
MbedJSONValue library example
Dependencies: MbedJSONValue mbed
main.cpp
00001 #include "mbed.h" 00002 #include "MbedJSONValue.h" 00003 00004 /* 00005 * The MbedJSONValue is memory hungry class as I think. 00006 * If your platform has enough SRAM, you can go for it. 00007 * The library itself works fine but user need to caution using it. 00008 * It's fine with this: 00009 * MbedJSONValue &ele = demo["menu"]["popup"]["menuitem"][i]; 00010 * It's big problem with this(without &): 00011 * MbedJSONValue ele = demo["menu"]["popup"]["menuitem"][i]; 00012 */ 00013 void parse() { 00014 MbedJSONValue demo; 00015 00016 const char *json = "{\"string\": \"it works\", \"number\": 3.14, \"integer\":5}"; 00017 00018 parse(demo, json); 00019 printf("string =%s\r\n" , demo["string"].get<string>().c_str()); 00020 printf("number =%f\r\n" , demo["number"].get<double>()); 00021 printf("integer =%d\r\n" , demo["integer"].get<int>()); 00022 } 00023 00024 void serialize() { 00025 MbedJSONValue v; 00026 MbedJSONValue inner; 00027 string val = "tt"; 00028 00029 v["aa"] = val; 00030 v["bb"] = 1.66; 00031 inner["test"] = true; 00032 inner["integer"] = 1.0; 00033 v["inner"] = inner; 00034 00035 string str = v.serialize(); 00036 printf("serialized content = %s\r\n" , str.c_str()); 00037 } 00038 00039 void advanced() { 00040 MbedJSONValue demo; 00041 const char *jsonsoure = 00042 "{\"menu\": {" 00043 "\"id\": \"f\"," 00044 "\"popup\": {" 00045 " \"menuitem\": [" 00046 " {\"v\": \"0\"}," 00047 " {\"v\": \"1\"}," 00048 " {\"v\": \"2\"}" 00049 " ]" 00050 " }" 00051 "}" 00052 "}"; 00053 00054 std::string err; 00055 parse(demo, jsonsoure, jsonsoure + strlen(jsonsoure), &err); 00056 printf("res error? %s\r\n", err.c_str()); 00057 00058 printf("id =%s\r\n", demo["menu"]["id"].get<string>().c_str()); 00059 00060 for (int i = 0; i < demo["menu"]["popup"]["menuitem"].size(); ++i) { 00061 MbedJSONValue &ele = demo["menu"]["popup"]["menuitem"][i]; 00062 const string &s = ele["v"].get<string>(); 00063 00064 printf("menu item value =%s\r\n",s.c_str()); 00065 } 00066 00067 printf("serialized content = %s\r\n" , demo.serialize().c_str()); 00068 } 00069 00070 int main() { 00071 printf("Starting MbedJSON\r\n"); 00072 printf(">>> parsing \r\n"); 00073 parse(); 00074 printf(">>> serializing \r\n"); 00075 serialize(); 00076 printf(">>> advanced parsing \r\n"); 00077 advanced(); 00078 printf("Ending MbedJSON\r\n"); 00079 }
Generated on Fri Jul 15 2022 01:36:42 by
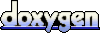