
STM32F411RE Embedded FLASH(internal FLASH) write.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "stm32f4xx_hal_flash.h" 00003 00004 #if 1 00005 #define DEBUG_PRINTF pc.printf 00006 #else 00007 #define DEBUG_PRINTF(...) 00008 #endif 00009 00010 namespace { 00011 Serial pc(SERIAL_TX, SERIAL_RX); 00012 00013 //STM32F411.C/E(DM00119316.pdf p.43 Table 4) 00014 const struct { 00015 uint8_t sector; 00016 uint32_t addr; 00017 } FLASH_SECTOR[] = { 00018 { 00019 FLASH_SECTOR_0, 00020 (uint32_t)0x08000000 /* Base address of Sector 0, 16 Kbytes */ 00021 }, 00022 { 00023 FLASH_SECTOR_1, 00024 (uint32_t)0x08004000 /* Base address of Sector 1, 16 Kbytes */ 00025 }, 00026 { 00027 FLASH_SECTOR_2, 00028 (uint32_t)0x08008000 /* Base address of Sector 2, 16 Kbytes */ 00029 }, 00030 { 00031 FLASH_SECTOR_3, 00032 (uint32_t)0x0800c000 /* Base address of Sector 3, 16 Kbytes */ 00033 }, 00034 { 00035 FLASH_SECTOR_4, 00036 (uint32_t)0x08010000 /* Base address of Sector 4, 64 Kbytes */ 00037 }, 00038 { 00039 FLASH_SECTOR_5, 00040 (uint32_t)0x08020000 /* Base address of Sector 5, 128 Kbytes */ 00041 }, 00042 { 00043 FLASH_SECTOR_6, 00044 (uint32_t)0x08040000 /* Base address of Sector 6, 128 Kbytes */ 00045 }, 00046 { 00047 FLASH_SECTOR_7, 00048 (uint32_t)0x08060000 /* Base address of Sector 7, 128 Kbytes */ 00049 } 00050 }; 00051 00052 /** @brief 内蔵FLASHへのbyte書込み(セクタ消去有り) 00053 * 00054 * @param[in] addr 書込み先アドレス(ADDR_FLASH_SECTOR_x) 00055 * @param[in] pData 書き込みデータ 00056 * @param[in] Len 書き込みデータサイズ 00057 */ 00058 void programByte(int sector, const uint8_t *pData, uint8_t Len) 00059 { 00060 HAL_StatusTypeDef ret; 00061 00062 /* flash control registerへのアクセス許可 */ 00063 HAL_FLASH_Unlock(); 00064 DEBUG_PRINTF("unlocked.\n"); 00065 00066 /* 消去(電圧 [2.7V to 3.6V]) */ 00067 FLASH_Erase_Sector(FLASH_SECTOR[sector].sector, FLASH_VOLTAGE_RANGE_3); 00068 DEBUG_PRINTF("erased.\n"); 00069 00070 /* 書込み(4byte単位) */ 00071 uint32_t addr = FLASH_SECTOR[sector].addr; 00072 for (int lp = 0 ; lp < Len; lp++) { 00073 DEBUG_PRINTF("addr:0x%08x data=%02x\n", addr, *pData); 00074 ret = HAL_FLASH_Program(FLASH_TYPEPROGRAM_BYTE, addr, *pData); 00075 if (ret != HAL_OK) { 00076 //trouble!! 00077 DEBUG_PRINTF("fail\n"); 00078 while (true) {} 00079 } 00080 addr++; 00081 pData++; 00082 } 00083 00084 /* flash control registerへのアクセス禁止 */ 00085 HAL_FLASH_Lock(); 00086 } 00087 00088 const uint8_t *getFlash(int sector) { return (const uint8_t *)FLASH_SECTOR[sector].addr; } 00089 } 00090 00091 00092 00093 int main() 00094 { 00095 uint8_t str[] = "Hello."; 00096 00097 DEBUG_PRINTF("%s\n", str); 00098 00099 programByte(7, str, sizeof(str)); 00100 00101 DEBUG_PRINTF("success[%s]\n", getFlash(7)); 00102 }
Generated on Thu Jul 14 2022 07:37:41 by
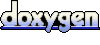