)min; 00116 _sec=(char)sec; 00117 _wday=(char)wday; 00118 } 00119 ~TimeStamp() { 00120 }; 00121 00122 /** 00123 @param ts the time stamp to compare to 00124 @returns true when both timestamp are the same point in time 00125 */ 00126 bool isSame (TimeStamp* ts); 00127 00128 /** 00129 @param ts the time stamp to compare to 00130 @return true when the current time stamp is before the given time stamp 00131 */ 00132 bool isBefore (TimeStamp* ts); 00133 00134 /** 00135 @returns time stamp transformed to ASCII (done by asctime() - but transformed to local time) 00136 */ 00137 char *asChar () { struct tm * time=getTm();char *s=asctime(time); delete time;return s;}; 00138 00139 /** 00140 @param ts the time stamp to compare to 00141 @return true when the current time stamp is after the given time stamp 00142 */ 00143 bool isAfter (TimeStamp* ts); 00144 int getStartOfDay() 00145 { 00146 tm* t=getTm(); 00147 t->tm_hour=0; 00148 t->tm_min=0; 00149 t->tm_sec=0; 00150 return mktime(t); 00151 } 00152 private: 00153 tm *getTm() 00154 { 00155 tm *time=new tm(); 00156 00157 time->tm_year=_year; 00158 time->tm_mon=_mon; 00159 time->tm_mday=_mday; 00160 time->tm_hour=_hour; 00161 time->tm_min=_min; 00162 time->tm_sec=_sec; 00163 time->tm_wday=_wday; 00164 00165 return time; 00166 } 00167 void updateTime(time_t unixTime) { 00168 struct tm *time; 00169 time=localtime(&unixTime); 00170 _year=time->tm_year; 00171 _mon=time->tm_mon; 00172 _mday=time->tm_mday; 00173 _hour=time->tm_hour; 00174 _min=time->tm_min; 00175 _sec=time->tm_sec; 00176 _wday=time->tm_wday; 00177 } 00178 void print() 00179 { 00180 printf("ts=%i.%i.%i %i:%i.%i\n",_mday,_mon+1,_year+1900,_hour,_min,_sec); 00181 } 00182 // make sure this structure needs only 7 bytes - otherwise it's 48 bytes... 00183 char __packed _year; 00184 char __packed _mon; 00185 char __packed _mday; 00186 char __packed _hour; 00187 char __packed _min; 00188 char __packed _sec; 00189 char __packed _wday; 00190 }; 00191 00192 class TimeZoneEntry; 00193 00194 /** 00195 This class handles the time zone calculation, and is used for creating time stamp objects. 00196 */ 00197 class Time { 00198 public: 00199 /** 00200 creates a new Time instance. On the first call, it reads the file 'timezone.csv' from the USB disk (local file system). 00201 */ 00202 Time(); 00203 ~Time(); 00204 /** 00205 creates a new TimeStamp instance. The caller is responsible for deleting it afterwards! 00206 @returns the time stamp 00207 */ 00208 TimeStamp* getTime(); 00209 /** 00210 @returns the current time stamp (UTC) in UNIX format, without time zone correction. 00211 */ 00212 long getUnixTime (); 00213 private: 00214 static TimeZoneEntry* _timeZoneEntries; 00215 static void readTimeZones(); 00216 static int getTimeOffset(TimeStamp* ts); 00217 }; 00218 00219 #endif
Generated on Thu Jul 14 2022 13:56:12 by
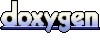