
ESE project: Portable Camera Safe Box
Dependencies: Freetronics_16x2_LCD X_NUCLEO_IKS01A1 mbed
Fork of HelloWorld_IKS01A1 by
main.cpp
00001 /* Includes */ 00002 #include "mbed.h" 00003 #include "x_nucleo_iks01a1.h" 00004 #include "freetronicsLCDShield.h" 00005 00006 freetronicsLCDShield lcd(D12, D11, D10, D9, D8, D7, D3, A0); 00007 00008 00009 00010 /* Instantiate the expansion board */ 00011 static X_NUCLEO_IKS01A1 *mems_expansion_board = X_NUCLEO_IKS01A1::Instance(D14, D15); 00012 00013 /* Retrieve the composing elements of the expansion board */ 00014 static GyroSensor *gyroscope = mems_expansion_board->GetGyroscope(); 00015 static MotionSensor *accelerometer = mems_expansion_board->GetAccelerometer(); 00016 //static MagneticSensor *magnetometer = mems_expansion_board->magnetometer; 00017 static HumiditySensor *humidity_sensor = mems_expansion_board->ht_sensor; 00018 //static PressureSensor *pressure_sensor = mems_expansion_board->pt_sensor; 00019 static TempSensor *temp_sensor1 = mems_expansion_board->ht_sensor; 00020 static TempSensor *temp_sensor2 = mems_expansion_board->pt_sensor; 00021 00022 /* Helper function for printing floats & doubles */ 00023 static char *printDouble(char* str, double v, int decimalDigits=2) 00024 { 00025 int i = 1; 00026 int intPart, fractPart; 00027 int len; 00028 char *ptr; 00029 00030 /* prepare decimal digits multiplicator */ 00031 for (;decimalDigits!=0; i*=10, decimalDigits--); 00032 00033 /* calculate integer & fractinal parts */ 00034 intPart = (int)v; 00035 fractPart = (int)((v-(double)(int)v)*i); 00036 00037 /* fill in integer part */ 00038 sprintf(str, "%i.", intPart); 00039 00040 /* prepare fill in of fractional part */ 00041 len = strlen(str); 00042 ptr = &str[len]; 00043 00044 /* fill in leading fractional zeros */ 00045 for (i/=10;i>1; i/=10, ptr++) { 00046 if(fractPart >= i) break; 00047 *ptr = '0'; 00048 } 00049 00050 /* fill in (rest of) fractional part */ 00051 sprintf(ptr, "%i", fractPart); 00052 00053 return str; 00054 } 00055 00056 00057 /* Simple main function */ 00058 int main() { 00059 uint8_t id; 00060 float value1, value2; 00061 char buffer1[32], buffer2[32]; 00062 int32_t axes[3]; 00063 int32_t m[3]; 00064 int32_t n[3]; 00065 int32_t r; 00066 int32_t i; 00067 int32_t lock_unlock=0; 00068 int temp=0; 00069 00070 // turn on the back light (it's off by default) 00071 lcd.setBackLight(true); 00072 00073 // print the first line and wait 3 sec 00074 lcd.printf("Starting ESEproc"); 00075 wait(2); 00076 00077 00078 printf("\r\n--- Starting ESEproc ---\r\n"); 00079 00080 00081 00082 00083 // print the counter prefix; the number will be printed in the while loop 00084 lcd.setCursorPosition(1, 0); 00085 lcd.printf("Initializing..."); 00086 00087 humidity_sensor->ReadID(&id); 00088 printf("HTS221 humidity & temperature = 0x%X\r\n", id); 00089 //pressure_sensor->ReadID(&id); 00090 // printf("LPS25H pressure & temperature = 0x%X\r\n", id); 00091 //magnetometer->ReadID(&id); 00092 //printf("LIS3MDL magnetometer = 0x%X\r\n", id); 00093 gyroscope->ReadID(&id); 00094 printf("LSM6DS0 accelerometer & gyroscope = 0x%X\r\n", id); 00095 00096 DigitalOut myled(LED1); 00097 DigitalIn mybutton(USER_BUTTON); 00098 00099 wait(2); 00100 00101 // turn off the back light 00102 lcd.setBackLight(false); 00103 00104 while(1) { 00105 wait(0.001); 00106 printf("\r\n"); 00107 00108 temp_sensor1->GetTemperature(&value1); 00109 humidity_sensor->GetHumidity(&value2); 00110 00111 // print the Temperature and Humidity 00112 lcd.cls(); 00113 lcd.setBackLight(false); 00114 lcd.printf("T:%4s C", printDouble(buffer1, value1)); 00115 00116 00117 lcd.setCursorPosition(1, 0); 00118 lcd.printf("H:%s %%", printDouble(buffer2, value2)); 00119 if ((value1>37) && (value2>80)) 00120 { 00121 myled=1; 00122 00123 printf("Excessive Temperature & Excessive Humidity\r\n"); 00124 lcd.setBackLight(true); 00125 lcd.setCursorPosition(0, 12); 00126 lcd.printf("T"); 00127 lcd.setCursorPosition(1, 12); 00128 lcd.printf("H"); 00129 wait(0.1); 00130 myled=0; 00131 } 00132 if ((value1>37) && (value2<=80)) 00133 { 00134 myled=1; 00135 00136 printf("Excessive Temperature\r\n"); 00137 lcd.setBackLight(true); 00138 lcd.setCursorPosition(0, 12); 00139 lcd.printf("T"); 00140 wait(0.4); 00141 myled=0; 00142 } 00143 if ((value1<=37) && (value2>80)) 00144 { 00145 myled=1; 00146 00147 printf("Excessive Humidity\r\n"); 00148 lcd.setBackLight(true); 00149 lcd.setCursorPosition(1, 12); 00150 lcd.printf("H"); 00151 wait(0.8); 00152 myled=0; 00153 } 00154 00155 00156 printf("HTS221: [temp] %7s°C, [hum] %s%%\r\n", printDouble(buffer1, value1), printDouble(buffer2, value2)); 00157 00158 00159 00160 // temp_sensor2->GetFahrenheit(&value1); 00161 //pressure_sensor->GetPressure(&value2); 00162 //printf("LPS25H: [temp] %7s°F, [press] %smbar\r\n", printDouble(buffer1, value1), printDouble(buffer2, value2)); 00163 00164 00165 // if (temp>=100){ 00166 // lock_unlock =! lock_unlock; 00167 // myled=1; 00168 // wait(0.2); 00169 // myled=0; 00170 // wait(0.2); 00171 // myled=1; 00172 // wait(0.2); 00173 // myled=0; 00174 // } 00175 00176 if (lock_unlock == 0) 00177 { 00178 printf("SecuritySystemON\r\n"); 00179 lcd.setCursorPosition(0, 15); 00180 lcd.printf("S"); 00181 accelerometer->Get_X_Axes(axes); 00182 for (i=0;i<3;i++) 00183 { 00184 m[i]=axes[i]; 00185 } 00186 wait(0.2); 00187 accelerometer->Get_X_Axes(axes); 00188 for (i=0;i<3;i++) 00189 { 00190 n[i]=axes[i]; 00191 } 00192 r=(m[0]-n[0])*(m[0]-n[0])+(m[1]-n[1])*(m[1]-n[1])+(m[2]-n[2])*(m[2]-n[2]); 00193 if(r>1500) 00194 { 00195 myled=1; 00196 printf("Security Alert\r\n"); 00197 lcd.cls(); 00198 lcd.setBackLight(true); 00199 lcd.printf("Security Alert"); 00200 lcd.setCursorPosition(1, 0); 00201 lcd.printf("BUZZING..."); 00202 wait(5); 00203 00204 } 00205 printf("LSM6DS0 [acc/mg]:%6ld, %6ld, %6ld\r\n", axes[0], axes[1], axes[2]); 00206 } 00207 if (lock_unlock != 0) 00208 { 00209 printf("UNLOCKED\r\n"); 00210 lcd.setCursorPosition(0, 15); 00211 lcd.printf(" ");//means unlock by removing the s on LCD 00212 printf("SecuritySystemOFF\r\n"); 00213 } 00214 if(mybutton == 0) 00215 { 00216 temp=0; 00217 lcd.setBackLight(true); 00218 while(mybutton==0 and temp<500){ 00219 wait(0.01); 00220 temp++; 00221 } 00222 if (temp==500){ 00223 lock_unlock =! lock_unlock; 00224 if (lock_unlock==0){ 00225 lcd.setCursorPosition(0, 15); 00226 lcd.printf("S");//means lock by adding a s on LCD 00227 } 00228 if (lock_unlock==1){ 00229 lcd.setCursorPosition(0, 15); 00230 lcd.printf(" ");//means unlock by removing the s on LCD 00231 } 00232 } 00233 00234 wait(0.2); 00235 lcd.setBackLight(false); 00236 } 00237 lcd.setBackLight(false); 00238 wait(0.3); 00239 } 00240 }
Generated on Wed Jul 13 2022 02:28:22 by
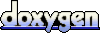