Library for the (decidedly not as good as the 24L01+) Nordic 2401A radio.
Fork of Nrf2401 by
Nrf2401A.h
00001 /* 00002 * Nrf2401A.h 00003 * A simplistic interface for using Sparkfun's Nrf2401A breakout boards with Arduino 00004 * 00005 * Ported to mbed by Jas Strong <jasmine@heroicrobotics.com> 00006 * 00007 * Original code for http://labs.ideo.com by Jesse Tane March 2009 00008 * 00009 * License: 00010 * -------- 00011 * This is free software. You can redistribute it and/or modify it under 00012 * the terms of Creative Commons Attribution 3.0 United States License. 00013 * To view a copy of this license, visit http://creativecommons.org/licenses/by/3.0/us/ 00014 * or send a letter to Creative Commons, 171 Second Street, Suite 300, San Francisco, California, 94105, USA. 00015 * 00016 * Original version Notes: 00017 * ----------------------- 00018 * For documentation on how to use this library, please visit http://www.arduino.cc/playground/Main/InterfacingWithHardware 00019 * Pin connections should be as follows for Arduino: 00020 * 00021 * DR1 = 2 (digital pin 2) 00022 * CE = 3 00023 * CS = 4 00024 * CLK = 5 00025 * DAT = 6 00026 * 00027 */ 00028 00029 #include "mbed.h" 00030 00031 #define NRF2401_BUFFER_SIZE 25 00032 00033 class Nrf2401 00034 { 00035 public: 00036 00037 // properties 00038 00039 volatile unsigned char data[NRF2401_BUFFER_SIZE]; 00040 volatile unsigned int remoteAddress; 00041 volatile unsigned int localAddress; 00042 volatile unsigned char dataRate; 00043 volatile unsigned char channel; 00044 volatile unsigned char power; 00045 volatile unsigned char mode; 00046 00047 // methods 00048 00049 Nrf2401(PinName n_DR1, PinName n_CE, PinName n_CS, PinName n_CLK, PinName n_DAT); 00050 void rxMode(unsigned char messageSize=0); 00051 void txMode(unsigned char messageSize=0); 00052 void write(unsigned char dataByte); 00053 void write(unsigned char* dataBuffer=0); 00054 void read(unsigned char* dataBuffer=0); 00055 bool available(void); 00056 00057 // you shouldn't need to use anything below this point.. 00058 private: 00059 volatile unsigned char payloadSize; 00060 volatile unsigned char configuration[15]; 00061 void configure(void); 00062 void loadConfiguration(bool modeSwitchOnly=false); 00063 void loadByte(unsigned char byte); 00064 inline void select_chip(void) { 00065 _cs = 1; 00066 } 00067 00068 inline void deselect_chip(void) { 00069 _cs = 0; 00070 } 00071 00072 inline void enable_chip(void) { 00073 _ce = 1; 00074 } 00075 00076 inline void disable_chip(void) { 00077 _ce = 0; 00078 } 00079 00080 inline void cycle_clock(void) { 00081 _clk = 1; 00082 wait_us(1); 00083 _clk = 0; 00084 } 00085 00086 inline void tx_data_lo(void) { 00087 _dat.output(); 00088 _dat = 1; 00089 } 00090 00091 inline void tx_data_hi(void) { 00092 _dat.output(); 00093 _dat = 1; 00094 } 00095 00096 inline int rx_data_hi(void) { 00097 _dat.input(); 00098 return _dat; 00099 } 00100 00101 inline int data_ready(void) { 00102 return _dr1; 00103 } 00104 00105 DigitalOut _ce, _cs, _clk; 00106 DigitalIn _dr1; 00107 DigitalInOut _dat; 00108 };
Generated on Wed Jul 20 2022 01:09:57 by
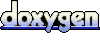