Library for the (decidedly not as good as the 24L01+) Nordic 2401A radio.
Fork of Nrf2401 by
Nrf2401A.cpp
00001 /* 00002 * Nrf2401A.h 00003 * A simplistic interface for using Sparkfun's Nrf2401A breakout boards with Arduino 00004 * 00005 * Ported to mbed by Jas Strong <jasmine@heroicrobotics.com> 00006 * 00007 * Original code for http://labs.ideo.com by Jesse Tane March 2009 00008 * 00009 * License: 00010 * -------- 00011 * This is free software. You can redistribute it and/or modify it under 00012 * the terms of Creative Commons Attribution 3.0 United States License. 00013 * To view a copy of this license, visit http://creativecommons.org/licenses/by/3.0/us/ 00014 * or send a letter to Creative Commons, 171 Second Street, Suite 300, San Francisco, California, 94105, USA. 00015 * 00016 * Notes: 00017 * ------ 00018 * For documentation on how to use this library, please visit http://www.arduino.cc/playground/Main/InterfacingWithHardware 00019 * 00020 */ 00021 00022 #include "Nrf2401A.h" 00023 #include "mbed.h" 00024 00025 00026 Nrf2401::Nrf2401(PinName n_DR1, PinName n_CE, PinName n_CS, PinName n_CLK, PinName n_DAT) : 00027 _dr1(n_DR1), _ce(n_CE), _cs(n_CS), _clk(n_CLK), _dat(n_DAT) 00028 { 00029 _dat.output(); 00030 00031 remoteAddress = 0; 00032 localAddress = 0; 00033 payloadSize = 0; 00034 dataRate = 1; 00035 channel = 111; 00036 power = 3; 00037 mode = 0; 00038 00039 configuration[7] = 234; 00040 configuration[8] = 223; 00041 configuration[9] = 212; 00042 00043 disable_chip(); 00044 deselect_chip(); 00045 } 00046 00047 void Nrf2401::rxMode(unsigned char messageSize) 00048 { 00049 mode = 1; 00050 if(messageSize) payloadSize = messageSize, configure(); 00051 else configuration[14] |= 1, loadConfiguration(true); 00052 enable_chip(); 00053 wait_us(250); 00054 } 00055 00056 void Nrf2401::txMode(unsigned char messageSize) 00057 { 00058 mode = 0; 00059 if(messageSize) payloadSize = messageSize, configure(); 00060 else configuration[14] &= ~1, loadConfiguration(true); 00061 wait_us(250); 00062 } 00063 00064 void Nrf2401::write(unsigned char* dataBuffer) 00065 { 00066 if(!dataBuffer) dataBuffer = (unsigned char*) data; 00067 enable_chip(); 00068 wait_us(5); 00069 loadByte(configuration[7]); 00070 loadByte(configuration[8]); 00071 loadByte(configuration[9]); 00072 loadByte(remoteAddress >> 8); 00073 loadByte(remoteAddress); 00074 for(int i=0; i<payloadSize; i++) loadByte(dataBuffer[i]); 00075 disable_chip(); 00076 wait_us(250); 00077 } 00078 00079 void Nrf2401::write(unsigned char dataByte) 00080 { 00081 data[0] = dataByte; 00082 write(); 00083 } 00084 00085 void Nrf2401::read(unsigned char* dataBuffer) 00086 { 00087 if(!dataBuffer) dataBuffer = (unsigned char*) data; 00088 disable_chip(); 00089 wait_ms(2); 00090 for(int i=0; i<payloadSize; i++) 00091 { 00092 dataBuffer[i] = 0; 00093 for(int n=7; n>-1; n--) 00094 { 00095 if(rx_data_hi()) dataBuffer[i] |= (1 << n); 00096 wait_us(1); 00097 cycle_clock(); 00098 } 00099 } 00100 enable_chip(); 00101 wait_us(1); 00102 } 00103 00104 bool Nrf2401::available(void) 00105 { 00106 return data_ready(); 00107 } 00108 00109 //// you shouldn't need to call directly any of the methods below this point... 00110 00111 void Nrf2401::configure(void) 00112 { 00113 configuration[1] = payloadSize << 3; 00114 configuration[10] = localAddress >> 8; 00115 configuration[11] = localAddress; 00116 configuration[12] = 163; 00117 configuration[13] = power | (dataRate << 5) | 76; 00118 configuration[14] = mode | (channel << 1); 00119 loadConfiguration(); 00120 } 00121 00122 void Nrf2401::loadConfiguration(bool modeSwitchOnly) 00123 { 00124 disable_chip(); 00125 select_chip(); 00126 wait_us(5); 00127 if(modeSwitchOnly) loadByte(configuration[14]); 00128 else for(int i=0; i<15; i++) loadByte(configuration[i]); 00129 deselect_chip(); 00130 } 00131 00132 void Nrf2401::loadByte(unsigned char byte) 00133 { 00134 for(int i=7; i>-1; i--) 00135 { 00136 if((byte & (1 << i)) == 0) tx_data_lo(); 00137 else tx_data_hi(); 00138 wait_us(1); 00139 cycle_clock(); 00140 } 00141 }
Generated on Wed Jul 20 2022 01:09:57 by
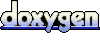