Brought over the files from the Freescale site and modified for mbed.
Embed:
(wiki syntax)
Show/hide line numbers
vfnLCD.h
00001 //#include "common.h" 00002 #include "mbed.h" 00003 #include "LCDConfig.h" // indicates how LCD module is configured and what type of LCD is going to be used 7seg, 14-seg, 16 seg, DotMatrix etc 00004 00005 #define LCD_ALTERNATE_MODE 4 // Write message to Alternate Backplanes 00006 #define LCD_NORMAL_MODE 0 // Write message to Original BackPlanes 00007 00008 00009 /* variables */ 00010 extern uint32_t __VECTOR_RAM[]; //Get vector table that was copied to RAM 00011 00012 extern const uint32_t MASK_BIT[]; 00013 extern const uint32_t *LCD_TO_PORT[]; 00014 00015 00016 #if _LCDINTENABLE == 1 00017 extern void LcdInterruptCallBack(void); 00018 #endif 00019 00020 class vfnLCD { 00021 00022 public: 00023 /*|||||||||||||||||||||| vfnLCD_Init |||||||||||||||||||||| 00024 brief: Initialize all the registers on the mcu module 00025 param: void return: void 00026 */ 00027 vfnLCD(void); 00028 00029 void vfnLCD_Home (void); 00030 /*|||||||||||||||||||||| vfnLCD_Write_Char |||||||||||||||||||||| 00031 brief: Writes on char after the last character was write 00032 param: uint8 --- Ascii to write return: void 00033 */ 00034 void vfnLCD_Write_Char (uint8_t lbValue); 00035 00036 /* 00037 Send a message until a end of char or max number of characters 00038 if the message size is greater than LCD character the message is cut ad the size of LCD Characters 00039 If the message lenght is minor than Display character is filled with Blanks 00040 */ 00041 void vfnLCD_Write_Msg (uint8_t *lbpMessage); 00042 00043 00044 00045 /*|||||||||||||||||||||| vfnLCD_Write_Msg |||||||||||||||||||||| 00046 brief: Writes on message on the LCD if the message is longer than the number of character is send to the Scroll function 00047 param: uint8 pointer --- the first character on the array to write 00048 param: uint8 --- the numbers of characterts to write 00049 return: void 00050 */ 00051 void vfnLCD_Write_MsgPlace (uint8_t *lbpMessage, uint8_t lbSize ); 00052 00053 /*|||||||||||||||||||||| vfnLCD_Scroll |||||||||||||||||||||| 00054 brief: Moves the message on the LCD 00055 param: uint8 pointer --- the first character on the array to write 00056 param: uint8 --- the size of the character 00057 return: void 00058 */ 00059 00060 void vfnLCD_Scroll (uint8_t *lbpMessage,uint8_t lbSize); 00061 00062 /*|||||||||||||||||||||| vfnLCD_All_Segments_ON |||||||||||||||||||||| 00063 brief: Turns on all the segments on the LCD 00064 param: uint8 pointer --- the first character on the array to write 00065 param: uint8 --- the size of the character 00066 return: void 00067 */ 00068 00069 void vfnLCD_All_Segments_ON (void); 00070 00071 /*Same as clear dispay */ 00072 void vfnLCD_All_Segments_OFF (void); 00073 00074 void vfnLCD_Contrast (uint8_t lbContrast); 00075 void vfnLCD_isrv(void) ; 00076 00077 00078 /* functions for DOT matrix LCD panel only*/ 00079 void vfnLCD_All_Segments_Char (uint8_t val); 00080 void PutPoint(uint8_t x, uint8_t y); 00081 void ClrPoint(uint8_t x, uint8_t y); 00082 void SetX(uint8_t x, uint8_t value); 00083 00084 private: 00085 char lcdBuffer[]; 00086 00087 00088 uint8_t lcd_alternate_mode; 00089 00090 00091 void LCD_vector_interrupt_init(void); 00092 void lcd_pinmux(uint8_t mux_val); //only 0 or 7 is allowed 00093 00094 void vfnBP_VScroll(int8_t scroll_count); 00095 00096 // LCD call samples 00097 // vfnLCD_Home(); 00098 // vfnLCD_Write_Char ('0'); 00099 // vfnLCD_All_Segments_ON(); 00100 // vfnLCD_All_Segments_OFF(); 00101 // vfnLCD_Write_Msg("@@@@@@@@@"); // TURN ON all characters 00102 // vfnLCD_All_Segments_OFF(); 00103 00104 00105 00106 /*{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{ Macros }}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}*/ 00107 /*{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{ ---------------------------- }}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}*/ 00108 00109 #define LCD_ENABLE() (LCD_GCR |= LCD_GCR_LCDEN_MASK) 00110 #define LCD_DISABLE() (LCD_GCR &= ~LCD_GCR_LCDEN_MASK) 00111 #define LCD_VTRIM(x) (LCD_GCR &= ~LCD_GCR_RVTRIM_MASK; LCD_GCR |= LCD_GCR_RVTRIM(x)); 00112 00113 00114 00115 00116 00117 00118 00119 /*{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{ Functions }}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}*/ 00120 /*{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{{ ---------------------------- }}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}}*/ 00121 00122 00123 00124 /*|||||||||||||||||||||| vfnLCD_EnablePins |||||||||||||||||||| 00125 brief: Enable all the pins of the mcu directly connected to the LCD on use 00126 param: void return: void 00127 */ 00128 void vfnEnablePins (void); 00129 00130 /*|||||||||||||||||||||| vfnLCD_SetBackplanes |||||||||||||| 00131 brief: Set as a backplane and give the number of COM that corresponds 00132 param: void return: void 00133 */ 00134 void vfnSetBackplanes (void); 00135 00136 /*|||||||||||||||||||||| vfnLCD_Home |||||||||||||||||||||| 00137 brief: Reset the counter to the first position 00138 param: void 00139 return: void 00140 */ 00141 00142 00143 00144 void vfnLCD_interrupt_init(void); 00145 }; 00146
Generated on Tue Jul 26 2022 03:58:29 by
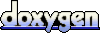