update data rate, jadi 860SPS. Belum ditest
Embed:
(wiki syntax)
Show/hide line numbers
Adafruit_ADS1015.cpp
Go to the documentation of this file.
00001 /**************************************************************************/ 00002 /*! 00003 @file Adafruit_ADS1015.cpp 00004 @author K.Townsend (Adafruit Industries) 00005 @license BSD (see LICENSE.txt) 00006 00007 Ported to mbed by Arve Seljebu - arve0.github.io 00008 00009 Driver for the ADS1015/ADS1115 ADC 00010 00011 This is a library for the Adafruit MPL115A2 breakout 00012 ----> https://www.adafruit.com/products/1083 00013 00014 Adafruit invests time and resources providing this open source code, 00015 please support Adafruit and open-source hardware by purchasing 00016 products from Adafruit! 00017 00018 @section HISTORY 00019 00020 v1.0 - First release 00021 v1.1.1 - Ported to mbed 00022 */ 00023 /**************************************************************************/ 00024 00025 #include <mbed.h> 00026 #include "Adafruit_ADS1015.h " 00027 00028 /**************************************************************************/ 00029 /*! 00030 @brief Writes 16-bits to the specified destination register 00031 */ 00032 /**************************************************************************/ 00033 void Adafruit_ADS1015::writeRegister(uint8_t i2cAddress, uint8_t reg, uint16_t value) 00034 { 00035 char cmd[3]; 00036 cmd[0] = (char)reg; 00037 cmd[1] = (char)(value>>8); 00038 cmd[2] = (char)(value & 0xFF); 00039 m_i2c->write(i2cAddress, cmd, 3); 00040 } 00041 00042 /**************************************************************************/ 00043 /*! 00044 @brief Reads 16-bits from the specified register 00045 */ 00046 /**************************************************************************/ 00047 uint16_t Adafruit_ADS1015::readRegister(uint8_t i2cAddress, uint8_t reg) 00048 { 00049 char data[2]; 00050 data[0] = reg; // temporary use this to send address to conversion register 00051 m_i2c->write(i2cAddress, data, 1, 1); // no stop 00052 m_i2c->read(i2cAddress, data, 2); 00053 return (data[0] << 8 | data [1]); 00054 } 00055 00056 /**************************************************************************/ 00057 /*! 00058 @brief Instantiates a new ADS1015 class w/appropriate properties 00059 */ 00060 /**************************************************************************/ 00061 Adafruit_ADS1015::Adafruit_ADS1015(I2C* i2c, uint8_t i2cAddress) 00062 { 00063 // shift 7 bit address 1 left: read expects 8 bit address, see I2C.h 00064 m_i2cAddress = i2cAddress << 1; 00065 m_conversionDelay = ADS1115_CONVERSIONDELAY_860SPS; 00066 m_bitShift = 4; 00067 m_gain = GAIN_TWOTHIRDS; /* +/- 6.144V range (limited to VDD +0.3V max!) */ 00068 m_i2c = i2c; 00069 } 00070 00071 /**************************************************************************/ 00072 /*! 00073 @brief Instantiates a new ADS1115 class w/appropriate properties 00074 */ 00075 /**************************************************************************/ 00076 Adafruit_ADS1115::Adafruit_ADS1115(I2C* i2c, uint8_t i2cAddress) 00077 { 00078 // shift 7 bit address 1 left: read expects 8 bit address, see mbed's I2C.h 00079 m_i2cAddress = i2cAddress << 1; 00080 m_conversionDelay = ADS1115_CONVERSIONDELAY_860SPS; 00081 m_bitShift = 0; 00082 m_gain = GAIN_TWOTHIRDS; /* +/- 6.144V range (limited to VDD +0.3V max!) */ 00083 m_i2c = i2c; 00084 } 00085 00086 /**************************************************************************/ 00087 /*! 00088 @brief Sets the gain and input voltage range 00089 */ 00090 /**************************************************************************/ 00091 void Adafruit_ADS1015::setGain(adsGain_t gain) 00092 { 00093 m_gain = gain; 00094 } 00095 00096 /**************************************************************************/ 00097 /*! 00098 @brief Gets a gain and input voltage range 00099 */ 00100 /**************************************************************************/ 00101 adsGain_t Adafruit_ADS1015::getGain() 00102 { 00103 return m_gain; 00104 } 00105 00106 /**************************************************************************/ 00107 /*! 00108 @brief Gets a single-ended ADC reading from the specified channel 00109 */ 00110 /**************************************************************************/ 00111 uint16_t Adafruit_ADS1015::readADC_SingleEnded(uint8_t channel) 00112 { 00113 if (channel > 3) { 00114 return 0; 00115 } 00116 00117 // Start with default values 00118 uint16_t config = ADS1015_REG_CONFIG_CQUE_NONE | // Disable the comparator (default val) 00119 ADS1015_REG_CONFIG_CLAT_NONLAT | // Non-latching (default val) 00120 ADS1015_REG_CONFIG_CPOL_ACTVLOW | // Alert/Rdy active low (default val) 00121 ADS1015_REG_CONFIG_CMODE_TRAD | // Traditional comparator (default val) 00122 ADS1115_REG_CONFIG_DR_860SPS | // 860 Samples per Second, ADS1115 00123 ADS1015_REG_CONFIG_MODE_SINGLE; // Single-shot mode (default) 00124 00125 // Set PGA/voltage range 00126 config |= m_gain; 00127 00128 // Set single-ended input channel 00129 switch (channel) { 00130 case (0): 00131 config |= ADS1015_REG_CONFIG_MUX_SINGLE_0; 00132 break; 00133 case (1): 00134 config |= ADS1015_REG_CONFIG_MUX_SINGLE_1; 00135 break; 00136 case (2): 00137 config |= ADS1015_REG_CONFIG_MUX_SINGLE_2; 00138 break; 00139 case (3): 00140 config |= ADS1015_REG_CONFIG_MUX_SINGLE_3; 00141 break; 00142 } 00143 00144 // Set 'start single-conversion' bit 00145 config |= ADS1015_REG_CONFIG_OS_SINGLE; 00146 00147 // Write config register to the ADC 00148 writeRegister(m_i2cAddress, ADS1015_REG_POINTER_CONFIG, config); 00149 00150 // Wait for the conversion to complete 00151 wait_ms(m_conversionDelay); 00152 00153 // Read the conversion results 00154 // Shift 12-bit results right 4 bits for the ADS1015 00155 return readRegister(m_i2cAddress, ADS1015_REG_POINTER_CONVERT); 00156 } 00157 00158 /**************************************************************************/ 00159 /*! 00160 @brief Reads the conversion results, measuring the voltage 00161 difference between the P (AIN0) and N (AIN1) input. Generates 00162 a signed value since the difference can be either 00163 positive or negative. 00164 */ 00165 /**************************************************************************/ 00166 int16_t Adafruit_ADS1015::readADC_Differential_0_1() 00167 { 00168 // Start with default values 00169 uint16_t config = ADS1015_REG_CONFIG_CQUE_NONE | // Disable the comparator (default val) 00170 ADS1015_REG_CONFIG_CLAT_NONLAT | // Non-latching (default val) 00171 ADS1015_REG_CONFIG_CPOL_ACTVLOW | // Alert/Rdy active low (default val) 00172 ADS1015_REG_CONFIG_CMODE_TRAD | // Traditional comparator (default val) 00173 ADS1015_REG_CONFIG_DR_1600SPS | // 1600(ADS1015) or 250(ADS1115) samples per second (default) 00174 ADS1015_REG_CONFIG_MODE_SINGLE; // Single-shot mode (default) 00175 00176 // Set PGA/voltage range 00177 config |= m_gain; 00178 00179 // Set channels 00180 config |= ADS1015_REG_CONFIG_MUX_DIFF_0_1; // AIN0 = P, AIN1 = N 00181 00182 // Set 'start single-conversion' bit 00183 config |= ADS1015_REG_CONFIG_OS_SINGLE; 00184 00185 // Write config register to the ADC 00186 writeRegister(m_i2cAddress, ADS1015_REG_POINTER_CONFIG, config); 00187 00188 // Wait for the conversion to complete 00189 wait_ms(m_conversionDelay); 00190 00191 // Read the conversion results 00192 uint16_t res = readRegister(m_i2cAddress, ADS1015_REG_POINTER_CONVERT) >> m_bitShift; 00193 if (m_bitShift == 0) { 00194 return (int16_t)res; 00195 } else { 00196 // Shift 12-bit results right 4 bits for the ADS1015, 00197 // making sure we keep the sign bit intact 00198 if (res > 0x07FF) { 00199 // negative number - extend the sign to 16th bit 00200 res |= 0xF000; 00201 } 00202 return (int16_t)res; 00203 } 00204 } 00205 00206 /**************************************************************************/ 00207 /*! 00208 @brief Reads the conversion results, measuring the voltage 00209 difference between the P (AIN2) and N (AIN3) input. Generates 00210 a signed value since the difference can be either 00211 positive or negative. 00212 */ 00213 /**************************************************************************/ 00214 int16_t Adafruit_ADS1015::readADC_Differential_2_3() 00215 { 00216 // Start with default values 00217 uint16_t config = ADS1015_REG_CONFIG_CQUE_NONE | // Disable the comparator (default val) 00218 ADS1015_REG_CONFIG_CLAT_NONLAT | // Non-latching (default val) 00219 ADS1015_REG_CONFIG_CPOL_ACTVLOW | // Alert/Rdy active low (default val) 00220 ADS1015_REG_CONFIG_CMODE_TRAD | // Traditional comparator (default val) 00221 ADS1015_REG_CONFIG_DR_1600SPS | // 1600(ADS1015) or 250(ADS1115) samples per second (default) 00222 ADS1015_REG_CONFIG_MODE_SINGLE; // Single-shot mode (default) 00223 00224 // Set PGA/voltage range 00225 config |= m_gain; 00226 00227 // Set channels 00228 config |= ADS1015_REG_CONFIG_MUX_DIFF_2_3; // AIN2 = P, AIN3 = N 00229 00230 // Set 'start single-conversion' bit 00231 config |= ADS1015_REG_CONFIG_OS_SINGLE; 00232 00233 // Write config register to the ADC 00234 writeRegister(m_i2cAddress, ADS1015_REG_POINTER_CONFIG, config); 00235 00236 // Wait for the conversion to complete 00237 wait_ms(m_conversionDelay); 00238 00239 // Read the conversion results 00240 uint16_t res = readRegister(m_i2cAddress, ADS1015_REG_POINTER_CONVERT) >> m_bitShift; 00241 if (m_bitShift == 0) { 00242 return (int16_t)res; 00243 } else { 00244 // Shift 12-bit results right 4 bits for the ADS1015, 00245 // making sure we keep the sign bit intact 00246 if (res > 0x07FF) { 00247 // negative number - extend the sign to 16th bit 00248 res |= 0xF000; 00249 } 00250 return (int16_t)res; 00251 } 00252 } 00253 00254 /**************************************************************************/ 00255 /*! 00256 @brief Sets up the comparator to operate in basic mode, causing the 00257 ALERT/RDY pin to assert (go from high to low) when the ADC 00258 value exceeds the specified threshold. 00259 00260 This will also set the ADC in continuous conversion mode. 00261 */ 00262 /**************************************************************************/ 00263 void Adafruit_ADS1015::startComparator_SingleEnded(uint8_t channel, int16_t threshold) 00264 { 00265 // Start with default values 00266 uint16_t config = ADS1015_REG_CONFIG_CQUE_1CONV | // Comparator enabled and asserts on 1 match 00267 ADS1015_REG_CONFIG_CLAT_LATCH | // Latching mode 00268 ADS1015_REG_CONFIG_CPOL_ACTVLOW | // Alert/Rdy active low (default val) 00269 ADS1015_REG_CONFIG_CMODE_TRAD | // Traditional comparator (default val) 00270 ADS1015_REG_CONFIG_DR_1600SPS | // 1600(ADS1015) or 250(ADS1115) samples per second (default) 00271 ADS1015_REG_CONFIG_MODE_CONTIN | // Continuous conversion mode 00272 ADS1015_REG_CONFIG_MODE_CONTIN; // Continuous conversion mode 00273 00274 // Set PGA/voltage range 00275 config |= m_gain; 00276 00277 // Set single-ended input channel 00278 switch (channel) { 00279 case (0): 00280 config |= ADS1015_REG_CONFIG_MUX_SINGLE_0; 00281 break; 00282 case (1): 00283 config |= ADS1015_REG_CONFIG_MUX_SINGLE_1; 00284 break; 00285 case (2): 00286 config |= ADS1015_REG_CONFIG_MUX_SINGLE_2; 00287 break; 00288 case (3): 00289 config |= ADS1015_REG_CONFIG_MUX_SINGLE_3; 00290 break; 00291 } 00292 00293 // Set the high threshold register 00294 // Shift 12-bit results left 4 bits for the ADS1015 00295 writeRegister(m_i2cAddress, ADS1015_REG_POINTER_HITHRESH, threshold << m_bitShift); 00296 00297 // Write config register to the ADC 00298 writeRegister(m_i2cAddress, ADS1015_REG_POINTER_CONFIG, config); 00299 } 00300 00301 /**************************************************************************/ 00302 /*! 00303 @brief In order to clear the comparator, we need to read the 00304 conversion results. This function reads the last conversion 00305 results without changing the config value. 00306 */ 00307 /**************************************************************************/ 00308 int16_t Adafruit_ADS1015::getLastConversionResults() 00309 { 00310 // Wait for the conversion to complete 00311 wait_ms(m_conversionDelay); 00312 00313 // Read the conversion results 00314 uint16_t res = readRegister(m_i2cAddress, ADS1015_REG_POINTER_CONVERT) >> m_bitShift; 00315 if (m_bitShift == 0) { 00316 return (int16_t)res; 00317 } else { 00318 // Shift 12-bit results right 4 bits for the ADS1015, 00319 // making sure we keep the sign bit intact 00320 if (res > 0x07FF) { 00321 // negative number - extend the sign to 16th bit 00322 res |= 0xF000; 00323 } 00324 return (int16_t)res; 00325 } 00326 }
Generated on Fri Jul 29 2022 07:13:23 by
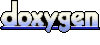